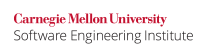
According to Sun's Secure Coding Guidelines [[SCG 2007]]
The (Java) language is type-safe, and the runtime provides automatic memory management and range-checking on arrays. These features also make Java programs immune to the stack-smashing and buffer overflow attacks possible in the C and C++ programming languages, and that have been described as the single most pernicious problem in computer security today.
While this statement is true, arithmetic operations in the Java platform require as much caution as in C and C++. Integer operations can result in overflow because Java does not provide any indication of overflow conditions and silently wraps. While integer overflows in vulnerable C and C++ programs may result in execution of arbitrary code, in Java, wrapped values typically result in incorrect computations and unanticipated outcomes.
According to the Java Language Specification Section 4.2.2 "Integer Operations"
The built-in integer operators do not indicate overflow or underflow in any way. Integer operators can throw a
NullPointerException
if unboxing conversion of anull
reference is required. Other than that, the only integer operators that can throw an exception are the integer divide operator/
and the integer remainder operator%
, which throw anArithmeticException
if the right-hand operand is zero, and the increment and decrement operators ++ and -- which can throw anOutOfMemoryError
if boxing conversion is required and there is not sufficient memory available to perform the conversion.
The integral types in Java are byte
, short
, int
, and long
, whose values are 8-bit, 16-bit, 32-bit and 64-bit signed twoâs-complement integers, respectively, and char
, whose values are 16-bit unsigned integers representing UTF-16 code units.
According to the Java Language Specification Section 4.2.1 "Integral Types and Values," the values of the integral types are integers in the following ranges:
- For byte, from â“128 to 127, inclusive
- For short, from â“32,768 to 32,767, inclusive
- For int, from â“2,147,483,648 to 2,147,483,647
- For long, from â“9,223,372,036,854,775,808 to 9,223,372,036,854,775,807, inclusive
- For char, from
\u0000
to\uffff
inclusive, that is, from 0 to 65,535
The table below shows the integer overflow behavior of the integral operators.
Operator |
Overflow |
|
Operator |
Overflow |
|
Operator |
Overflow |
|
Operator |
Overflow |
---|---|---|---|---|---|---|---|---|---|---|
yes |
|
yes |
|
no |
|
|
no |
|||
yes |
|
yes |
|
|
no |
|
|
no |
||
yes |
|
yes |
|
|
no |
|
|
no |
||
yes |
|
no |
|
\ |
no |
|
|
no |
||
no |
|
no |
|
|
no |
|
|
no |
||
|
yes |
|
|
no |
|
|
no |
|
|
no |
|
yes |
|
|
no |
|
|
no |
|
|
no |
|
no |
|
|
no |
|
un |
no |
|
|| |
no |
yes |
|
|
no |
|
yes |
|
|
no |
Failure to account for integer overflow has resulted in failures of real systems, for instance, when implementing the compareTo()
method. The meaning of the return value of the compareTo()
method is defined only in terms of its sign and whether it is zero; the magnitude of the return value is irrelevant. Consequently, an apparent — but incorrect — optimization would be to subtract the operands and return the result. For operands of opposite sign, this can result in integer overflow; consequently violating the compareTo()
contract [[Bloch 2008, item 12]].
Addition
Addition (as with all arithmetic operations) in Java is performed on signed numbers only; unsigned numbers are unsupported. One exception is the unsigned char
type. Performing arithmetic operations that use operands of type char
is strongly discouraged.
Noncompliant Code Example
In this noncompliant code example, the result of the addition can overflow.
public int do_operation(int a, int b){ // May result in overflow int temp = a + b; return temp; }
When the result of the addition is outside the range that can be represented as an int
, the variable temp
will contain an erroneous result. This problem does not occur when using short types, such as byte
and short
because the operands are promoted to type int
before the operation is carried out. The language disallows storing the result of such operations in variables of types shorter than type int
.
Compliant Solution (Bounds Checking)
Explicitly check the range of the operands of arithmetic operations; throw an ArithmeticException
when overflow would occur.
Compliant Solution (Use a larger type and downcast)
For all integral types other than long
, the next larger integral type can represent the result of any single integral operation. For example, operations on values of type int
, can be safely performed using type long
. Therefore, we can perform an operation using the larger type and range-check before down casting to the original type. Note, however, that this guarantee holds only for a one arithmetic operation; larger expressions without per-operation bounds checks may overflow the larger type.
Because type long
is the largest primitive integral type, the only possible way to use a larger type and downcast is to perform arithmetic operations using the BigInteger
class, range-check, and then convert the result back to type long
.
