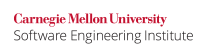
According to the Java Language Specification §15.7, "Evaluation Order":
The Java programming language guarantees that the operands of operators appear to be evaluated in a specific evaluation order, namely, from left to right.
§15.7.3, "Evaluation Respects Parentheses and Precedence" of the Java Language Specification adds
Java programming language implementations must respect the order of evaluation as indicated explicitly by parentheses and implicitly by operator precedence.
These two requirements can be counter-intuitive when expressions contain side effects. Evaluation of the operands proceeds left-to-right, without regard to operator precedence rules and indicative parentheses; evaluation of the operators, however, obeys precedence rules and parentheses.
Expressions must not write to memory that they subsequently read, and are also must not write to any memory twice. Note that memory writing and reading can occur either directly in the expression from assignments or indirectly through side effects in functions called in the expression.
Noncompliant Code Example (Order of Evaluation)
This noncompliant code example shows how side effects in expressions can lead to unanticipated outcomes. The programmer intends to write access control logic based on different threshold levels. Each user has a rating that must be above the threshold to be granted access. As shown, a simple function can calculate the rating. The get()
method is expected to return a non-zero factor for users who are authorized, and a zero value for those who are unauthorized.
In this case, the programmer expects the rightmost subexpression to evaluate first because the *
operator has a higher precedence than the +
operator. The parentheses reinforce this belief. These ideas lead to the incorrect conclusion that the right hand side evaluates to zero whenever the get()
method returns zero. The programmer expects number
to be assigned 0 because of the rightmost number = get()
subexpression. Consequently, the test in the left hand subexpression is expected to reject the unprivileged user because the rating value (number
) is below the threshold of 10
.
However, the program grants access to the unauthorized user because evaluation of the side-effect-infested subexpressions follows the left to right ordering rule.
class BadPrecedence { public static void main(String[] args) { int number = 17; int[] threshold = new int[20]; threshold[0] = 10; number = (number > threshold[0]? 0 : -2) + ((31 * ++number) * (number = get())); // ... if(number == 0) { System.out.println("Access granted"); } else { System.out.println("Denied access"); // number = -2 } } public static int get() { int number = 0; // Assign number to non zero value if authorized else 0 return number; } }
Noncompliant Code Example (Order of Evaluation)
This noncompliant code example reorders the previous expression so that the left-to-right evaluation order of the operands corresponds with the programmer's intent.
Although this code performs as expected, it still represents poor practice by writing to number
three times in a single expression.
int number = 17; number = ((31 * ++number) * (number=get())) + (number > threshold[0]? 0 : -2);
Compliant Solution (Order of Evaluation)
This compliant solution uses equivalent code with no side effects. It performs only one write, to number
. The resulting expression can be reordered without concern for the evaluation order of the component expressions, making the code easier to understand and maintain.
int number = 17; final int authnum = get(); number = ((31 * (number + 1)) * authnum) + (authnum > threshold[0]? 0 : -2);
Exceptions
EXP05-EX0: The postfix increment and postfix decrement operators (++
and --
) assign a new value to a variable and then subsequently read it. These are well-understood and are an exception to the rule against reading memory that was written in the same expression.
EXP05-EX1: The logical operators ||
and &&
have well-understood short-circuit semantics, so expressions involving these operators may violate this rule. Consider the following code:
public void exampleFunction(InputStream in) { int i; // Skip one char, process next while ((i = in.read()) != -1 && (i = in.read()) != -1) { // ... } }
Although the overall conditional expression violates this rule, this code is compliant because the sub-expressions on either side of the &&
operator do not violate it. Each has exactly one assignment and one side effect (the reading of a character from in
).
Risk Assessment
Failure to understand the evaluation order of expressions containing side effects can result in unexpected output.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP05-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
Detection of all expressions involving both side effects and also multiple operator precedence levels is straightforward. Determining the correctness of such uses is infeasible in the general case; heuristic warnings could be useful.
Related Guidelines
EXP30-C. Do not depend on order of evaluation between sequence points |
||||
EXP30-CPP. Do not depend on order of evaluation between sequence points |
||||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="033885b1-045e-4f2f-b533-ca47d922c14f"><ac:plain-text-body><![CDATA[ |
[ISO/IEC TR 24772:2010 |
http://www.aitcnet.org/isai/] |
"Side?effects and Order of Evaluation [SAM]" |
]]></ac:plain-text-body></ac:structured-macro> |
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="89914622-5f6c-42c2-a984-0f2815f6f66c"><ac:plain-text-body><![CDATA[ |
[[JLS 2005 |
AA. Bibliography#JLS 05]] |
[§15.7, "Evaluation Order" |
http://java.sun.com/docs/books/jls/third_edition/html/expressions.html#15.7] |
]]></ac:plain-text-body></ac:structured-macro> |
|
EXP04-J. Ensure that autoboxed values have the intended type 02. Expressions (EXP) EXP06-J. Do not use side-effecting expressions in assertions