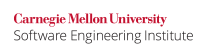
According to the Java Language Specification [[JLS 05]] section 15.7 "Evaluation Order":
The Java programming language guarantees that the operands of operators appear to be evaluated in a specific evaluation order, namely, from left to right.
Section 15.7.3 "Evaluation Respects Parentheses and Precedence", on the other hand states:
Java programming language implementations must respect the order of evaluation as indicated explicitly by parentheses and implicitly by operator precedence.
These two requirements can be counter intuitive when expressions contain side-effects. This is because such expressions follow the left to right evaluation order irrespective of operator precedence, associativity rules and indicative parentheses. The best practice is to avoid using expressions containing multiple side-effects. When used, the expressions must be carefully structured to respect the left to right evaluation order.
Noncompliant Code Example
This noncompliant code example shows how side-effects in expressions can lead to unanticipated outcomes. The programmer intends to write access control logic based on different threshold levels. Each user has a rating that must be above the threshold to be granted access. As shown, a simple function can be used to calculate the rating. The get()
method is expected to return a non-zero factor when the user is authorized, and a zero value when not.
In this case, the programmer expects the rightmost subexpression to evaluate first because the *
operator has a higher precedence than the +
operator. The parentheses reinforce this belief. These ideas lead to the incorrect conclusion that the right hand side evaluates to zero whenever the get()
method returns zero. The programmer expects number
to be assigned 0 because of the rightmost number = get()
subexpression. Consequently, the test in the left hand subexpression is expected to reject the unprivileged user because the rating value (number
) is below the threshold of 10
.
However, the program grants access to the unauthorized user. The reason is that evaluation of the side-effect infested subexpressions follows the left to right ordering rule which should not be confused with the tenets of operator precedence, associativity and indicative parentheses.
class BadPrecedence { public static void main(String[] args) { int number = 17; int[] threshold = new int[20]; threshold[0] = 10; number = (number > threshold[0]? 0 : -2) + ((31 * ++number) * (number = get())); // ... if(number == 0) { System.out.println("Access granted"); } else { System.out.println("Denied access"); // number = -2 } } public static int get() { int number = 0; // Assign number to non zero value if authorized else 0 return number; } }
Compliant Solution
By diligently following the left to right evaluation order, a programmer can expect this compliant solution to evaluate to the expected final outcome depending on the value returned by the get()
method.
number = ((31 * ++number) * (number=get())) + (number > threshold[0]? 0 : -2);
Although this solution solves the problem, in general it is advisable to avoid using expressions with more than one side-effect. It is also inadvisable to depend on the left-right ordering for evaluation of side-effects because operands are evaluated in place first, and then subject to laws of operator precedence and associativity.
Compliant Solution
This compliant solution uses an equivalent expression with no side-effects. This allows the expression to be reordered without concern for the evaluation order of the component expressions, making the code easier to understand and maintain.
number = ((31 * (number + 1)) * get()) + (get() > threshold[0]? 0 : -2);
Risk Assessment
Failing to keep in mind the evaluation order of expressions containing side effects can result in unexpected output.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP30- J |
low |
unlikely |
medium |
P2 |
L3 |
Other Languages
This rule appears in the C Coding Standard as EXP30-C. Do not depend on order of evaluation between sequence points.
This rule appears in the C++ Coding Standard as EXP30-CPP. Do not depend on order of evaluation between sequence points.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Section 15.7 "Evaluation Order" and 15.7.3 "Evaluation Respects Parentheses and Precedence"
EXP10-J. Understand the evaluation of expressions containing non short-circuit operators 04. Expressions (EXP) EXP31-J. Avoid side effects in assertions