This compliant solution converts two variables of type int
to type long
, performs the addition of the long
values, and range checks the result before converting back to type int
using a range-checking method. The range-checking method determines whether its input can be represented by type int
. If so, it returns the downcast result; otherwise it throws an ArithmeticException
.
public int do_operation(int a, int b) throws ArithmeticException { return intRangeCheck((long) a + (long) b); } // Either perform a safe downcast to int, or throw ArithmeticException public static int intRangeCheck(long val) throws ArithmeticException { if (val > Integer.MAX_VALUE || val < Integer.MIN_VALUE) { throw new ArithmeticException("Out of range"); } return (int)val; // Value within range; downcast is safe }
Compliant Solution (Bounds Checking)
This compliant solution range checks the operand values to ensure that the result does not overflow.
public int add(int a, int b) throws ArithmeticException { if( b > 0 ? a > Integer.MAX_VALUE - b : a < Integer.MIN_VALUE - b ) { throw new ArithmeticException("Not in range"); } return a + b; // Value within range so addition can be performed }
public int add(int a, int b) throws ArithmeticException { if (((a > 0) && (b > 0) && (a > (Integer.MAX_VALUE - b))) || ((a < 0) && (b < 0) && (a < (Integer.MIN_VALUE - b)))) { throw new ArithmeticException("Not in range"); } else { return a + b; // Value within range so addition can be performed }
Compliant Solution (Use BigInteger Class)
This compliant solution uses the BigInteger
class as a wrapper to test for overflow.
public long do_operation(long a, long b) throws ArithmeticException { return longRangeCheck(BigInteger.valueOf(a).add(BigInteger.valueOf(b)); } public long longRangeCheck(BigInteger val) throws ArithmeticException { if (val.compareTo(BigInteger.valueOf(Long.MAX_VALUE)) == 1 || val.compareTo(BigInteger.valueOf(Long.MIN_VALUE)) == -1) { throw new ArithmeticException("Out of range for long"); } return val.longValue(); }
The BigInteger
class eliminates integer overflows but at the cost of increased overhead.
Subtraction
Subtraction overflows when the first operand is a negative integer and the second operand is a large positive integer such that their difference is not representable as a value of type int
. Subtraction also overflows when the first operand is positive and the second operand is negative and their difference is not representable as a value of type int
.
Note that the "Use a larger type and downcast" approach suffices to avoid overflow for subtraction.
Multiplication
Multiplication overflows whenever the sum of the number of bits required to represent its operands is larger than the number of bits in the result type. Once again, the "Use a larger type and downcast" approach suffices to avoid overflow.
Division
Although Java throws a java.lang.ArithmeticException
for division by zero, it fails to do so when dividing Integer.MIN_VALUE
by -1. Rather, Java produces Integer.MIN_VALUE
in this case, because the result is -(Integer.MIN_VALUE) = Integer.MAX_VALUE + 1
)) which overflows to Integer.MIN_VALUE
; this may surprise many programmers.
Once again, the "Use a larger type and downcast" approach suffices to avoid overflow. In some cases, checking for the specific case above may be more efficient.
Remainder Operator
The Java remainder operator does not present overflow issues. However, it has the following behavior for corner cases:
- When the modulo of
Integer.MIN_VALUE
with -1 is taken, the result is always 0.
- When the right-hand operand is zero, the integer remainder operator % will throw an
ArithmeticException
.
- The sign of the remainder is always the same as that of the dividend. For example,
-3
%-2
results in the value-1
. This behavior may be unexpected.
Refer to guideline INT02-J. Remember that the remainder operator may return a negative result value for more details.
Unary Negation
The result of negating Integer.MIN_VALUE
is Integer.MIN_VALUE
, because -Integer.MIN_VALUE
is logically equivalent to Integer.MAX_VALUE+1
which overflows to Integer.MIN_VALUE
.
Once again, the "Use a larger type and downcast" approach suffices to avoid overflow. In some cases, checking for the specific case above may be more efficient.
Absolute Value
A related pitfall is the use of the Math.abs()
method that takes a parameter of type int
and returns its absolute value. Because of the asymmetry between the two's complement representation of negative and positive integer values (Integer.MAX_VALUE
is 2147483647 and Integer.MIN_VALUE
is -2,147,483,648, which means there is one more negative integer than positive integers), there is no equivalent positive value (+2,147,483,648) for Integer.MIN_VALUE
. Consequenly, the Math.abs()
returns Integer.MIN_VALUE
when the value of its argument is Integer.MIN_VALUE
; this may surprise many programmers.
Once again, the "Use a larger type and downcast" approach suffices to avoid overflow. In some cases, checking for the specific case above may be more efficient.
Shifting
The shift operation in Java has the following properties:
- The right shift is an arithmetic shift.
- The types
boolean, float and double
cannot use the bit shifting operators.
- If the value to be shifted is of type
int
, only the five lowest-order bits of the right-hand operand are used as the shift distance. That is, the shift distance is the value of the right-hand operand masked by 31 (0x1F). This results in a value modulo 31, inclusive.
