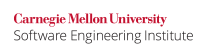
If a program relies on finalize()
to release system resources, or if there is confusion over which part of the program is responsible for releasing system resources, then there exists a possibility for a potential resource leak. In a busy system, there might be a time gap before the finalize()
method is called for an object. An attacker might exploit this vulnerability to induce a denial-of-service attack. The recommendation OBJ02-J. Avoid using finalizers has more information on the demerits of using finalizers.
The Java garbage collector is called to free up unreleased memory. However, if the program relies on nonmemory resources like file descriptors and database connections, unreleased resources might lead the program to prematurely exhaust its pool of resources. In addition, if the program uses resources like Lock
or Semaphore
, waiting for finalize()
to release the resources may lead to resource starvation. Caching of object references in the output stream also implies that the objects will not be garbage collected unless the streams are closed promptly after use.
Noncompliant Code Example
This problem is aggravated in the case of database connections. Traditionally, database servers allow a fixed number of connections, which may be dependent on configuration or licensing issues. Not releasing such connections could lead to rapid exhaustion of available connections.
public void getResults(String sqlQuery) { try { Connection conn = getConnection(); Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery(sqlQuery); processResults(rs); stmt.close(); } catch (SQLException e) { } }
In the case above, if an error occurs while executing the statement or while processing the results of the statement, the connection is not closed. A finally
block can be used to ensure that the close
statements are eventually called.
Noncompliant Code Example
However, while being slightly better, this code is also noncompliant. Both rs
and stmt
might be null.
Statement stmt = null; ResultSet rs = null Connection conn = getConnection(0; try { stmt = conn.createStatement(); rs = stmt.executeQuery(sqlQuery); processResults(rs); } catch(SQLException e) { } finally { rs.close(); stmt.close(); } }
Noncompliant Code Example
Again, while being still better, the code is still noncompliant. This is because rs.close()
might itself result in a SQLException
, and so stmt.close()
will never be called.
Statement stmt = null; ResultSet rs = null; Connection conn = getConnection(); try { stmt = conn.createStatement(); rs = stmt.executeQuery(sqlQuery); processResults(rs); } catch(SQLException e) { } finally { if(rs != null) { rs.close(); } if(stmt != null) { stmt.close(); } } }
Compliant Solution
This compliant solution shows how to ensure that resources have been released.
Statement stmt = null; ResultSet rs = null; Connection conn = getConnection(); try { stmt = conn.createStatement(); rs = stmt.executeQuery(sqlQuery); processResults(rs); } catch(SQLException e) { } finally { try { if(rs != null) { rs.close(); } } finally ( try { if(stmt != null) { stmt.close(); } } finally { conn.close(); } } }
Noncompliant Code Example
The worst form of noncompliance is not calling methods to release the resource at all. If files are opened, they must be explicitly closed when their work is done.
public int processFile(String fileName) throws IOException, FileNotFoundException { FileInputStream stream = new FileInputStream(fileName); BufferedReader bufRead = new BufferedReader(new InputStreamReader(stream)); String line; while((line=bufRead.readLine()) != null) { sendLine(line); } return 1; }
Compliant Code Example
This compliant code example would release all acquired resources, regardless of any exceptions which might occur. Hence, in the compliant code below, even though bufRead
might result in an exception, if a FileInputStream
object was instantiated, it will be closed.
FileInputStream stream = null; BufferedReader bufRead = null; String line; try { stream = new FileInputStream(fileName); bufRead = new BufferedReader(new InputStreamReader(stream)); while((line=bufRead.readLine()) != null) { sendLine(line); } } catch (IOException e) { } finally { stream.close(); }
Risk Assessment
Acquiring nonmemory system resources and not releasing them explicitly might lead to resource exhaustion.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO34-J |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as FIO42-C. Ensure files are properly closed when they are no longer needed.
This rule appears in the C++ Secure Coding Standard as FIO42-CPP. Ensure files are properly closed when they are no longer needed.
References
[[API 06]] Class Object
[[Goetz 06b]]
[[MITRE 09]] CWE ID 405 "Asymmetric Resource Consumption (Amplification)", CWE ID 404
"Improper Resource Shutdown or Release"
FIO33-J. Do not allow serialization and deserialization to bypass the Security Manager 07. Input Output (FIO) FIO35-J. Exclude user input from format strings