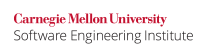
A Java OutofMemoryError
occurs if the program attempts to use more heap space than is available, even after garbage collection. Amongst others, this error can result from:
- A memory leak
- An infinite loop
- Limited amounts of default heap memory available
- Incorrect implementation of common data structures (hash tables, vectors and so on)
- Unbound deserialization
- Upon writing a large number of objects to an
ObjectOutputStream
Noncompliant Code Example
This noncompliant code example places no upper bounds on the memory space required to execute the program. Consequently, the program can easily exhaust the heap.
public class ShowHeapError { Vector<String> names = new Vector<String>(); InputStreamReader input = new InputStreamReader(System.in); BufferedReader reader = new BufferedReader(input); public void addNames() throws IOException { while(true) { // Adding unknown number of records to a list; user can exhaust the heap String newName = reader.readLine(); if(!newName.equalsIgnoreCase("quit")) { // Enter "quit" to quit the program names.addElement(newName); } else { break; } } // Close "reader" and "input" } public static void main(String[] args) throws IOException { ShowHeapError demo = new ShowHeapError(); demo.addNames(); } }
Compliant Solution
If the objects or data structures are large enough to potentially cause heap exhaustion, the programmer must consider using databases instead.
To remedy the noncompliant code example, the user can reuse a single long
variable to store the input and write that value into a database containing a table User
, with a field userID
along with any other required fields. This prevents the heap from getting exhausted.
Noncompliant Code Example
This noncompliant code example requires more memory on the heap than is available by default. In a server-class machine using a parallel garbage collector, the default initial and maximum heap sizes are as follows for J2SE 6.0 [[Sun 06]]:
- initial heap size: larger of 1/64th of the machine's physical memory on the machine or some reasonable minimum
- maximum heap size: smaller of 1/4th of the physical memory or 1GB
/** Assuming the heap size as 512 MB (calculated as 1/4th of 2 GB RAM = 512 MB) * Considering long values being entered (64 bits each, the max number of elements * would be 512 MB/64bits = 67108864) */ public class ShowHeapError { Vector<Long> names = new Vector<Long>(); // Accepts unknown number of records long newID = 0L; int count = 67108865; int i = 0; InputStreamReader input = new InputStreamReader(System.in); Scanner reader = new Scanner(input); public void addNames(){ do{ // Adding unknown number of records to a list // The user can enter more number of IDs than what the heap can support and // exhaust the heap. Assume that the record ID is a 64 bit long value System.out.print("Enter recordID (To quit, enter -1): "); newID = reader.nextLong(); names.addElement(newID); i++; }while (i < count || newID != -1); // Close "reader" and "input" } public static void main(String[] args) { ShowHeapError demo = new ShowHeapError(); demo.addNames(); } }
Compliant Solution
The OutOfMemoryError
can be avoided by making sure that there are no infinite loops, memory leaks or unnecessary object retention. If memory requirements are known ahead of time, the heap size in Java can be tailored to fit the requirements using the following runtime parameters [[Java 06]]:
java -Xms<initial heap size> -Xmx<maximum heap size>
For example:
java -Xms128m -Xmx512m ShowHeapError
Here the initial heap size is set to 128 MB and the maximum heap size to 512 MB.
This setting can be changed either using the Java Control Panel or from the command line. It cannot be adjusted through the application itself.
Noncompliant Code Example
According to the Java API [[API 06]], Class ObjectInputStream
documentation:
ObjectOutputStream
andObjectInputStream
can provide an application with persistent storage for graphs of objects when used with aFileOutputStream
andFileInputStream
respectively.ObjectInputStream
is used to recover the objects previously serialized. Other uses include passing objects between hosts using a socket stream or for marshaling and unmarshaling arguments and parameters in a remote communication system.
By design, only the first time an object is written, does it get reflected in the stream. Subsequent writes write a handle to the object into the stream. A table mapping the objects written to the stream to the corresponding handle is also maintained. Because of this handle, references that may not persist during normal runs of the program are also retained. This can cause an OutOfMemoryError
when streams remain open for extended durations.
FileOutputStream fos = new FileOutputStream("data.txt"); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(new Date()); // ...
Compliant Solution (3)
If heap related issues arise, it is recommended that the ObjectOutputStream.reset()
method be called so that references to previously written objects may be garbage collected.
FileOutputStream fos = new FileOutputStream("data.txt"); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(new Date()); oos.reset(); // Reset the Object-Handle table to its initial state // ...
Risk Assessment
Assuming that infinite heap space is available can result in denial of service.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC07- J |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website
Other Languages
This rule appears in the C Secure Coding Standard as MEM11-C. Do not assume infinite heap space.
This rule appears in the C++ Secure Coding Standard as MEM12-CPP. Do not assume infinite heap space.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Sun 06]] Garbage Collection Ergonomics, "Default values for the Initial and Maximum heap size"
[[Java 06]] java - the Java application launcher, "Syntax for increasing the heap size"
[[Sun 03]] Chapter 5: Tuning the Java Runtime System, Tuning the Java Heap
[[API 06]] Class ObjectInputStream and ObjectOutputStream
[[SDN 08]] Serialization FAQ
[[MITRE 09]] CWE ID 400 "Uncontrolled Resource Consumption (aka 'Resource Exhaustion')"
MSC06-J. Finish every set of statements associated with a case label with a break statement 49. Miscellaneous (MSC) MSC30-J. Generate truly random numbers