When the value to be shifted (left-operand) is of type
long
, only the last 6 bits of the right-hand operand are used to perform the shift. The shift distance is the value of the right-hand operand masked by 63 (0x3D) [[JLS 2003]]. (That is to say, the shift value is always between 0 and 63. If the shift value is greater than 64, then the shift isvalue % 64
.)
Refer to guideline INT05-J. Use shift operators correctly for further details about the behavior of the shift operators.
Noncompliant Code Example
This noncompliant code example attempts to shift the value i
of type int
until, after 32 iterations, the value becomes 0. Unfortunately, this loop never terminates because an attempt to shift a value of type int
by 32 bits results in the original value rather than the value 0 [[Bloch 2005]].
int i = 0; while ((-1 << i) != 0) i++;
Compliant Solution
This compliant solution initially sets the value val
to -1 and repeatedly shifts the value by one place on each successive iteration.
for (int val = -1; val != 0; val <<= 1) { /* ... */ }
Noncompliant Code Example (Concurrent Code)
This noncompliant code example uses an AtomicInteger
which is part of the concurrency utilities. The concurrency utilities do not enforce checks for integer overflow.
class InventoryManager { private final AtomicInteger itemsInInventory = new AtomicInteger(100); //... public final void returnItem() { itemsInInventory++; } }
Consequently, itemsInInventory
may wrap around to Integer.MIN_VALUE
after the increment operation.
Noncompliant Code Example (Concurrent Codeâ”TOCTOU Condition in Check)
This noncompliant code example installs a check for integer overflow; however, there is a time-of-check-time-of-use vulnerability between the check and the increment operation.
class InventoryManager { private volatile int itemsInInventory = 100; // ... public final void returnItem() { if (itemsInInventory == Integer.MAX_VALUE) { throw new IllegalStateException("Out of bounds"); } itemsInInventory++; } }
Compliant Solution (java.util.concurrent.atomic classes
)
The java.util.concurrent
utilities can be used to atomically manipulate a shared variable. This compliant solution defines itemsInInventory
as a java.util.concurrent.atomic.AtomicInteger
variable, allowing composite operations to be performed atomically.
class InventoryManager { private final AtomicInteger itemsInInventory = new AtomicInteger(100); public final void returnItem() { while (true) { int old = itemsInInventory.get(); if (old == Integer.MAX_VALUE) { throw new IllegalStateException("Out of bounds"); } int next = old + 1; // Increment if (itemsInInventory.compareAndSet(old, next)) { break; } } // end while } // end removeItem() }
The compareAndSet()
method takes two arguments, the expected value of a variable when the method is invoked and the updated value. This compliant solution uses this method to atomically set the value of itemsInInventory
to the updated value if and only if the current value equals the expected value [[API 2006]]. The while loop ensures that the removeItem()
method succeeds in decrementing the most recent value of itemsInInventory
as long as the inventory count is greater than MIN_INVENTORY
. Refer to guideline VNA02-J. Ensure that compound operations on shared variables are atomic for more details.
Exceptions
INT00-EX1: Depending on circumstances, integer overflow may be benign. For instance, the Object.hashcode()
method may return all representable values of type int
; further, many algorithms for computing hashcodes intentionally allow overflow to occur.
INT00-EX2: The added complexity and cost of programmer-written overflow checks may exceed their value for all but the most-critical code. In such cases, consider the alternative of treating integral values as though they are tainted data, using appropriate range checks as the notional "sanitizing" code. These range checks should ensure that incoming values cannot cause integer overflow. Note that sound determination of allowable ranges may require deep understanding of the details of the code protected by the range checks; this is non-trivial.
Risk Assessment
Failure to perform appropriate range checking can lead to integer overflows, which may cause unexpected program control flow or unanticipated program behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT00-J |
medium |
unlikely |
medium |
P4 |
L3 |
Automated Detection
Automated detection of integer operations that may potentially overflow is straightforward. Automatic determination of which potential overflows are true errors and which are intended by the programmer is infeasible. Heuristic warnings may be helpful.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Other Languages
This guideline appears in the C Secure Coding Standard as INT32-C. Ensure that operations on signed integers do not result in overflow.
This guideline appears in the C++ Secure Coding Standard as INT32-CPP. Ensure that operations on signed integers do not result in overflow.
Bibliography
[[Bloch 2005]] Puzzle 27: Shifty i's[[SCG 2007]] Introduction
[[JLS 2003]] 4.2.2 Integer Operations and 15.22 Bitwise and Logical Operators
[[MITRE 2009]] CWE ID 682 "Incorrect Calculation", CWE ID 190
"Integer Overflow or Wraparound", CWE ID 191
"Integer Underflow (Wrap or Wraparound)"
[[Seacord 2005]] Chapter 5. Integers
[[Tutorials 2008]] Primitive Data Types
06. Integers (INT) 06. Integers (INT) INT01-J. Check ranges before casting integers to narrower types