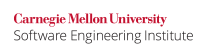
If a while
or for
statement uses a loop counter, and increments or decrements the counter by more than one, it must use an inequality operator (that is, <
, <=
, >
, or >=
) to terminate the loop. This prevents the loop from executing indefinitely or until the counter wraps around and reaches the final value. (See NUM00-J. Detect or prevent integer overflow.)
Noncompliant Code Example
This noncompliant code example appears to iterate five times.
for (i = 1; i != 10; i += 2) { // ... }
However, the loop never terminates! Successive values of i
are 1, 3, 5, 7, 9, 11, etc.; the comparison with 10 never evaluates to true
. The value reaches the maximum representable positive number (Integer.MAX_VALUE
), then wraps to the second lowest negative number (Integer.MIN_VALUE
+ 1). It then works its way up to -1, then 1, and proceeds as described earlier.
Noncompliant Code Example
This noncompliant code example terminates but performs more iterations than expected.
for (i = 1; i != 10; i += 5) { // ... }
Successive values of i
are 1, 6, and 11, skipping 10. The value of i
then wraps from near the maximum positive value to near the lowest negative value and works its way up toward zero. It then assumes 2, 7, and 12, skipping 10 again. After the value wraps from the high positive to the low negative side three more times, it finally reaches 0, 5, and 10, terminating the loop.
Compliant Solution
Using an inequality operator guarantees proper loop termination.
for (i = 1; i <= 10; i += 2) { // ... }
Noncompliant Code Example
Inequality operators fail to ensure loop termination when comparing with Integer.MAX_VALUE
or Integer.MIN_VALUE
.
for (i = 1; i <= Integer.MAX_VALUE; i += 2) { // ... }
This usually happens when the step size is more than 1.
Compliant Solution
It is insufficient to compare with Integer.MAX_VALUE - 1
when the loop increment is greater than 1. To be compliant, ensure that the comparison is carried out with (Integer.MAX_VALUE
minus the step's value).
for (i = 1; i <= Integer.MAX_VALUE - 2; i += 2) { // ... }
Applicability
Testing for exact values to terminate a loop may result in infinite loops and denial of service.