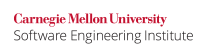
Hardcoding sensitive information, such as passwords and encryption keys, is an extremely dangerous practice. This is because adversaries who have access to the class files can decompile them to discover the sensitive information. Additionally, once the system goes into production mode, it can become unwieldy to manage and accommodate changes to the code. For instance, a change in password may need to be communicated using a patch [[Chess 07]].
Noncompliant Code Example
This noncompliant code example uses a password field instantiated as a String
.
class Hardcoded { String password = new String("guest"); public static void main(String[] args) { //.. } }
A malicious user can use the javap -c Hardcoded
command to disassemble the class and discover the hardcoded password. The output of the disassembler as shown below, reveals the password guest
in cleartext.
Compiled from "Hardcoded.java" class Hardcoded extends java.lang.Object{ java.lang.String password; Hardcoded(); Code: 0: aload_0 1: invokespecial #1; //Method java/lang/Object."<init>":()V 4: aload_0 5: new #2; //class java/lang/String 8: dup 9: ldc #3; //String guest 11: invokespecial #4; //Method java/lang/String."<init>":(Ljava/lang/String;)V 14: putfield #5; //Field password:Ljava/lang/String; 17: return public static void main(java.lang.String[]); Code: 0: return }
Compliant Solution
This compliant solution uses a char
array to store the password after it is retrieved from an external file existing in a secured directory. The password is immediately cleared out after use. This limits the exposure time.
class Password { public static void main(String[] args) throws IOException { char[] password = new char[100]; BufferedReader br = new BufferedReader(new InputStreamReader( new FileInputStream("password.txt"))); // Reads the password into the char array, returns the number of bytes read int n = br.read(password); // Decrypt password, perform operations for(int i = n - 1; i >= 0; i--) { // Manually clear out the password immediately after use password[i] = 0; } br.close(); } }
To further limit the exposure time of the sensitive password, follow the guideline MSC10-J. Limit the lifetime of sensitive data by replacing BufferedReader
with a direct NIO buffer.
Noncompliant Code Example
This noncompliant code example hardcodes the user name and password fields in the SQL connection request.
public final Connection getConnection() throws SQLException { return DriverManager.getConnection("jdbc:mysql://localhost/dbName", "username", "password"); }
Note that the one and two argument java.sql.DriverManager.getConnection()
methods may also be used incorrectly. Applets that contain similar code are also noncompliant because they may be executed in untrusted environments.
Compliant Solution
This compliant solution reads the user name and password from a configuration file present in a secure directory.
// Username and password are read at runtime from a secure config file public final Connection getConnection() throws SQLException { return DriverManager.getConnection("jdbc:mysql://localhost/dbName", username, password); }
It is also permissible to prompt the user for the user name and password at runtime and use the entered values.
Risk Assessment
Hardcoding sensitive information allows a malicious user to glean the information.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC03- J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Other Languages
This rule appears in the C Secure Coding Standard as MSC18-C. Be careful while handling sensitive data, such as passwords, in program code
References
[[Gong 03]] 9.4 Private Object State and Object Immutability
[[Chess 07]] 11.2 Outbound Passwords: Keep Passwords out of Source Code
[[Fortify 08]] "Unsafe Mobile Code: Database Access"
[[MITRE 09]] CWE ID 259 "Hard-Coded Password"
MSC02-J. Generate truly random numbers 49. Miscellaneous (MSC) MSC04-J. Do not use Object.equals() to compare cryptographic keys