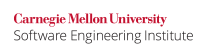
Tasks that depend on other tasks should not be executed in the same bounded thread pool. A bounded thread pool is one that has a fixed size. A task that submits another task to a single threaded Executor
remains blocked until the results are received whereas the second task may have dependencies on the first task. This constitutes a deadlock. Another form of deadlock called thread starvation deadlock can arise when a task spawns several other tasks and waits for them to complete. Because of the limited thread pool size, only a fixed number of tasks can execute to completion at a particular time. In this case, neither task can finish executing when there are no available slots on the thread pool's work queue to accommodate the sub-tasks.
Noncompliant Code Example
This noncompliant code example suffers from a thread starvation deadlock issue. It consists of class ValidationService
that performs various input validation tasks such as checking whether a user-supplied field exists in a back-end database. The fieldAggregator()
method accepts a variable number of String
arguments and creates a task corresponding to each argument to gain some speedup. The task performs input validation using class ValidateInput
. The class ValidateInput
in turn, attempts to sanitize the input by creating a sub-task for each request using class SanitizeInput
. All tasks are executed in the same thread pool. The method fieldAggregator()
blocks until all the tasks have finished executing. When all results are available, it aggregates the processed inputs as a StringBuilder
that is returned to its caller.
final class ValidationService { private final ExecutorService pool; public ValidationService(int poolSize) { pool = Executors.newFixedThreadPool(poolSize); } public void shutdown() { pool.shutdown(); } public StringBuilder fieldAggregator(String... inputs) throws InterruptedException, ExecutionException { StringBuilder sb = new StringBuilder(); Future<String>[] results = new Future[inputs.length]; // Stores the results for(int i = 0; i < inputs.length; i++) { // Submits the tasks to thread pool results[i] = pool.submit(new ValidateInput<String>(inputs[i], pool)); } for(int i = 0; i < inputs.length; i++) { // Aggregates the results sb.append(results[i].get()); } return sb; } } public final class ValidateInput<V> implements Callable<V> { private final String input; private final ExecutorService pool; ValidateInput(String input, ExecutorService pool) { this.input = input; this.pool = pool; } @Override public V call() throws Exception { // If validation fails, throw an exception here Future<String> future = pool.submit(new SanitizeInput<String>(input)); // Sub-task return (V)future.get(); } } public final class SanitizeInput<V> implements Callable<V> { private final String input; SanitizeInput(String input) { this.input = input; } @Override public V call() throws Exception { // Sanitize input and return return (V)input; } }
// Hidden main() method
public static void main(String[] args) throws InterruptedException, ExecutionException {
ValidationService vs = new ValidationService(5);
System.out.println(vs.fieldAggregator("field1", "field2","field3","field4", "field5","field6"));
vs.shutdown();
}
Assume that the caller sets the thread pool size as 6. When it calls ValidationService.fieldAggregator()
with six arguments that are required to be validated, six tasks are submitted to the thread pool. Six more sub-tasks corresponding to SanitizeInput
must also execute before these threads can return their results. However, this is not possible because the queue is full with all threads blocked. This issue is deceptive because the program may appear to function correctly when fewer arguments are supplied. Choosing a bigger pool size appears to solve the problem, however there is no easy way to determine a suitable size.
This situation can also occur when using single threaded Executors, for example, when the caller creates several sub-tasks and waits for the results. A thread starvation deadlock arises when all the threads executing in the pool are blocked on tasks that are waiting on the queue. A blocking operation within a sub-task can also lead to unbounded queue growth. [[Goetz 06]]
Compliant Solution
This compliant solution refactors all three classes so that the tasks corresponding to SanitizeInput
are not executed in a thread pool. Consequently, the tasks are independent of each other. An alternative is to use a different thread pool at each level, though in this example, another thread pool is unnecessary.
class ValidationService { // ... public StringBuilder fieldAggregator(String... inputs) throws InterruptedException, ExecutionException { // ... for (int i = 0; i < inputs.length; i++) { results[i] = pool.submit(new ValidateInput<String>(inputs[i])); // Don't pass-in thread pool } // ... } } public final class ValidateInput<V> implements Callable<V> { // Does not use same thread pool private final String input; ValidateInput(String input) { this.input = input; } @Override public V call() throws Exception { // If validation fails, throw an exception here return (V)SanitizeInput.sanitizeString(input); } } public final class SanitizeInput { // No longer a Callable task private SanitizeInput() { } public static String sanitizeString(String input) { // Sanitize input and return return input; } }
Always submit independent tasks to the Executor
. Thread starvation issues can be mitigated by choosing a large pool size, however, the limitations of the application when using this approach should be clearly documented.
