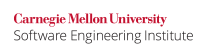
A boxing conversion converts the value of a primitive type to the corresponding value of the reference type, for example, from int
to Integer
[[JLS 2005]]. This is convenient in cases where an object parameter is required, such as with collection classes like Map
and List
. Another use case is for interoperation with methods that require their parameters to be object references rather than primitive types. Automatic conversion to the resulting wrapper types also reduces clutter in code.
Expressions autobox into the intended type when the reference type causing the boxing conversion is one of the specific numeric wrapper types (e.g., Byte
, Short
, Integer
, Long
, Float
, or Double
). However, autoboxing can produce unexpected results when the reference type causing the boxing conversion is nonspecific (e.g., Number
or Object
) and the value being converted is the result of an expression that mixes primitive numeric types. In this latter case, the specific wrapper type that results from the boxing conversion is chosen based on the numeric promotion rules governing the expression evaluation. See rule "NUM18-J. Be aware of numeric promotion behavior" for additional explanation of the details of the promotion rules. Consequently, programs that use primitive arithmetic expressions as actual arguments passed to method parameters that have non-specific reference types must cast the expression to the intended primitive numeric type before the boxing conversion takes place.
Noncompliant Code Example
This noncompliant code example prints 100
as the size of the HashSet
rather than the expected result (1
). The combination of values of types short
and int
in the operation i-1
causes the result to be autoboxed into an object of type Integer
, rather than one of type Short
. See rule "[NUM18-J. Be aware of numeric promotion behavior]" for additional explanation of the details of the promotion rules. The HashSet
contains only values of type Short
; the code attempts to remove objects of type Integer
. Consequently, the remove()
operation accomplishes nothing. The language's type checking guarantees that only values of type Short
can be inserted into the HashSet
. Nevertheless, programmers are free to attempt to remove an object of any type because Collections<E>.remove()
accepts an argument of type Object
rather than of type E
. Such behavior can result in unintended object retention or memory leaks [[Techtalk 2007]].
public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for (short i = 0; i < 100; i++) { s.add(i); s.remove(i - 1); } System.out.println(s.size()); } }
Compliant Solution
Objects removed from a collection must share the type of the elements of the collection. Numeric promotion and autoboxing can produce unexpected object types. This compliant solution uses an explicit cast to short
that parallels the intended boxed type.
public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for (short i = 0; i < 100; i++) { s.add(i); s.remove((short)(i - 1)); // cast to short } System.out.println(s.size()); } }
Risk Assessment
Allowing autoboxing to produce objects of an unintended type can cause silent failures with some APIs, such as the Collections
library. These failures can result in unintended object retention, memory leaks, or incorrect program operation.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP04-J |
low |
probable |
low |
P6 |
L2 |
Automated Detection
Detection of invocations of Collection.remove()
whose operand fails to match the type of the elements of the underlying collection is straightforward. It is possible, albeit unlikely, that some of these invocations could be intended. The remainder are heuristically likely to be in error. Automated detection for other APIs could be possible.
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="60f0ea49-7c44-40aa-b954-34bd28e28987"><ac:plain-text-body><![CDATA[ |
[[Core Java 2004 |
AA. Bibliography#Core Java 04]] |
Chapter 5 |
]]></ac:plain-text-body></ac:structured-macro> |
|
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="e9910f64-040e-4bf1-8be3-f2714e40efa4"><ac:plain-text-body><![CDATA[ |
[[JLS 2005 |
AA. Bibliography#JLS 05]] |
[§5.1.7, "Boxing Conversions" |
http://java.sun.com/docs/books/jls/third_edition/html/conversions.html#5.1.7] |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="b0ce40a1-b413-447d-9070-33d7fc2172e7"><ac:plain-text-body><![CDATA[ |
[[Techtalk 2007 |
AA. Bibliography#Techtalk 07]] |
"The Joy of Sets" |
]]></ac:plain-text-body></ac:structured-macro> |
EXP06-J. Do not use side-effecting expressions in assertions 02. Expressions (EXP) EXP01-J. Never dereference null pointers