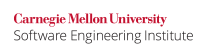
Validating method parameters ensures that any operations that use the method's arguments yield consistent results. Failure to do so can result in incorrect calculations, runtime exceptions, violation of class invariants and inconsistent object state.
It if often assumed that private
methods do not require any validation because they are not directly accessible from code present outside the class. This assumption is misleading as programming errors often arise due to legit code misbehaving in unanticipated ways. For example, a tainted value may propagate from a public
API to one of the internal methods via its parameters.
Assertions should not be used to validate parameters of public
methods. According to the Java Language Specification [[JLS 05]], section 14.10 "The assert
Statement":
Along similar lines, assertions should not be used for argument-checking in
public
methods. Argument-checking is typically part of the contract of a method, and this contract must be upheld whether assertions are enabled or disabled.Another problem with using assertions for argument checking is that erroneous arguments should result in an appropriate runtime exception (such as
IllegalArgumentException
,IndexOutOfBoundsException
orNullPointerException
). An assertion failure will not throw an appropriate exception. Again, it is not illegal to use assertions for argument checking onpublic
methods, but it is generally inappropriate.
Also, note that any defensive copying must be performed before validating the parameters and the checks must be performed on the copies instead of the original parameters. (See SER07-J. Make defensive copies of private mutable components)
Noncompliant Code Example
The method AbsAdd()
takes the absolute value of parameters x
and y
and returns their sum. It does not perform any validation on the input. The code snippet is vulnerable and can produce incorrect results as a result of integer overflow or because of a negative number being returned from the computation Math.abs(Integer.MIN_VALUE)
.
public static int AbsAdd(int x, int y) { return Math.abs(x) + Math.abs(y); } AbsAdd(Integer.MIN_VALUE,1);
Noncompliant Code Example
This noncompliant code example uses assertions to validate arguments of a public
method.
public static int AbsAdd(int x, int y) { assert x != Integer.MIN_VALUE; assert y != Integer.MIN_VALUE; assert ((x <= Integer.MAX_VALUE - y)); assert ((x >= Integer.MIN_VALUE - y)); return Math.abs(x) + Math.abs(y); }
Compliant Solution
This compliant solution validates the input to Math.abs()
to ensure it is not Integer.MIN_VALUE
and checks for arithmetic overflow. The result of the computation can also be stored in a long
variable to avoid overflow, however, in this case the upper bound of the addition is required to be representable as the type int
.
public static int AbsAdd(int x, int y) { if((x == Integer.MIN_VALUE || y == Integer.MIN_VALUE) || (x>0 && y>0 && (x > Integer.MAX_VALUE - y)) || (x<0 && y<0 && (x < Integer.MIN_VALUE - y))) throw new IllegalArgumentException(); return Math.abs(x) + Math.abs(y); }
Risk Assessment
Failing to validate method parameters can result in inconsistent computations, runtime exceptions and control flow vulnerabilities.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET02- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] 14.10 The assert Statement
[[Bloch 08]] Item 38: Check parameters for validity
[[ESA 05]] Rule 68: Explicitly check method parameters for validity, and throw an adequate exception in case they are not valid. Do not use the assert statement for this purpose
[[Daconta 03]] Item 7: My Assertions Are Not Gratuitous
MET01-J. Avoid ambiguous uses of overloading 12. Methods (MET)