Note that operations that have further constraints, such as the total number of database connections or total ResultSets
open at a particular time, impose an upper bound on the thread pool size as each thread continues to block until the resource becomes available. The other rules of fair concurrency, such as not running time consuming tasks, also apply. When this is not possible, expecting to obtain real time result guarantees from the execution of tasks is conceivably, an unreasonable target.
Sometimes, a private static
ThreadLocal
variable is used per thread to maintain local state. When using thread pools, ThreadLocal
variables should be used only if their lifetime is shorter than that of the corresponding task [[Goetz 06]]. Moreover, such variables should not be used as a communication mechanism between tasks.
Noncompliant Code Example
This noncompliant code example (based on [[Gafter 06]]) shows a BrowserManager
class that has several methods that use a fork-join mechanism, that is, they start threads and wait for them to finish. The methods are called in the sequence perUser()
, perProfile
and perTab()
. The method methodInvoker()
spawns several instances of the specified runnable depending on the value of the variable numberOfTimes
. A fixed sized thread pool is used to execute the enumerations of tasks created at different levels.
public final class BrowserManager { private final ExecutorService pool = Executors.newFixedThreadPool(10); private final int numberOfTimes; private static volatile int count = 0; public BrowserManager(int n) { this.numberOfTimes = n; } public void perUser() { methodInvoker(numberOfTimes, "perProfile"); pool.shutdown(); } public void perProfile() { methodInvoker(numberOfTimes, "perTab"); } public void perTab() { methodInvoker(numberOfTimes, "doSomething"); } public void doSomething() { System.out.println(++count); } public void methodInvoker(int n, final String method) { final BrowserManager fm = this; Runnable run = new Runnable() { public void run() { try { Method meth = fm.getClass().getMethod(method); meth.invoke(fm); } catch (Throwable t) { // Forward to exception reporter } } }; Collection<Callable<Object>> collection = new ArrayList<Callable<Object>>(); for(int i = 0; i < n; i++) { collection.add(Executors.callable(run)); } Collection<Future<Object>> futures = null; try { futures = pool.invokeAll(collection); } catch (InterruptedException e) { // Forward to handler Thread.currentThread().interrupt(); // Reset interrupted status } // ... } public static void main(String[] args) { BrowserManager manager = new BrowserManager(5); manager.perUser(); } }
Contrary to what is expected, this program does not print the total count, that is, the number of times doSomething()
is invoked. This is because it is susceptible to a thread starvation deadlock because the size of the thread pool (10) does not allow either thread from perTab()
to invoke the doSomething()
method. The output of the program varies for different values of numberOfTimes
and the thread pool size. Note that different threads are allowed to invoke doSomething()
in different orders; we are concerned only with the maximum value of count
to determine how many times the method executed.
Compliant Solution
This compliant solution selects and schedules tasks for execution, and consequently, avoids the thread starvation deadlock. Every level (worker) must have a double ended queue where all sub-tasks are queued [[Goetz 06]]. Each level removes the most recently generated sub-task from the queue so that it can process it. When there are no more threads left to process, the current level runs the least-recently created sub-task of another level by picking and removing it from that level's queue (work stealing).
This compliant solution sets the CallerRuns
policy on a ThreadPoolExecutor
, and uses a synchronous queue [[Gafter 06]].
public final class BrowserManager { private final static ThreadPoolExecutor pool = new ThreadPoolExecutor(0, 10, 60L, TimeUnit.SECONDS, new SynchronousQueue<Runnable>()); private final int numberOfTimes; private static volatile int count = 0; static { pool.setRejectedExecutionHandler( new ThreadPoolExecutor.CallerRunsPolicy()); } // ... }
According to the Java API [[API 06]], class java.util.concurrent.ThreadPoolExecutor.CallerRunsPolicy
documentation:
A handler for rejected tasks that runs the rejected task directly in the calling thread of the
execute
method, unless the executor has been shut down, in which case the task is discarded.
The caller runs policy allows graceful degradation of service when faced with many requests by distributing the workload across the work queue, the underlying abstraction (such as the queue of a TCP/IP connection) and the caller.
This compliant solution is subject to the vagaries of the thread scheduler which may not optimally schedule the tasks, however, it avoids the thread starvation deadlock.
Risk Assessment
Executing interdependent tasks in a thread pool can lead to denial of service.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON29- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[Gafter 06]] A Thread Pool Puzzler
[[Goetz 06]] 5.3.3 Deques and work stealing
[!The CERT Sun Microsystems Secure Coding Standard for Java^button_arrow_left.png!] [!The CERT Sun Microsystems Secure Coding Standard for Java^button_arrow_up.png!] [!The CERT Sun Microsystems Secure Coding Standard for Java^button_arrow_right.png!]