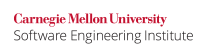
Many methods offer invariants, which can be any or all of the guarantees made about what the method can do, requirements about the required state of the object when the method is invoked, or guarantees about the state of the object when the method completes. For instance, the %
operator, which computes the remainder of a number, provides the invariant that
0 <= abs(a % b) < abs(b), for all integers a, b where b != 0
Many classes also offer invariants, which are guarantees made about the state of their objects' fields upon the completion of any of their methods. For instance, classes whose member fields may not be modified once they have assumed a value are called immutable classes. An important consequence of immutability is that the invariants of instances of these classes are preserved throughout their lifetimes.
A fundamental principle of object-oriented design is that a subclass that extends a superclass must preserve the invariants provided by the superclass. Unfortunately, design principles fail to constrain attackers, who can (and do) construct malicious classes that extend benign classes and provide methods that deliberately violate the invariants of the benign classes.
For instance, an immutable class that lacks the final qualifier can be extended by a malicious subclass that can modify the state of the supposedly immutable object. Furthermore, a malicious subclass object can impersonate the immutable object while actually remaining mutable. Such malicious subclasses can violate program invariants on which clients depend, consequently introducing security vulnerabilities.
To prevent misuse, classes with invariants on which other code depends should be declared final. Furthermore, immutable classes must be declared final.
Some superclasses must permit extension by trusted subclasses while simultaneously preventing extension by untrusted code. Declaring such superclasses to be final is infeasible because it would prevent the required extension by trusted code. Such problems require careful design for inheritance.
Consider two classes belonging to different protection domains: one is malicious and extends the other, which is trusted. Consider an object of the malicious subclass with a fully qualified invocation of a method defined by the trusted superclass, not overridden by the malicious class. In this case, the trusted superclass's permissions are examined to execute the method, and, as a result, the malicious object gets the method invoked inside the protection domain of the trusted superclass [Gong 2003].
One commonly suggested solution is to place code at each point where the superclass can be instantiated to ensure that the instance being created has the same type as the superclass. When the type is found to be that of a subclass rather than the superclass's type, the checking code performs a security manager check to ensure that malicious classes cannot misuse the superclass. This approach is insecure because it allows a malicious class to add a finalizer and obtain a partially initialized instance of the superclass. This attack is detailed in rule OBJ11-J. Be wary of letting constructors throw exceptions.
For non-final classes, the method that performs the security manager check must be invoked as an argument to a private constructor to ensure that the security check is performed before any superclass's constructor can exit. For an example of this technique, see rule OBJ11-J. Be wary of letting constructors throw exceptions.
A method that receives an untrusted, nonfinal input argument must beware that other methods or threads might concurrently modify the input object. Some methods attempt to prevent modification by making a local copy of the input object. This is insufficient because a shallow copy of an object can still allow it to refer to mutable subobjects, that can be modified by other methods or threads. Some methods go further and perform a deep copy of the input object. Although this mitigates the problem of modifiable subobjects, the method could still receive as an argument a mutable object that extends the input object class and provides inadequate copy functionality.
Noncompliant Code Example (BigInteger
)
This noncompliant code example extends the java.math.BigInteger
class. This class is non-final and consequently extendable. This can be a problem when operating on an instance of BigInteger
that was obtained from an untrusted client. For example, a malicious client could construct a spurious mutable BigInteger
instance by overriding BigInteger
's member functions [Bloch 2008].
The following code example demonstrates such an attack.
BigInteger msg = new BigInteger("123"); msg = msg.modPow(exp, m); // Always returns 1 // Malicious subclassing of java.math.BigInteger class BigInteger extends java.math.BigInteger { private int value; public BigInteger(String str) { super(str); value = Integer.parseInt(str); } public void setValue(int value) { this.value = value; } @Override public java.math.BigInteger modPow( java.math.BigInteger exponent, java.math.BigInteger m) { this.value = ((int) (Math.pow(this.doubleValue(), exponent.doubleValue()))) % m.intValue(); return this; } }
Unlike the benign BigInteger
class, this malicious BigInteger
class is clearly mutable because of the setValue()
method. Furthermore, the malicious modPow()
method (which overrides a benign modPow()
method) is subject to precision loss. (See rules NUM00-J. Detect or prevent integer overflow, NUM08-J. Check floating-point inputs for exceptional values, NUM12-J. Ensure conversions of numeric types to narrower types do not result in lost or misinterpreted data, and NUM13-J. Avoid loss of precision when converting primitive integers to floating-point for more information.) Any code that receives an object of this class and assumes that the object is immutable will behave unexpectedly. This is particularly important because the BigInteger.modPow()
method has several useful cryptographic applications.
