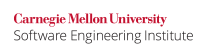
Declaring shared variables as volatile ensures visibility and limits reordering of accesses. Volatile accesses do not guarantee the atomicity of composite operations such as incrementing a variable. Consequently, this recommendation is not applicable in cases where the atomicity of composite operations must be guaranteed (see CON01-J. Do not assume that composite operations on primitive data are atomic).
Declaring variables as volatile establishes a happens-before relationship such that a write to the volatile variable is always seen by a subsequent read. Statements that occur before the write to the volatile field also happen-before the read of the volatile field.
Consider two threads that are executing some statements:
Thread 1 and Thread 2 have a happens-before relationship such that Thread 2 does not start before Thread 1 finishes. This is established by the semantics of volatile accesses.
In this example, Statement 3 writes to a volatile variable, and statement 4 (in Thread 2) reads the same volatile variable. The read sees the most recent write (to the same variable v
) from statement 3.
Volatile read and write operations cannot be reordered with respect to each other and also with respect to nonvolatile variables accesses. When Thread 2 reads the volatile variable it sees the results of all the writes occurring before the write to the volatile variable in Thread 1. Because of the relatively strong guarantees of volatile, the performance overhead of volatile is almost the same as that of synchronization
However, this does not mean that statements 1 and 2 are executed in the order in which they appear in the program. They may be freely reordered by the compiler.
The possible reorderings between volatile and non-volatile variables are summarized in the matrix shown below. Load and store operations are synonymous to read and write operations, respectively. [[Lea 08]]
Noncompliant Code Example (status flag)
This noncompliant code example uses a shutdown()
method to set a non-volatile done
flag that is checked in the run()
method. If one thread invokes the shutdown()
method to set the flag, it is possible that another thread might not observe this change. Consequently, the second thread may still observe that done
is false
and incorrectly invoke the sleep()
method.
final class ControlledStop implements Runnable { private boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } protected void shutdown(){ done = true; } }
Compliant Solution (volatile
status flag)
This compliant solution qualifies the done
flag as volatile
so that updates are visible to other threads.
final class ControlledStop implements Runnable { private volatile boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } protected void shutdown(){ done = true; } }
Noncompliant Code Example (non-volatile guard)
This noncompliant code example declares a non-volatile variable of type int
which is initialized in the constructor depending on a security check.
class BankOperation { private int balance = 0; private boolean initialized = false; public BankOperation() { if (!performAccountVerification()) { throw new SecurityException("Invalid Account"); } balance = 1000; initialized = true; } private int getBalance() { if (initialized == true) { return balance; } else { return -1; } } }
The Java compiler is allowed to reorder the statements of the BankOperation()
constructor so that the boolean
flag initialized
is set to true
before the initialization has concluded. If it is possible to obtain a partially initialized instance of the class in a subclass using a finalizer attack (see OBJ04-J. Do not allow partially initialized objects to be accessed), a race condition can be exploited by invoking the getBalance()
method to obtain the balance even though initialization is still underway.
Compliant Solution (volatile guard)
This compliant solution declares the initialized
flag as volatile to ensure that initialization happens before the initialized
flag is set.
class BankOperation { private int balance = 0; private volatile boolean initialized = false; // Now volatile public BankOperation() { if (!performAccountVerification()) { throw new SecurityException("Invalid Account"); } balance = 1000; initialized = true; } private int getBalance() { if (initialized == true) { return balance; } else { return -1; } } }
Noncompliant Code Example (visibility)
This noncompliant code example consists of two classes: an immutable ImmutablePoint
class and a mutable Holder
class. Holder
is mutable because a new ImmutablePoint
instance can be assigned to it using the setPoint()
method (see CON09-J. Do not assume that classes having only immutable members are thread-safe).
class Holder { ImmutablePoint ipoint; Holder(ImmutablePoint ip) { ipoint = ip; } ImmutablePoint getPoint() { return ipoint; } void setPoint(ImmutablePoint ip) { this.ipoint = ip; } } public class ImmutablePoint { final int x; final int y; public ImmutablePoint(int x, int y) { this.x = x; this.y = y; } }
Because the ipoint
field is non-volatile, changes to this value may not be immediately visible to other threads.
Compliant Solution (visibility)
This compliant solution declares the ipoint
field as volatile so that updates are immediately visible to other threads.
class Holder { volatile ImmutablePoint ipoint; Holder(ImmutablePoint ip) { ipoint = ip; } ImmutablePoint getPoint() { return ipoint; } void setPoint(ImmutablePoint ip) { this.ipoint = ip; } }
The setPoint()
method does not need to be synchronized because it operates atomically on immutable data, that is, on an instance of ImmutablePoint
.
Declaring immutable fields as volatile enables their safe publication in that, once published, it is impossible to change the state of the sub-object.
Noncompliant Code Example (partial initialization)
Thread-safe classes (which may not be strictly immutable), such as Container
in this noncompliant code example, must not declare fields as non-final and non-volatile (see CON09-J. Do not assume that classes having only immutable members are thread-safe). Non-final, non-volatile fields may be observed by other threads before the sub-objects' initialization has concluded.
This noncompliant code example fails to declare the map
field as volatile or final. Consequently, a thread that invokes the get()
method may observe the value of the map
field before initialization has concluded.
public class Container<K,V> { Map<K,V> map; public synchronized void initialize() { if (map == null) { map = new HashMap<K,V>(); // Fill some useful values into HashMap } } public V get(Object k) { if (map != null) { return map.get(k); } else { return null; } } }
Compliant Solution (proper initialization)
This compliant solution declares the map
field as volatile to ensure other threads see an up-to-date HashMap
reference.
public class Container<K,V> { volatile Map<K,V> map; public synchronized void initialize() { if (map == null) { map = new HashMap<K,V>(); // Fill some useful values into HashMap } } public V get(Object k) { if (map != null) { return map.get(k); } else { return null; } } }
Alternative solutions to using volatile
for safe publication are described in [CON26-J. Do not publish partially-constructed objects]. These alternative solutions are recommended if the values of the map
can be mutated after initialization because the use of volatile
only guarantees "one time safe publication" [[Goetz 06]], that is the reference is made visible only after initialization. There is no guarantee that any future updates to the map's contents will be visible immediately (see [CON11-J. Do not assume that declaring an object volatile guarantees visibility of its members] for more information).
Risk Assessment
Failing to use volatile to guarantee visibility of shared values across multiple thread and prevent reordering of accesses can result in unpredictable control flow.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON00- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[Goetz 06]] 3.4.2. "Example: Using Volatile to Publish Immutable Objects"
[[JPL 06]] 14.10.3. "The Happens-Before Relationship"
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object