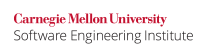
This coding standard consists of rules and recommendations, collectively referred to as guidelines. Rules are meant to provide normative requirements for code, whereas recommendations are meant to provide guidance that, when followed, should improve the safety, reliability, and security of software systems. However, a violation of a recommendation does not necessarily indicate the presence of a defect in the code.
Rules
Rules must meet the following criteria:
- Violation of the guideline is likely to result in a defect that may adversely affect the safety, reliability, or security of a system, for example, by introducing a security flaw that may result in an exploitable vulnerability.
- The guideline does not rely on source code annotations or assumptions.
- Conformance to the guideline can be determined through automated analysis (either static or dynamic), formal methods, or manual inspection techniques.
Rules are identified by the label rule.
Recommendations
Recommendations are suggestions for improving code quality. Guidelines are defined to be recommendations when all of the following conditions are met:
- Application of a guideline is likely to improve the safety, reliability, or security of software systems.
- One or more of the requirements necessary for a guideline to be considered a rule cannot be met.
The set of recommendations that a particular development effort adopts depends on the requirements of the final software product. Projects with stricter requirements may decide to dedicate more resources to ensuring the safety, reliability, and security of a system and consequently are likely to adopt a broader set of recommendations.
Recommendations are identified by the label recommendation.
Noncompliant Code Examples and Compliant Solutions
Noncompliant code examples illustrate code that violates the guideline under discussion. It is important to note that these are only examples, and eliminating all occurrences of the example does not necessarily mean that the code being analyzed is now compliant with the guideline.
Noncompliant code examples are typically followed by compliant solutions, which show how the noncompliant code example can be recoded in a secure, compliant manner. Except where noted, noncompliant code examples should contain violations only of the guideline under discussion. Compliant solutions should comply with all of the secure coding rules but may on occasion fail to comply with a recommendation.
Coding Conventions
Unless otherwise specified, all code should compile on a reasonably modern version of Java.
Code that is only expected to run on more modern versions of Java should indicate the oldest version of Java that supports them: e.g. Compliant Solution (Java 7).
In order to compile the code, you will need to include appropriate packages. For example, if the code invokes List.of()
, you may need to import the java.util.List
class.
Many code examples will contain ellipsis in comments. This indicates that the comment may be replaced by arbitrary code that satisfies the comment. A comment with only ellipsis suggests that the code may do anything.
Proper error handling is a controversial subject, and many applications and libraries provide their own idiosyncratic error handling mechanisms. See Rule 07. Exceptional Behavior (ERR) and Rec. 07. Exceptional Behavior (ERR) for our guidelines on handling errors. When our code detects that an error condition might have occurred, and handling that error condition is not endemic to the guideline itself, we will use the comment: /* Handle Error */
. This comment implies that the error is somehow addressed, so that the code does not fall through. The code may abort, or fix the error somehow. For example:
File file = new File("file"); if (!file.delete()) { /* Handle Error */ } /* ... file must no longer exist here. Continue ... */
Exceptions
Any rule or recommendation may specify a small set of exceptions detailing the circumstances under which the guideline is not necessary to ensure the safety, reliability, or security of software. Exceptions are informative only and are not required to be followed.
Coding practices that specify one or more exceptions are identified by the label exceptions.
Identifiers
Each rule and recommendation is given a unique identifier. These identifiers consist of three parts:
- A three-letter mnemonic representing the section of the standard
- A two-digit numeric value in the range of 00 to 99
- The letter J indicating that this is a Java language guideline
The three-letter mnemonic can be used to group similar coding practices and to indicate to which category a coding practice belongs.
The numeric value is used to give each coding practice a unique identifier. Numeric values in the range of 00 to 49 are reserved for rules, and values in the range of 50 to 99 are reserved for recommendations.
2 Comments
Anthony Vanelverdinghe
The links for "security flaw" and "vulnerability" should be updated to point to Rec. BB. Definitions
The links for "rule"/"recommendation"/"exceptions" don't work.
The following phrase should be changed
> For example, if the code invokes
Math.sin()
, you may need to import thejava.lang.Math
package.because (a) Math is a class, not a package and (b) the java.lang package is imported automatically, so an import is never required for Math.sin(). Better would be:
> For example, if the code invokes
List.of()
, you may need to import thejava.util
.List class.The phrase "The letter J indicating that this is a Java language guideline" should be a separate bullet point.
PS: is there a way to edit pages directly, and then have them validated by an authorized user (similar to a Pull Request on GitHub)? That would be more efficient, both for me and the person addressing this.
David Svoboda
Thank you for this comment. I have fixed each of these problems.