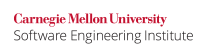
If a program relies on finalize()
to release system resources, or if there is confusion over which part of the program is responsible for releasing system resources, then there exists a possibility of a potential resource leak. In a busy system, there might be a time gap before the finalize()
method is called for an object. An attacker might exploit this vulnerability to induce a denial-of-service attack. The guideline OBJ08-J. Avoid using finalizers has more information on the demerits of using finalizers.
The Java garbage collector is called to free up unreleased memory. However, if the program relies on non-memory resources like file descriptors and database connections, unreleased resources might lead the program to prematurely exhaust its pool of resources. In addition, if the program uses resources like Lock
or Semaphore
, waiting for finalize()
to release the resources may result in resource starvation. Caching of object references in the output stream also implies that the objects will not be garbage collected unless the streams are closed promptly after use.
Also note that on the Windows platform, attempts to delete open files fail silently. See guideline FIO07-J. Do not create temporary files in shared directories for more information.
There is a similar guideline for releasing concurrency locks: guideline LCK08-J. Ensure actively held locks are released on exceptional conditions.
Noncompliant Code Example
The problem of resource pool exhaustion is aggravated in the case of database connections. Traditionally, database servers have allowed a fixed number of connections, depending on configuration and licensing. Failing to release database connections can result in rapid exhaustion of available connections. This noncompliant code example does not close the connection if an error occurs while executing the statement or while processing the results.
public void getResults(String sqlQuery) { try { Connection conn = getConnection(); Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery(sqlQuery); processResults(rs); stmt.close(); } catch (SQLException e) { /* forward to handler */ } }
Noncompliant Code Example
While being slightly better than the previous noncompliant code example, this code is also noncompliant. Both rs
and stmt
might be null
and the clean-up code in the finally block may result in a NullPointerException
.
Statement stmt = null; ResultSet rs = null Connection conn = getConnection(); try { stmt = conn.createStatement(); rs = stmt.executeQuery(sqlQuery); processResults(rs); } catch(SQLException e) { // forward to handler } finally { rs.close(); stmt.close(); }
Noncompliant Code Example
In this noncompliant code example, rs.close()
might itself result in a SQLException
, and so stmt.close()
will never be called.
Statement stmt = null; ResultSet rs = null; Connection conn = getConnection(); try { stmt = conn.createStatement(); rs = stmt.executeQuery(sqlQuery); processResults(rs); } catch(SQLException e) { // forward to handler } finally { if(rs != null) { rs.close(); } if(stmt != null) { stmt.close(); } }
Compliant Solution
This compliant solution ensures that resources are released as required.
Statement stmt = null; ResultSet rs = null; Connection conn = getConnection(); try { stmt = conn.createStatement(); rs = stmt.executeQuery(sqlQuery); processResults(rs); } catch(SQLException e) { // forward to handler } finally { try { if(rs != null) { rs.close(); } } finally { try { if(stmt != null) { stmt.close(); } } finally { conn.close(); } } }
Noncompliant Code Example
This noncompliant code example opens a file, uses it, but does not explicitly close the handle.
public int processFile(String fileName) throws IOException, FileNotFoundException { FileInputStream stream = new FileInputStream(fileName); BufferedReader bufRead = new BufferedReader(new InputStreamReader(stream)); String line; while((line = bufRead.readLine()) != null) { sendLine(line); } return 1; }
Compliant Solution
This compliant solution releases all acquired resources, regardless of any exceptions that might occur. Even though dereferencing bufRead
might result in an exception, if a FileInputStream
object is instantiated, it will be closed as required.
FileInputStream stream = null; BufferedReader bufRead = null; String line; try { stream = new FileInputStream(fileName); bufRead = new BufferedReader(new InputStreamReader(stream)); while((line = bufRead.readLine()) != null) { sendLine(line); } } catch (IOException e) { // forward to handler } finally { if(stream != null) { stream.close(); } }
Risk Assessment
Acquiring non-memory system resources and not releasing them explicitly may result in resource exhaustion.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO06-J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
The Coverity Prevent Version 5.0 RESOURCE_LEAK checker can detect the instances where there is leak of a socket resource or leak of a stream representing a file or other system resources.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as FIO42-C. Ensure files are properly closed when they are no longer needed.
This rule appears in the C++ Secure Coding Standard as FIO42-CPP. Ensure files are properly closed when they are no longer needed.
References
[[API 2006]] Class Object
[[Goetz 2006b]]
[[MITRE 2009]] CWE-405 "Asymmetric Resource Consumption (Amplification)", CWE-404
"Improper Resource Shutdown or Release", CWE-459
"Incomplete Cleanup," CWE-770
, "Allocation of Resources Without Limits or Throttling"
FIO05-J. Do not create multiple buffered wrappers on an InputStream 09. Input Output (FIO) FIO07-J. Do not create temporary files in shared directories