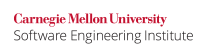
The synchronized
keyword is used to acquire a mutual-exclusion lock so that no other thread can acquire the lock, while it is being held by the executing thread. Recall that there are two ways to synchronize access to shared mutable variables, method synchronization and block synchronization. Excessive method synchronization can induce a denial of service (DoS) vulnerability because another class whose member locks on the class object, can fail to release the lock promptly. However, this requires the victim class to be accessible from the hostile class.
The private lock object idiom can be used to prevent the DoS vulnerability. The idiom consists of a private
object declared as an instance field. The private
object must be explicitly used for locking purposes in synchronized
blocks, within the class's methods. This lock object belongs to an instance of the object and is not associated with the object itself. Consequently, there is no lock contention between a class method and a method of the hostile class when both try to lock on the class object. [[Bloch 01]]
This idiom can also be suitably used by classes designed for inheritance. If a superclass thread requests a lock on the class object's monitor, a subclass thread can interfere with its operation. Refer to the guideline CON01-J. Always synchronize on the appropriate object for more details.
Noncompliant Code Example
This noncompliant code example exposes the class object someObject
to untrusted code. The untrusted code attempts to acquire a lock on the class object's monitor and upon succeeding, introduces an indefinite delay which holds up the synchronized
changeValue()
method from acquiring the same lock. Note that the untrusted code also violates CON07-J. Do not defer a thread that is holding a lock.
public class SomeObj { public synchronized void changeValue() { // Locks on the class object's monitor // ... } } // Untrusted code synchronized (someObject) { while(true) { Thread.sleep(Integer.MAX_VALUE); // Indefinitely delay someObject } }
Compliant Solution
Thread-safe classes that use intrinsic synchronization may be protected by using the private lock object idiom and adapting them to use block synchronization. In this compliant solution, if the method changeValue()
is called, the lock is obtained on a private
Object
that is both invisible and inaccessible from the caller.
public class SomeObj { private final Object lock = new Object(); // private lock object public void changeValue() { synchronized(lock) { // Locks on the private Object // ... } } }
For more details on using the private
Object lock refer to CON01-J. Always synchronize on the appropriate object.
Risk Assessment
Exposing the class object to untrusted code can result in denial-of-service.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON10- J |
low |
probable |
medium |
P4 |
L3 |
References
[[Bloch 01]] Item 52: "Document Thread Safety"
CON04-J. Ensure that threads do not fail during activation 11. Concurrency (CON) CON00-J. Synchronize access to shared mutable variables