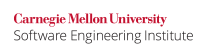
Sensitive data is vulnerable to compromise. An adversary who has control of the file system may be able to access such data if the application:
- uses objects to store sensitive data whose contents are not cleared or garbage-collected after use
- has memory pages that can be swapped out to disk as required by the operating system (e.g., to perform memory management tasks or to support hibernation)
- holds sensitive data in a buffer (such as
BufferedReader
) that retains copies of the data in the OS cache or in memory - bases its control flow on reflection that allows circumventing countermeasures to limit the lifetime of sensitive variables
- reveals sensitive data in debugging messages, log files, environment variables, or through thread and core dumps
Using such attacks to compromise sensitive data is far easier for live data than for data that has been cleared or reclaimed. Sensitive data that remains live beyond the minimum period required for its use has an unnecessarily large window of vulnerability. Consequently, programs must minimize the lifetime of sensitive data.
Currently, complete mitigation requires support from the underlying operating system. For instance, if swapping sensitive data out to disk is an issue, a secure operating system that disables swapping and hibernation is indispensable.
Noncompliant Code Example
This noncompliant code example reads login information from the console and stores the password as a String
object. The credentials remain exposed until the garbage collector reclaims the memory associated with the String
objects.
class Password { public static void main (String args[]) throws IOException { Console c = System.console(); if (c == null) { System.err.println("No console."); System.exit(1); } String login = c.readLine("Enter your user name: "); String password = c.readLine("Enter your password: "); if (!verify(login, password)) { throw new IOException("Invalid Credentials"); } // ... } // Dummy verify method, always returns true private static final boolean verify(String login, String password) { return true; } }
Compliant Solution
This compliant solution uses the Console.readPassword()
method to obtain the password from the console. This method allows the password to be returned as a sequence of characters rather than as a String
object. Consequently, the programmer can clear the password from the array immediately after use. The method also disables echoing of the password to the console.
class Password { public static void main (String args[]) throws IOException { Console c = System.console(); if (c == null) { System.err.println("No console."); System.exit(1); } String login = c.readLine("Enter your user name: "); char [] password = c.readPassword("Enter your password: "); if (!verify(login, password)) { throw new IOException("Invalid Credentials"); } // Clear the password Arrays.fill(password, ' '); } // Dummy verify method, always returns true private static final boolean verify(String login, char[] password) { return true; } }
Noncompliant Code Example
This noncompliant code example uses a BufferedReader
to wrap an InputStreamReader
object so that sensitive data can be read from a file.
BufferedReader br = new BufferedReader(new InputStreamReader( new FileInputStream("file"))); // Read from the file
Compliant Solution
This compliant solution uses a directly allocated NIO (new I/O) buffer to read sensitive data from the file. The data can be cleared immediately after use and is not cached or buffered at multiple locations. It exists only in the system memory.
private void readIntoDirectBuffer() throws IOException { ByteBuffer buffer = ByteBuffer.allocateDirect(16 * 1024); FileChannel rdr = (new FileInputStream("file")).getChannel(); while(rdr.read(buffer) > 0) { // Do something with the buffer buffer.clear(); } rdr.close(); }
Note that manual clearing of the buffer data is mandatory because direct buffers are exempt from garbage collection.
Applicability
Failure to limit the lifetime of sensitive data can lead to information leaks.
This rule may be violated when both of the following are true:
1. It can be proved that the code is free from other errors that can expose the sensitive data, and
2. Attackers lack physical access to the target machine.
Related Guidelines
MITRE 2009 | CWE ID 524 "Information Exposure through Caching" |
CWE ID 528 "Exposure of Core Dump File to an Unauthorized Control Sphere" | |
CWE ID 215 "Information Exposure through Debug Information" | |
CWE ID 534 "Information Exposure through Debug Log Files" | |
CWE ID 526 "Information Exposure through Environmental Variables" | |
CWE ID 226 "Sensitive Information Uncleared before Release" |
Bibliography
[API 2011] Class ByteBuffer
[Oracle 2012] Reading ASCII Passwords From an InputStream Example (Java Cryptography Architecture (JCA) Reference Guide)
[Tutorials 2008] I/O from the Command Line