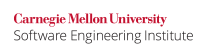
The serialization and deserialization features can be exploited to bypass security manager checks. A serializable class may install security manager checks in its constructors for various reasons. For example, the checks prevent untrusted code from modifying the internal state of the class.
Security manager checks must be replicated at all points where a class can be constructed. Because deserialization acts like a constructor, all the relevant methods must contain a security check.
If the class enables a caller to retrieve sensitive internal state contingent upon security checks, the same checks must be replicated during deserialization. This ensures that an attacker cannot glean sensitive information by deserializing the object.
Noncompliant Code Example
In this noncompliant code example, security manager checks are used within the constructor but are not replicated within the writeObject()
and readObject()
methods that are used in the serialization-deserialization process. This allows untrusted code to maliciously create instances of the class.
public final class CreditCard implements Serializable { // Private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; public CreditCard() { performSecurityManagerCheck(); // Initialize credit_card to default value credit_card = DEFAULT; } // Allows callers to modify (private) internal state public void changeCC(String newCC) { if (credit_card.equals(newCC)) { // No change return; } else { validateInput(newCC); credit_card = newCC; } } // readObject() correctly enforces checks during deserialization private void readObject(ObjectInputStream in) throws IOException { in.defaultReadObject(); // If the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { validateInput(credit_card); } } // Allows callers to retrieve internal state String getValue() { return credit_card; } // writeObject() correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) throws IOException { out.writeObject(credit_card); } }
Compliant Solution
This compliant solution implements the required security manager checks in all constructors and methods that can either modify or retrieve internal state. As a result, an attacker cannot create a modified instance of the object (using deserialization) or read the serialized byte stream to uncover sensitive serialized data.
public final class SecureCreditCard implements Serializable { // Private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; public SecureCreditCard() { performSecurityManagerCheck(); // Initialize credit_card to default value credit_card = DEFAULT; } //allows callers to modify (private) internal state public void changeCC(String newCC) { if (credit_card.equals(newCC)) { // No change return; } else { // Check permissions to modify credit_card performSecurityManagerCheck(); validateInput(newCC); credit_card = newCC; } } // readObject() correctly enforces checks during deserialization private void readObject(ObjectInputStream in) throws IOException { in.defaultReadObject(); // If the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { performSecurityManagerCheck(); validateInput(credit_card); } } // Allows callers to retrieve internal state public String getValue() { // Check permission to get value performSecurityManagerCheck(); return somePublicValue; } // writeObject() correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) throws IOException { // Duplicate check from getValue() performSecurityManagerCheck(); out.writeObject(credit_card); } }
Refer to the guideline SEC08-J. Enforce security checks in code that performs sensitive operations to learn about implementing the performSecurityManagerCheck()
method. As with SER04-J. Validate deserialized objects, it is important to protect against the finalizer attack.
Risk Assessment
Allowing serialization or deserialization to bypass the Security Manager may result in sensitive data being exposed or modified.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SER05- J |
high |
probable |
high |
P6 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 2007]] Guideline 5-3 Duplicate the SecurityManager checks enforced in a class during serialization and deserialization
[[Long 2005]] Section 2.4, Serialization
SER04-J. Validate deserialized objects 18. Serialization (SER) SER06-J. Do not serialize instances of inner classes