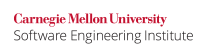
Java does not support the use of unsigned types, except for the 16 bit char
datatype. Sometimes, it is necessary to interoperate with native languages such as C or C++ that use unsigned types extensively. The standard practice to deal with unsigned input is to read values into Java's larger signed
primitives. For example, a signed long
can be used to hold an unsigned integer
obtained from native code.
Noncompliant Code Example
This example incorrectly uses a generic method for reading in integer data irrespective of the signedness. It assumes that the value is always signed and treats the most significant bit (MSB) as the sign bit causing misinterpretations about the actual sign and magnitude of the integer.
public static int getInteger(DataInputStream is) throws IOException { return is.readInt(); }
Compliant Solution
This compliant solution assumes that the unsigned
integer has 32 bits. It reads in an unsigned
integer value into a long
variable using the readInt()
method. If the read integer is unsigned
, the most significant bit may be turned on. Consequently, all the higher order bits of the resulting long
are set because of sign extension, and these must be masked off as demonstrated. For other integer sizes, the mask size should vary depending on the size of the unsigned
integer.
public static long getInteger2(DataInputStream is) throws IOException { return is.readInt() & 0xFFFFFFFFL; }
Risk Assessment
Treating an unsigned type as signed can result in misinterpretations and can lead to lost or misinterpreted data.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT01- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class DataInputStream: method readInt
[[Harold 97]] Chapter 2: Primitive Data Types, Cross Platform Issues, Unsigned Integers
INT00-J. Provide methods to read and write Little-Endian data 05. Integers (INT) INT30-J. Range check before casting integers to narrower types