Noncompliant Code Example (Security Manager)
This noncompliant code example installs a security manager check in the constructor of the BigInteger
class. The security manager denies access when it detects that a subclass without the requisite permissions is attempting to instantiate the superclass [SCG 2009]. It also compares class types, in compliance with rule OBJ09-J. Compare classes and not class names. Note that this check does not prevent malicious extensions of BigInteger
, it instead prevents the creation of BigInteger
objects from untrusted code, which also prevents creation of objects of malicious extensions of BigInteger
.
public class BigInteger { public BigInteger(String str) { securityManagerCheck(); // ... } // Check the permission needed to subclass BigInteger // throws a security exception if not allowed private void securityManagerCheck() { // ... } }
Unfortunately, throwing an exception from the constructor of a non-final class is insecure because it allows a finalizer attack. (See rule OBJ11-J. Be wary of letting constructors throw exceptions.)
Compliant Solution (Final)
This compliant solution prevents creation of malicious subclasses by declaring the immutable BigInteger
class to be final. Although this solution would be appropriate for locally maintained code, it cannot be used in the case of java.math.BigInteger
because it would require changing the Java SE API, which has already been published and must remain compatible with previous versions.
final class BigInteger { // ... }
Compliant Solution (Class Sanitization)
The instances of nonfinal classes obtained from untrusted sources must be used with care because their methods might be overridden by malicious methods. This potential vulnerability can be mitigated by making defensive copies of the acquired instances prior to use. This compliant solution demonstrates this technique for a BigInteger
argument [Bloch 2008].
public static BigInteger safeInstance(BigInteger val) { // create a defensive copy if it is not java.math.BigInteger if (val.getClass() != java.math.BigInteger.class) { return new BigInteger(val.toByteArray()); } return val; }
Rules OBJ06-J. Defensively copy mutable inputs and mutable internal components and OBJ04-J. Provide mutable classes with copy functionality to safely allow passing instances to untrusted code discuss defensive copying in great depth.
Compliant Solution (Java SE 6, Public and Private Constructors)
This compliant solution invokes a security manager check as a side effect of computing the Boolean value passed to a private constructor (as seen in rule OBJ11-J. Be wary of letting constructors throw exceptions). The rules for order of evaluation require that the security manager check must execute before invocation of the private constructor. Consequently, the security manager check also executes before invocation of any superclass's constructor. Note that the security manager check is made without regard to whether the object under construction has the type of the parent class or the type of a subclass (whether trusted or not).
This solution prevents the finalizer attack; it applies to Java SE 6 and later versions, where throwing an exception before the java.lang.Object
constructor exits prevents execution of finalizers [SCG 2009].
public class BigInteger { public BigInteger(String str) { this(str, check()); } private BigInteger(String str, boolean dummy) { // regular construction goes here } private static boolean check() { securityManagerCheck(); return true; } }
Noncompliant Code Example (Data-Driven Execution)
Code in privileged blocks should be as simple as possible, both to improve reliability and to simplify security audits. Invocation of overridable methods permits modification of the code that is executed in the privileged context without modification of previously audited classes. Furthermore, calling overridable methods disperses the code over multiple classes, making it harder to determine which code must be audited. Malicious subclasses cannot directly exploit this issue because privileges are dropped as soon as unprivileged code is executed. Nevertheless, maintainers of the subclasses might unintentionally violate the requirements of the base class. For example, even when the base class's overridable method is thread-safe, a subclass might provide an implementation that lacks this property, leading to security vulnerabilities.
This noncompliant code example invokes an overridable getMethodName()
method in the privileged block using the reflection mechanism.
public class MethodInvoker { public void invokeMethod() { AccessController.doPrivileged(new PrivilegedAction<Object>() { public Object run() { try { Class<?> thisClass = MethodInvoker.class; String methodName = getMethodName(); Method method = thisClass.getMethod(methodName, null); method.invoke(new MethodInvoker(), null); } catch (Throwable t) { // Forward to handler } return null; } } ); } String getMethodName() { return "someMethod"; } public void someMethod() { // ... } // Other methods }
A subclass can override getMethodName()
to return a string other than "someMethod"
. If an object of such a subclass runs invokeMethod()
, control flow will divert to a method other than someMethod()
.
Compliant Solution (Final)
This compliant solution declares the getMethodName()
method final so that it cannot be overridden.
final String getMethodName() { // ... }
Alternative approaches that prevent overriding of the getMethodName()
method include declaring it as private or declaring the enclosing class as final.
Risk Assessment
Permitting a nonfinal class or method to be inherited without checking the class instance allows a malicious subclass to misuse the privileges of the class.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
OBJ00-J | medium | likely | medium | P12 | L1 |
Related Guidelines
Secure Coding Guidelines for the Java Programming Language, Version 3.0 | Guideline 1-2. Limit the extensibility of classes and methods |
Bibliography
[API 2006] | Class BigInteger |
Item 1. Consider static factory methods instead of constructors | |
Chapter 6, Enforcing Security Policy | |
[Lai 2008] | Java Insecurity, Accounting for Subtleties That Can Compromise Code |
Chapter Seven, Rule 3. Make everything final, unless there's a good reason not to | |
04. Object Orientation (OBJ) 04. Object Orientation (OBJ) OBJ01-J. Declare data members as private and provide accessible wrapper methods