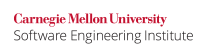
Many methods offer invariants, which can be any or all of guarantees made about what the method can do, requirements about the required state of the object when the method is invoked, or guarantees about the state of the object when the method completes. For instance, the %
operator, which computes the remainder of a number provides the invariant that
0 <= abs(a % b) <= abs(b), for all integers a, b where b != 0
Many classes also offer invariants, which are guarantees made about the state of their objects' fields upon the completion of all their methods. For instance, classes whose member fields may not be modified once they have assumed a value are called immutable classes. An important consequence of immutability is that the invariants of instances of these classes are preserved throughout their lifetimes.
A fundamental principle of object-oriented design is that a subclass which extends a superclass must preserve the invariants provided by the superclass. Unfortunately, design principles fail to constrain attackers, who can (and do!) construct malicious classes that extend benign classes and provide methods that deliberately violate the invariants of the benign classes.
For instance, an immutable class that lacks the final
qualifier can be extended by a malicious subclass that can modify the state of the supposedly-immutable object. Further, the malicious subclass can impersonate the immutable object while actually remaining mutable. Such malicious subclasses can then violate program invariants on which clients depend, consequently introducing security vulnerabilities.
As a result, classes with invariants on which other code depends should be declared final
, thereby preventing malicious subclasses from subverting their invariants. Furthermore, immutable classes must be declared final
.
Superclasses must permit extension by trusted subclasses while simultaneously preventing extension by untrusted code. Consequently, declaring such superclasses to be final
is infeasible, because it would prevent the required extension by trusted code. Such problems require careful design for inheritance.
Consider two classes belonging to different protection domains; one is malicious, and extends the other, which is trusted. Consider an object of the malicious subclass with a fully qualified invocation of a method defined by the trusted superclass, and not overridden by the malicious class. In this case, the trusted superclass's permissions are examined to execute the method, with the consequence that the malicious object gets the method invoked inside the protection domain of the trusted superclass [[Gong 2003]].
One commonly suggested solution is to place code at each point where the superclass can be instantiated to ensure that the instance being created has the same type as the superclass. When the type is found to be that of a subclass rather than the superclass's type, the checking code performs a security manager check to ensure that malicious classes cannot misuse the superclass. This approach is insecure because it allows a malicious class to add a finalizer and obtain a partially-initialized instance of the superclass. This attack is detailed in guideline OBJ05-J. Do not allow access to partially initialized objects.
For non-final classes, the method that performs the security manager check must be invoked as an argument to a private
constructor to ensure that the security check is performed before any superclass's constructor can exit.
When the parent class has members that are declared private
or are otherwise inaccessible to the attacker, the attacker must use reflection to exploit those members of the parent class. Declaring the parent class or its methods final
prohibits this level of access.
A method which receives an untrusted, non-final input argument must beware that other methods or threads might modify the input object. Some methods attempt to prevent modification by making a local copy of the input object. This is insufficient, because a shallow copy of an object may still allow it to refer to mutable sub-objects, which may still be modified by other methods or threads. Some methods go farther and perform a deep copy of the input object. This mitigates the problem of modifiable sub-objects, but the method might still receive as an argument a mutable object that extends the input object class.
Noncompliant Code Example (BigInteger
)
This noncompliant code example uses the immutable java.math.BigInteger
class.
class BigInteger { // ... }
The class is non-final and consequently extendable. This can be a problem when operating on an instance of BigInteger
that was obtained from an untrusted client. For example, a malicious client may construct a spurious mutable BigInteger
instance by overriding BigInteger
's member functions [[Bloch 2008]].
The following code example demonstrates such an attack.
BigInteger msg = new BigInteger("123"); msg = msg.modPow(exp, m); // Always returns 1 // Malicious subclassing of java.math.BigInteger class BigInteger extends java.math.BigInteger { private int value; public BigInteger(String str) { super(str); value = Integer.parseInt( str); } public setValue(int value) { this.value = value; } @Override public java.math.BigInteger modPow(java.math.BigInteger exponent, java.math.BigInteger m) { this.value = ((int) (Math.pow( this.value, exponent))) % m; return this; } }
This malicious BigInteger
class is clearly mutable, because of the setValue()
method. Furthermore, the modPow()
method is subject to precision loss (see NUM00-J. Detect or prevent integer overflow, NUM11-J. Check floating point inputs for exceptional values, NUM15-J. Ensure conversions of numeric types to narrower types do not result in lost or misinterpreted data and NUM17-J. Beware of precision loss when converting primitive integers to floating-point for more information). Any code that receives an object of this class, and assumes that the object is immutable will have unexpected behavior. This is particularly important because the BigInteger.modPow()
method has several useful cryptographic applications.
