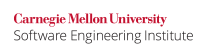
The write()
method that is defined in java.io.OutputStream
, takes an integer argument intended to be between 0 and 255. Because an int
is otherwise designed to store 4 byte numbers, failure to validate user input may lead to unexpected results.
The general contract for write is that one byte is written to the output stream. The byte to be written is the eight low-order bits of the argument b. The 24 high-order bits of b are ignored. [[API 06]]
Noncompliant Code Example
The noncompliant code example accepts a value from the user without validating it. If this value is greater than 255, it will result in a wrap around. For instance, write(305) will print '1' since the lower order bits of 305 are preserved while the top 24 order bits are lost. That is, the result is remainder modulo 256 of the absolute value of the input.
class ConsoleWrite { public static void main(String[] args) { //Any input value > 255 will result in unexpected output System.out.write(Integer.valueOf(args[0])); System.out.flush(); } }
Compliant Solution
Use alternative means to output integers such as the System.out.print*
methods.
class ConsoleWrite { public static void main(String[] args) { System.out.println(args[0]); } }
Compliant Solution 2
Alternatively, perform input validation.
class ConsoleWrite { public static void main(String[] args) { //Perform input validation if(Integer.valueOf(args[0]) <= 255) { System.out.write(Integer.valueOf(args[0])); System.out.flush(); } else { //handle error throw new ArithmeticException("Value is out of range"); } } }
Risk Assessment
Using the write()
method to output integers may result in unexpected values.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT31-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] method write()
[[Harold 99]]
INT30-J. Be careful while casting integers to narrower types 04. Integers (INT) INT32-J. Perform conversion from BigInteger to String and back properly