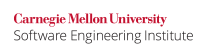
Parameterized classes have expectations of the objects that they reference; they expect certain objects to match their paramaterized types. Heap pollution occurs when a parameterized class references an object that it expects to be of the parameterized type, but the object is of a different type. For more information on heap pollution, see the Java Language Specification, §4.12.2.1, "Heap Pollution," [JLS 2005]). For instance, consider the following code snippet.
List l = new ArrayList(); List<String> ls = l; // Produces unchecked warning
It is insufficient to rely on unchecked warnings alone to detect violations of this rule. According to the Java Language Specification, §4.12.2.1, "Heap Pollution," [JLS 2005]:
Note that this does not imply that heap pollution only occurs if an unchecked warning actually occurred. It is possible to run a program where some of the binaries were compiled by a compiler for an older version of the Java programming language, or by a compiler that allows the unchecked warnings to suppressed [sic]. This practice is unhealthy at best.
Extending legacy classes and making the overriding methods generic fails because this is disallowed by the Java Language Specification..
Mixing generically typed code with raw typed code is one common source of heap pollution. Prior to Java 5, all code used raw types. Allowing mixing enabled developers to preserve compatibility between non-generic legacy code and newer generic code. Using raw types with generic code causes most Java compilers to issue "unchecked" warnings but still compile the code. When generic and nongeneric types are used together correctly, these warnings can be ignored; at other times, these warnings can denote potentially unsafe operations.
According to the Java Language Specification, §4.8, "Raw Types," [JLS 2005]:
The use of raw types is allowed only as a concession to compatibility of legacy code. The use of raw types in code written after the introduction of genericity into the Java programming language is strongly discouraged. It is possible that future versions of the Java programming language will disallow the use of raw types.
Note that mixing generic and raw types is perfectly acceptable if heap pollution can be guaranteed not to occur.
Noncompliant Code Example
This noncompliant code example compiles but produces an unchecked warning because the raw type of the List.add()
method is used (the list
parameter in the addToList()
method) rather than the parameterized type.
class ListUtility { private static void addToList(List list, Object obj) { list.add(obj); // unchecked warning } public static void main(String[] args) { List<String> list = new ArrayList<String> (); addToList(list, 1); System.out.println(list.get(0)); // throws ClassCastException } }
When executed, this code throws an exception. This happens not because a List<String>
receives an Integer
but because the value returned by list.get(0)
is an improper type (an Integer
rather than a String
). In other words, the code throws an exception some time after the execution of the operation that actually caused the error, complicating debugging.
Compliant Solution (Parameterized Collection)
This compliant solution enforces type safety by changing the addToList()
method signature to enforce proper type checking.
class ListUtility { private static void addToList(List<String> list, String str) { list.add(str); // No warning generated } public static void main(String[] args) { List<String> list = new ArrayList<String> (); addToList(list, "1"); System.out.println(list.get(0)); } }
The compiler prevents insertion of an Object
to the parameterized list
because addToList()
cannot be called with an argument whose type produces a mismatch. This code has consequently been changed to add a String
to the list instead of an Integer
.
Compliant Solution (Legacy Code)
While the previous compliant solution eliminates use of raw collections, it may be infeasible to implement this solution when interoperating with legacy code.
Suppose that the addToList()
method is legacy code that cannot be changed. The following compliant solution creates a checked view of the list by using the Collections.checkedList()
method. This method returns a wrapper collection that performs runtime type checking in its implementation of the add()
method before delegating to the backend List<String>
. The wrapper collection can be safely passed to the legacy addToList()
method.
class ListUtility { private static void addToList(List list, Object obj) { list.add(obj); // Unchecked warning, also throws ClassCastException } public static void main(String[] args) { List<String> list = new ArrayList<String> (); List<String> checkedList = Collections.checkedList(list, String.class); addToList(checkedList, 1); System.out.println(list.get(0)); } }
The compiler still issues the unchecked warning, which may still be ignored. However, the code now fails when it attempts to add the Integer
to the list, consequently preventing the program from proceeding with invalid data.