Noncompliant Code Example (Security Manager)
This noncompliant code example installs a security manager check in the constructor of the BigInteger
class. The security manager denies access when it detects that a subclass without the requisite permissions is attempting to instantiate the superclass [[SCG 2007]]. It also compares class types, in compliance with [OBJ06-J. Compare classes and not class names].
public class BigInteger { public BigInteger(String str) { Class c = getClass(); // java.lang.Object.getClass(), which is final // Confirm class type if (c != NonFinal.class) { // Check the permission needed to subclass NonFinal securityManagerCheck(); // throws a security exception if not allowed } // ... } }
Unfortunately, throwing an exception from the constructor of a non-final class is insecure because it allows a finalizer attack (see OBJ05-J. Do not allow access to partially initialized objects).
Compliant Solution (final
)
This compliant solution prevents creation of malicious subclasses by declaring the immutable BigInteger
class to be final
. Although this solution would be appropriate for locally maintained code, it cannot be used in the case of java.math.BigInteger
because it would require changing the Java SE API, which has already been published and must remain compatible with previous versions.
final class BigInteger { // ... }
Compliant Solution (Class Sanitization)
When instances of non-final classes are obtained from untrusted sources, such instances must be used with care, because their method calls might be overridden by malicious methods. This potential vulnerability can be mitigated by making defensive copies of the acquired instances prior to use. This compliant solution demonstrates this technique for a BigInteger
argument [[Bloch 2008]].
public static BigInteger safeInstance(BigInteger val) { // create a defensive copy if it is not java.math.BigInteger if (val.getClass() != java.math.BigInteger.class) return new BigInteger(val.toByteArray()); return val; }
The guidelines FIO00-J. Defensively copy mutable inputs and mutable internal components and OBJ10-J. Provide mutable classes with copy functionality to allow passing instances to untrusted code safely discuss defensive copying in great depth.
Compliant Solution (Java SE 6, public and private constructors)
This compliant solution invokes a security manager check as a side-effect of computing the boolean value passed to a private constructor (as seen in guideline OBJ05-J. Do not allow access to partially initialized objects). The rules for order of evaluation require that the security manager check must execute before invocation of the private constructor. Consequently, the security manager check also executes before invocation of any superclass's constructor. Note that the security manager check is made without regard to whether the object under construction has the type of the parent class or the type of a subclass (whether trusted or not).
This solution prevents the finalizer attack; it appiles to Java SE 6 and later versions, where throwing an exception before the java.lang.Object
constructor exits prevents execution of finalizers [[SCG 2009]].
public class BigInteger { public BigInteger(String str) { this( str, check( this.getClass())); // throws a security exception if not allowed } private BigInteger(String str, boolean securityManagerCheck) { // regular construction goes here } private static boolean check(Class c) { // Confirm class type if (c != BigInteger.class) { // Check the permission needed to subclass BigInteger securityManagerCheck(); // throws a security exception if not allowed } return true; } }
Risk Assessment
Permitting a non-final class or method to be inherited without checking the class instance allows a malicious subclass to misuse the privileges of the class.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ00-J |
medium |
likely |
medium |
P12 |
L1 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[API 2006]] Class BigInteger
[[Bloch 2008]] Item 1: "Consider static factory methods instead of constructors"
[[Gong 2003]] Chapter 6: "Enforcing Security Policy"
[[Lai 2008]]
[[McGraw 2000]] Chapter Seven Rule 3: "Make Everything Final, Unless There's a Good Reason Not To"[[SCG 2007]] Guideline 1-2 "Limit the extensibility of classes and methods"
[[SCG 2009]]
04. Object Orientation (OBJ) 04. Object Orientation (OBJ) OBJ01-J. Declare data members as private and provide accessible wrapper methods