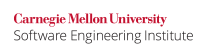
According to the Java API [[API 06]], interface java.util.concurrent.ExecutorService
, method shutdownNow()
documentation:
Attempts to stop all actively executing tasks, halts the processing of waiting tasks, and returns a list of the tasks that were awaiting execution. There are no guarantees beyond best-effort attempts to stop processing actively executing tasks. For example, typical implementations will cancel via Thread.interrupt(), so any task that fails to respond to interrupts may never terminate.
Consequently, care must be taken so that only interruptible tasks are submitted to the thread pool. When using worker threads that run untrusted tasks or dynamically loaded extension code, provide a way to interrupt the worker. For instance, a worker thread in a thread pool should generally have the following structure (adopted from [[Goetz 06]]):
public void run() { Throwable thrown = null; try { while (!isInterrupted()) runTask(getTaskFromWorkQueue()); } catch (Throwable e) { thrown = e; } finally { threadExited(this, thrown); // Notify upper layers } }
The worker thread based architecture that uses interruptible workers permits the caller to cleanly terminate misbehaving sub-tasks. If the sub-tasks die because of runtime exceptions, upper layers can take some suitable action such as replacing the worker thread when resources are constrained.
Noncompliant Code Example (shutting down thread pools)
This noncompliant code example uses the SocketReader
class defined earlier in Compliant Solution (close socket connection) of the guideline CON24-J. Ensure that threads and tasks performing blocking operations can be terminated and submits it as a task to a thread pool defined in class PoolService
.
final class PoolService { private final ExecutorService pool; public PoolService(int poolSize) { pool = Executors.newFixedThreadPool(poolSize); } public void doSomething() throws InterruptedException, IOException { pool.submit(new SocketReader()); // ... List<Runnable> awaitingTasks = pool.shutdownNow(); } public static void main(String[] args) throws InterruptedException, IOException { PoolService service = new PoolService(5); service.doSomething(); } } public final class SocketReader implements Runnable { private final Socket socket; // ... }
Because the task does not support interruption through the use of Thread.interrupted()
, there is no guarantee that the shutdownNow()
method will shutdown the thread pool. Using the shutdown()
method does not fix the problem either because it waits until all executing tasks have finished. Likewise, tasks that check a volatile
flag to determine whether it is safe to shutdown are not responsive to these methods.
Compliant Solution (submit interruptible tasks)
Tasks that do not support interruption using Thread.interrupt()
should not be submitted to a thread pool. This compliant solution submits the interruptible version of SocketReader
discussed in Compliant Solution (interruptible channel) of the guideline CON24-J. Ensure that threads and tasks performing blocking operations can be terminated, to the thread pool.
final class PoolService { // ... } public final class SocketReader implements Runnable { private final SocketChannel sc; // ... }
Similarly, when trying to cancel individual tasks within the thread pool using the Future.cancel()
method, ensure that the task supports interruption. If it does, pass a boolean
argument true
to cancel()
, otherwise pass false
. The value false
indicates that the task will be canceled if it has not already started.
Exceptions
EX1: Tasks that execute quickly and do not block may violate this guideline.
Risk Assessment
Submitting tasks that are not interruptible may preclude the shut down procedure of a thread pool and cause denial of service.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON36- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] interface ExecutorService
[[Goetz 06]] Chapter 7: Cancellation and shutdown
CON12-J. Avoid deadlock by requesting and releasing locks in the same order 11. Concurrency (CON) VOID CON14-J. Ensure atomicity of 64-bit operations