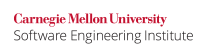
Applications should not use insecure or weak cryptographic primitives because it is possible to circumvent such protective measures given the computational capacity of modern computers. For example, the DES encryption algorithm is considered highly insecure in today's age of super computers and botnets. Messages encrypted using DES have known to be successfully decrypted by brute force within a single day by machines such as the Electronic Frontier Foundation's (EFF) Deep Crack.
Noncompliant Code Example
This noncompliant code example encrypts a String
input by using a weak cryptographic algorithm (DES).
SecretKey key = KeyGenerator.getInstance("DES").generateKey(); Cipher cipher = Cipher.getInstance("DES"); cipher.init(Cipher.ENCRYPT_MODE, key); // Encode bytes as UTF8; strToBeEncrypted contains the input string // that is to be encrypted byte[] encoded = strToBeEncrypted.getBytes("UTF8"); // Perform encryption byte[] encrypted = cipher.doFinal(encoded);
Compliant Solution
This compliant solution uses the more secure AES algorithm to perform the encryption. Decryption follows similar logic and has been omitted from this discussion.
Cipher cipher = Cipher.getInstance("AES"); KeyGenerator kgen = KeyGenerator.getInstance("AES"); kgen.init(128); // 192 and 256 bits may be unavailable SecretKey skey = kgen.generateKey(); byte[] raw = skey.getEncoded(); SecretKeySpec skeySpec = new SecretKeySpec(raw, "AES"); cipher.init(Cipher.ENCRYPT_MODE, skeySpec); // Encode bytes as UTF8; strToBeEncrypted contains the input string // that is to be encrypted byte[] encoded = strToBeEncrypted.getBytes("UTF8"); // Perform encryption byte[] encrypted = cipher.doFinal(encoded);
Risk Assessment
Failure to use a mathematically and computationally secure cryptographic algorithm can result in the disclosure of sensitive information.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC01- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
[[API 2006]]
[[MITRE 2009]] CWE ID 327 "Use of a Broken or Risky Cryptographic Algorithm"
MSC00-J. Do not mix generic with non-generic raw types in new code 49. Miscellaneous (MSC)