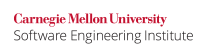
The Object.wait()
method is used to temporarily cede possession of a lock so that another requesting thread can proceed. It must always be used inside a synchronized
block or method. To let the waiting thread resume, the requesting thread must notify it. Furthermore, the wait()
method should be invoked in a loop that checks if a condition predicate holds. The correct way to invoke wait()
is shown below.
synchronized (object) { while (<condition does not hold>) { object.wait(); } // Proceed when condition holds }
The invocation of notify()
or notifyAll()
in another thread cannot precisely determine which waiting thread must be resumed. A condition predicate statement is used so that the correct thread resumes when it receives a notification. A condition predicate also helps when a thread is required to block until a condition becomes true, for instance, when it should not proceed without reading some data from an input stream.
Two properties come into the picture:
Safety: Its main goal is to ensure that all objects maintain consistent states in a multi-threaded environment. [[Lea 00]]
- Liveness: Every operation or method invocation executes to completion without interruptions, even if it goes against safety.
To guarantee liveness, the while
loop condition should be tested before invoking the wait()
method. This is because the condition might have already been made true by some other thread which indicates that a notify signal may have already been sent from the other thread. Invoking the wait()
method after the notify signal has been sent is futile and results in an infinitely blocked state.
To guarantee safety, the while
loop condition must be tested even after invoking wait()
. While wait()
is meant to block indefinitely until a notification is received, this practice is recommended because: [[Bloch 01]]
- Thread in the middle: A third thread can acquire the lock on the shared object during the interval between a notification being sent and the receiving thread resuming execution. This thread can change the state of the object leaving it inconsistent. This is a time of check, time of use (TOCTOU) condition.
- Malicious notifications: There is no guarantee that a random notification will not be received when the condition predicate evaluates to false. This means that the invocation of
wait()
may be nullified by the notification. - Mis-delivered notification: Sometimes on receipt of a
notifyAll()
signal, an unrelated thread can start executing and it is possible for its condition predicate to be true. Consequently, it may resume execution whilst it was required to remain dormant. Spurious wakeups: Certain JVM implementations are vulnerable to spurious wakeups that result in waiting threads waking up even without a notification [[API 06]].
Because of these reasons, it is indispensable to check the condition after wait()
is invoked. A while loop is the best choice to check the condition before and after invoking wait()
.
Similarly, the await()
method of interface Condition
must also be invoked inside a loop. According to the Java API [[API 06]], Interface Condition
:
When waiting upon a Condition, a "spurious wakeup" is permitted to occur, in general, as a concession to the underlying platform semantics. This has little practical impact on most application programs as a Condition should always be waited upon in a loop, testing the state predicate that is being waited for. An implementation is free to remove the possibility of spurious wakeups but it is recommended that applications programmers always assume that they can occur and so always wait in a loop.
Newer code should use the java.util.concurrent
concurrency utilities as opposed to the wait/notify mechanism, however, legacy code may require these methods.
Noncompliant Code Example
This noncompliant code example invokes the wait()
method inside a traditional if
block and fails to check the post condition after the notification (accidental or malicious) is received. This means that the thread can wake up when it is not supposed to.
synchronized(object) { if(<condition does not hold>) object.wait(); // Proceed when condition holds }
Compliant Solution
This compliant solution encloses the wait()
method in a while
loop and as a result checks the condition during both pre and post wait()
invocation times.
synchronized (object) { while (<condition does not hold>) { object.wait(); } // Proceed when condition holds }
Similarly, if the await()
method of the java.util.concurrent.locks.Condition
interface is implemented, it should be enclosed in a loop.
Risk Assessment
To guarantee liveness and safety, the wait()
and await()
methods should always be called inside a while
loop.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON18- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Object
[[Bloch 01]] Item 50: Never invoke wait outside a loop
[[Lea 00]] 3.2.2 Monitor Mechanics, 1.3.2 Liveness
[[Goetz 06]] Section 14.2, Using Condition Queues
CON17-J. Avoid using ThreadGroup APIs 11. Concurrency (CON) CON19-J. Notify all waiting threads instead of a single thread