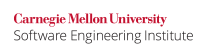
All good things must come to an end, and so do threads when they exit normally. Unfortunately, programmers often try to forcefully terminate threads when they believe that the task has been accomplished, the request has been canceled or the program needs to quickly shutdown.
A few APIs were introduced to facilitate thread suspension, resumption and termination but were later deprecated due to inherent design weaknesses. The Thread.stop
method is one such example. It was intended to throw a ThreadDeath
exception. Two cases arise:
- If
ThreadDeath
is left uncaught, it allows the execution of afinally
block which performs the usual cleanup operations. This is not a good idea because of two reasons. First, no thread can be forcefully stopped since an arbitrary thread can catch the thrown exception and simply choose to ignore it. Second, stopping threads leads to the release of all held monitors violating the guarantees provided by the critical sections. Moreover, the damaged objects end up in an inconsistent state with arbitrary behavior being a typical outcome.
- As a remediation measure, catching
ThreadDeath
on the other hand can itself ensnarl multithreaded code. For one, this exception can be thrown anywhere making it difficult to trace and recover effectively. Also, there is nothing stopping a thread from throwing anotherThreadDeath
exception while recovery is in progress.
Noncompliant Code Example
This noncompliant code example shows how a thread forcefully comes to a halt when the Thread.stop
method is invoked. Neither the catch
nor the finally
block is executed. Needless to say, any held monitors will be immediately released leaving the object in a delicate state.
class BadStop implements Runnable { public void run() { try { Thread.currentThread().sleep(1000); }catch(InterruptedException ie) { System.out.println("Performing cleanup"); } // not executed finally { System.out.println("Closing resources"); } // not executed System.out.println("Done!"); } } class Controller { public static void main(String[] args) { Thread t = new Thread(new BadStop()); t.start(); t.interrupt(); // artificially induce an InterruptedException t.stop(); // force thread cancellation } }
Compliant Solution (1)
This compliant example uses a boolean
flag called done
to indicate whether the thread should be stopped after any necessary cleanup code has finished executing. An accessor method shutdown()
is used to set the flag to true
upon which the thread will start the cancellation process. The done
flag has also been set immediately following the execution of the initial finally
block statements so that the system does not continue to relinquish the resources that it has already released, in the event of done
staying false
.
class ControlledStop implements Runnable{ protected volatile boolean done = false; public void run() { while(!done) { try { Thread.currentThread().sleep(1000); }catch(InterruptedException ie) { System.out.println("Performing cleanup"); } finally { System.out.println("Closing resources"); done = true; } } System.out.println("Done!"); } protected void shutdown(){ done = true; } } class Controller { public static void main(String[] args) throws InterruptedException { ControlledStop c = new ControlledStop(); Thread t = new Thread(c); t.start(); t.interrupt(); // artificially induce an InterruptedException Thread.sleep(1000); // wait for some time to allow the exception to be caught (demonstration only) c.shutdown(); } }
Compliant Solution (2)
Remove the default java.lang.RuntimePermission
"stopThread" from the policy file used by the security manager to deny the Thread.stop
invoking code, the required privileges.
Noncompliant Code Example
This noncompliant solution uses the advice suggested in the previous compliant solution. Unfortunately, this does not help in terminating the thread since it is blocked on some network IO due to the readLine
method. The boolean flag trick will thus not work in such cases; a good alternative method to end the thread is required.
class StopSocket extends Thread { protected Socket s; protected volatile boolean done = false; public void run() { while(!done) { try { s = new Socket("somehost",25); BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream())); String s = null; while((s = br.readLine()) != null) { // blocks until end of stream (null) } System.out.println("Blocked, will not get executed until some data is received. " + s); }catch (IOException ie) { System.out.println("Performing cleanup"); } finally { System.out.println("Closing resources"); done = true; } } } public void shutdown() throws IOException { done = true; } } class Controller { public static void main(String[] args) throws InterruptedException, IOException { StopSocket ss = new StopSocket(); Thread t = new Thread(ss); t.start(); Thread.sleep(1000); ss.shutdown(); } }
Compliant Solution
The compliant solution simply closes the socket connection, both using the shutdown
method as well as in the finally
block. Thus, the thread is bound to stop due to a socketException
. Note that there is no way to keep the connection alive if the thread is to be cleanly halted immediately.
class StopSocket extends Thread { protected Socket s; public void run() { try { s = new Socket("somehost",25); BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream())); String s = null; while((s = br.readLine()) != null) { // blocks until end of stream (null) } System.out.println("Blocked, will not get executed until some data is received. " + s); }catch (IOException ie) { System.out.println("Performing cleanup"); } finally { System.out.println("Closing resources"); try { if(s != null) s.close(); } catch (IOException e) { e.printStackTrace(); } } } public void shutdown() throws IOException { if(s != null) s.close(); } } class Controller { public static void main(String[] args) throws InterruptedException, IOException { StopSocket ss = new StopSocket(); Thread t = new Thread(ss); t.start(); Thread.sleep(1000); ss.shutdown(); } }
Risk Assessment
Trying to force thread shutdown can lead to inconsistent object state and thus corrupt the object. Critical resources may also leak if cleanup operations are not carried out as required.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON35-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Thread, method stop
[[Darwin 04]] 24.3 Stopping a Thread
[[JDK7 08]] Concurrency Utilities, More information: Java Thread Primitive Deprecation
[[JPL 05]] 14.12.1. Don't stop
CON34-J. Avoid deadlock by requesting fine-grained locks in the proper order 08. Concurrency (CON) 08. Concurrency (CON)