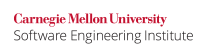
Autoboxing can automatically wrap the primitive type to the corresponding wrapper object, which can be convenient in many cases and avoid clutters in your own code. But you should always be careful about this process, especially when comparison. Consider the following code:
Noncompliant code Example
public class TestWrapper2 { Â public static void main(String[] args) { Â Â Â Â Integer i1 = 100; Â Â Â Â Integer i2 = 100; Â Â Â Â Integer i3 = 1000; Â Â Â Â Integer i4 = 1000; Â Â Â Â System.out.println(i1==i2); Â Â Â Â System.out.println(i1!=i2); Â Â Â Â System.out.println(i3==i4); Â Â Â Â System.out.println(i3!=i4); Â Â Â Â Â } }
Output of this code
true false false true
It is because that in JDK 5.0, if the value p being boxed is true, false, a byte, an ASCII character, or an integer or short number between -128 and 127, then let r1 and r2 be the results of any two boxing conversions of p. It is always the case that r1 == r2. In case of that, when we need to do some comparison of these wrapper class, we should use equal instead "==" (see EXP03-J for details):
Compliant solution
public class TestWrapper2 { Â public static void main(String[] args) { Â Â Â Â Integer i1 = 100; Â Â Â Â Integer i2 = 100; Â Â Â Â Integer i3 = 1000; Â Â Â Â Integer i4 = 1000; Â Â Â Â System.out.println(i1.equals(i2)); Â Â Â Â System.out.println(i3.equals(i4));Â Â Â Â Â } }Â
"Ideally, boxing a given primitive value p, would always yield an identical reference. In practice, this may not be feasible using existing implementation techniques. The rules above are a pragmatic compromise. The final clause above requires that certain common values always be boxed into indistinguishable objects. The implementation may cache these, lazily or eagerly."(From section 5.1.7 of JLS 3rd Ed_)_
To convince our idea, we can take an insight of the source code of Integer of JDK 1.6.0_10 from java SE:
 private static class IntegerCache {  private IntegerCache(){}  static final Integer cache[] = new Integer[-(-128) + 127 + 1];  static {     for(int i = 0; i < cache.length; i++)   cache[i] = new Integer(i - 128);  }    }
Here there exists a cache in the Integer, which clearly explains the result of above code. It also means that if we have enough memory, we could caches all the integer value(-32K-32K), which means that all the int value could be autoboxing to the same Integer object. However, actually it is impractical, so we should be careful about using the following code.
Noncompliant Code Example
In many times, you may want to create a dynamic array of integers. Unfortunately, the type parameter inside the angle brackets cannot be a primitive type. It is not possible to form an ArrayList<int>. Thanks to the wrapper class, now you can use ArrayList<Integer> to achieve this goal.
import java.util.ArrayList; public class TestWrapper1 {  public static void main(String[] args) {   //create an array list of integers, which each element   //is more than 127     ArrayList<Integer> list1 = new ArrayList<Integer>();     for(int i=0;i<10;i++)      list1.add(i+1000);   //create another array list of integers, which each element   //is the same with the first one     ArrayList<Integer> list2 = new ArrayList<Integer>();     for(int i=0;i<10;i++)      list2.add(i+1000);                int counter = 0;     for(int i=0;i<10;i++)      if(list1.get(i) == list2.get(i)) counter++;     //output the total equal number     System.out.println(counter);  } }Â
In JDK 1.6.0_10, the output of this code is 0. In this code, we want to count the same numbers of array list1 and array list2. Undoubtedly, the result is not the same as our expectation. But if we can set more caches inside Integer, then the result may be different!
Compliant solution
public class TestWrapper1 {  public static void main(String[] args) {   //create an array list of integers, which each element   //is more than 127     ArrayList<Integer> list1 = new ArrayList<Integer>();     for(int i=0;i<10;i++)      list1.add(i+1000);   //create another array list of integers, which each element   //is the same with the first one     ArrayList<Integer> list2 = new ArrayList<Integer>();     for(int i=0;i<10;i++)      list2.add(i+1000);                int counter = 0;     for(int i=0;i<10;i++)      if(list1.get(i).equals(list2.get(i))) counter++;     //output the total equal number     System.out.println(counter);  } }
In JDK 1.6.0_10, the output of this code is 10.
Risk Assessment
We often use array list with primitive type, so it will exert a potential security risk.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP05-J |
medium |
likely |
low |
P9 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Chapter 5, Core Java⢠2 Volume I - Fundamentals, Seventh Edition by Cay S. Horstmann, Gary Cornell
Publisher:Prentice Hall PTR;Pub Date:August 17, 2004.
Section 5.1.7, The Java⢠Language Specification,Third Edition by James Gosling, Bill Joy, Guy Steele, Gilad Bracha
Publisher:ADDISON-WESLEY;Pub Date:May 2005.