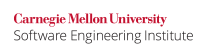
Copying data into an array that is not large enough to hold that data results in a buffer overflow. To prevent such errors, data copied to the destination array must be restricted based on the size of the destination array, or, preferably, the destination array must be guaranteed to be large enough to hold the data to be copied.
Vulnerabilities that result from copying data to an undersized buffer often involve null-terminated character arrays (NTCA). Consult STR31-CPP. Guarantee that storage for character arrays has sufficient space for character data and the null terminator for specific examples of this rule that involve NTCA.
Noncompliant Code Example (Array)
Improper use of functions that limit copies with a size specifier, such as memcpy()
, may result in a buffer overflow. In this noncompliant code example, an array of integers is copied from src
to dest
using memcpy()
. However, the programmer mistakenly specified the amount to copy based on the size of src
, which is stored in len
, rather than the space available in dest
. If len
is greater than 256, then a buffer overflow will occur.
enum { WORKSPACE_SIZE = 256 }; void func(const int src[], size_t len) { int dest[WORKSPACE_SIZE]; memcpy(dest, src, len * sizeof(int)); /* ... */ }
Compliant Solution (Array)
The amount of data copied should be limited based on the available space in the destination buffer. This can be accomplished by adding a check to ensure the amount of data to be copied from src
can fit in dest
.
enum { WORKSPACE_SIZE = 256 }; void func(const int src[], size_t len) { int dest[WORKSPACE_SIZE]; if (len > WORKSPACE_SIZE) { /* Handle Error */ } memcpy(dest, src, sizeof(int)*len); /* ... */ }
Noncompliant Code Example (Vector)
Vectors can be subject to the same vulnerabilities. The copy
function provides no inherent bounds checking, and can lead to a buffer overflow. In this noncompliant code example, a vector of integers is copied from src
to dest
using copy()
. Since copy()
does nothing to expand the dest
vector, thus the program will overflow the buffer on copying the first element.
void func(const vector<int> src) { vector<int> dest; copy( src.begin(), src.end(), dest.begin()); /* ... */ }
Compliant Solution (Vector)
The proper way to use copy()
is to ensure the destination container can hold all the elements being copied to it. This code example enlarges the capacity of the vector before starting the copyo.
void func(const vector<int> src) { vector<int> dest; dest.resize( src.size()); copy( src.begin(), src.end(), dest.begin()); /* ... */ }
Risk Assessment
Copying data to a buffer that is too small to hold that data results in a buffer overflow. Attackers can exploit this condition to execute arbitrary code.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ARR33-CPP |
high |
likely |
medium |
P18 |
L1 |
Automated Detection
Fortify SCA Version 5.0 can detect violations of this rule.
Splint Version 3.1.1 can detect violations of this rule.
Compass/ROSE can detect some violations of this rule.
Klocwork Version 8.0.4.16 can detect violations of this rule with the ABR checker.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as ARR33-C. Guarantee that copies are made into storage of sufficient size.
References
[[ISO/IEC PDTR 24772]] "XYB Buffer Overflow in Heap," "XYW Buffer Overflow in Stack," and "XYZ Unchecked Array Indexing"
[[MITRE 07]] CWE ID 119, "Failure to Constrain Operations within the Bounds of an Allocated Memory Buffer"
[[Seacord 05a]] Chapter 2, "Strings"
[[VU#196240]]
ARR32-CPP. Ensure size arguments for variable length arrays are in a valid range 06. Arrays (ARR) VOID ARR34-CPP. Ensure that array types in expressions are compatible