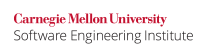
Division and modulo operations are susceptible to divide-by-zero errors. Consequently, the divisor in a division or modulo operation must be checked for zero prior to the operation.
Division
The result of the /
operator is the quotient from the division of the first arithmetic operand by the second arithmetic operand. Division operations are susceptible to divide-by-zero errors. Overflow can also occur during two's complement signed integer division when the dividend is equal to the minimum (negative) value for the signed integer type and the divisor is equal to —1. (See rule "NUM00-J. Detect or prevent integer overflow".)
Noncompliant Code Example
This code can result in a divide-by-zero error during the division of the signed operands sl1
and sl2
.
signed long sl1, sl2, result; /* Initialize sl1 and sl2 */ result = sl1 / sl2;
Compliant Solution
This compliant solution tests the suspect division operation to guarantee there is no possibility of divide-by-zero errors.
signed long sl1, sl2, result; /* Initialize sl1 and sl2 */ if ( (sl2 == 0) ) { /* handle error condition */ } else { result = sl1 / sl2; }
Modulo
The %
operator provides the remainder when two operands of integer type are divided.
Noncompliant Code Example
This code can result in a divide-by-zero error during the remainder operation on the signed operands sl1
and sl2
.
signed long sl1, sl2, result; /* Initialize sl1 and sl2 */ result = sl1 % sl2;
Compliant Solution
This compliant solution tests the suspect remainder operation to guarantee there is no possibility of a divide-by-zero error.
signed long sl1, sl2, result; /* Initialize sl1 and sl2 */ if ( (sl2 == 0 ) ) { /* handle error condition */ } else { result = sl1 % sl2; }
Risk Assessment
A divide-by-zero can result in abnormal program termination and denial of service.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
NUM15-J |
low |
likely |
medium |
P6 |
L2 |
Automated Detection
Automated detection exists for C and C++, but not for Java yet.
Related Guidelines
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="0b524024-1d0c-4092-845b-a06a9994f0bb"><ac:plain-text-body><![CDATA[ |
[[ISO/IEC 9899:1999 |
AA. Bibliography#ISO/IEC 9899-1999]] |
Section 6.5.5, "Multiplicative operators" |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="f877018a-91e2-4311-98e4-b013073b3cbe"><ac:plain-text-body><![CDATA[ |
[[Seacord 05 |
AA. Bibliography#Seacord 05]] |
Chapter 5, "Integers" |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="5efde24f-e8bd-4ad4-ba0d-a5265e19b776"><ac:plain-text-body><![CDATA[ |
[[Warren 02 |
AA. Bibliography#Warren 02]] |
Chapter 2, "Basics" |
]]></ac:plain-text-body></ac:structured-macro> |
NUM13-J. Beware of precision loss when converting primitive integers to floating-point 03. Numeric Types and Operations (NUM)