Noncompliant Code Example
This noncompliant code example compiles and runs cleanly because it suppresses the unchecked warning produced by the raw List.add()
method. The printOne()
method intends to print the value 1, either as an int
or as a double
depending on the type of the variable type
.
class ListAdder { @SuppressWarnings("unchecked") private static void addToList(List list, Object obj) { list.add(obj); // unchecked warning suppressed } private static <T> void printOne(T type) { if (!(type instanceof Integer || type instanceof Double)) { System.out.println("Cannot print in the supplied type"); } List<T> list = new ArrayList<T>(); addToList(list, 1); System.out.println(list.get(0)); } public static void main(String[] args) { double d = 1; int i = 1; System.out.println(d); ListAdder.printOne(d); System.out.println(i); ListAdder.printOne(i); } }
However, despite list
being correctly parameterized, this method prints 1 and never 1.0 because the int
value 1 is always added to list
without being type checked. This code produces the following output:
1.0 1 1 1
Compliant Solution (Parameterized Collection)
This compliant solution generifies the addToList()
method, eliminating any possible type violations.
class ListAdder { private static <T> void addToList(List<T> list, T t) { list.add(t); // No warning generated } private static <T> void printOne(T type) { if (type instanceof Integer) { List<Integer> list = new ArrayList<Integer>(); addToList(list, 1); System.out.println(list.get(0)); } else if (type instanceof Double) { List<Double> list = new ArrayList<Double>(); addToList(list, 1.0); // will not compile with 1 instead of 1.0 System.out.println(list.get(0)); } else { System.out.println("Cannot print in the supplied type"); } } public static void main(String[] args) { double d = 1; int i = 1; System.out.println(d); ListAdder.printOne(d); System.out.println(i); ListAdder.printOne(i); } }
This code compiles cleanly and produces the correct output:
1.0 1.0 1 1
If the method addToList()
is externally defined (such as in a library or as an upcall method) and cannot be changed, the same compliant method printOne()
can be used, but no warnings result if addToList(list, 1)
is used instead of addToList(list, 1.0)
. Great care must be taken to ensure type safety when generics are mixed with nongeneric code.
Noncompliant Code Example (List of lists)
Heap pollution occurs because Java does not enforce type correctness on lists of lists. This program happily tries to build a list of list of strings, while trying to sneak a single integer into the list. While the Java compiler emits several warnings, this program compiles and runs until it tries to extract the integer 42 from a List<String>
, and throws a ClassCastException
.
public class ListInspectorExample { public static void inspect(List<List<String>> l) { for (List<String> list : l) { for (String s : list) { // ClassCastException on 42 System.out.println(s); } } } public static void main(String[] args) { List<String> s = Arrays.asList("foo"); List i = Arrays.asList(42); // warning about raw types List<List<String>> l = new ArrayList<List<String>>(); l.add(s); l.add(i); // warning about unchecked convertion inspect(l); } }
Noncompliant Code Example (Array of lists)
The problem persists if the function parameter is replaced with an array of lists
public class ListInspectorExample { public static void inspect(List<String>[] l) { for (List<String> list : l) { for (String s : list) { // ClassCastException on 42 System.out.println(s); } } } public static void main(String[] args) { List<String> s = Arrays.asList("foo"); List i = Arrays.asList(42); // warning about raw types inspect(new List[] {s, i}); // warning about unchecked convertion } }
Noncompliant Code Example (Variadic Arguments)
The problem persists if the function parameter is changed to a variadic argument of List<String>
. During type erasure, the compiler translates this to a single array of objects.
public class ListInspectorExample { public static void inspect(List<String>... l) { for (List<String> list : l) { for (String s : list) { // ClassCastException on 42 System.out.println(s); } } } public static void main(String[] args) { List<String> s = Arrays.asList("foo"); List i = Arrays.asList(42); // warning about raw types inspect( s, i); // warning about variadic generic parameter } }
Compliant Solution (Generic Argument)
All such noncompliant examples can be mitigated by avoiding raw types. Simply replacing the List
of integers with a List<Integer>
causes the compiler to refuse to compile each noncompliant code example.
public class ListInspectorExample { public static void inspect(List<String>... l) { for (List<String> list : l) { for (String s : list) { // ClassCastException on 42 System.out.println(s); } } } public static void main(String[] args) { List<String> s = Arrays.asList("foo"); List<Integer> i = Arrays.asList(42); inspect( s, i); // improper argument types compiler error } }
Risk Assessment
Mixing generic and nongeneric code can produce unexpected results and exceptional conditions.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ03-J | low | probable | medium | P4 | L3 |
Bibliography
Item 23. Don't use raw types in new code | |
Puzzle 88. Raw Deal | |
8.3, Avoid Casting by Using Generics | |
| |
[JLS 2005] | |
| |
| |
Topic 3, Coping with Legacy | |
Chapter 8, Effective Generics | |
Principle of Indecent Exposure | |
Create a checked collection | |
Heap Pollution |