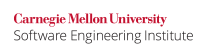
Threads always preserve class invariants when they are allowed to exit normally. Programmers often try to forcefully terminate threads when they believe that the task is accomplished, the request has been canceled or the program needs to quickly shutdown.
A few thread APIs were introduced to facilitate thread suspension, resumption and termination but were later deprecated due to inherent design weaknesses. The Thread.stop()
method is one example. It throws a ThreadDeath
exception to stop the thread. Two cases arise:
- If
ThreadDeath
is left uncaught, it allows the execution of afinally
block which performs the usual cleanup operations. Use of theThread.stop()
method is highly inadvisable because of two reasons. First, no particular thread can be forcefully stopped because an arbitrary thread can catch the thrownThreadDeath
exception and simply choose to ignore it. Second, stopping threads abruptly results in the release of all the associated monitors, violating the guarantees provided by the critical sections. Moreover, the objects end up in an inconsistent state, nondeterministic behavior being a typical outcome.
- As a remediation measure, catching the
ThreadDeath
exception on the other hand can itself ensnarl multithreaded code. For one, the exception can be thrown anywhere, making it difficult to trace and effectively recover from the exceptional condition. Also, there is nothing stopping a thread from throwing anotherThreadDeath
exception while recovery is in progress.
Noncompliant Code Example (Deprecated Thread.stop()
)
This noncompliant code example shows a thread that forcefully comes to a halt when the Thread.stop()
method is invoked. Neither the catch
nor the finally
block executes. Any monitors that are held are immediately released, leaving the object in a delicate state.
Try to retain the debug statements in the NCE to illustrate the problem
class BadStop implements Runnable { public void run() { try { Thread.currentThread().sleep(1000); } catch(InterruptedException ie) { // Not executed System.out.println("Performing cleanup"); } finally { // Not executed System.out.println("Closing resources"); } System.out.println("Done!"); } } class Controller { public static void main(String[] args) { Thread t = new Thread(new BadStop()); t.start(); t.interrupt(); // Artificially induce an InterruptedException t.stop(); // Force thread cancellation } }
The Thread.interrupt()
method is frequently used to awaken a blocked thread before it can be stopped. It awakens threads that are blocked on wait()
methods of class Object
, and join
and sleep
methods of class Thread
. In these cases, the thread's interrupt status is cleared and it receives an InterruptedException
. If the thread is blocked on I/O operations upon an interruptible channel, the channel is closed, the thread's interrupt status is set and it receives a ClosedByInterruptException
. Similarly, a thread waiting on a selector also returns from the operation with its interrupted status set. [[API 06]]
The java.lang.ThreadGroup.interrupt()
is not deprecated and is often seen as an option to interrupt all the threads belonging to a thread group. "This is no guarantee that a program will terminate, however, because libraries that you have used may have created user threads that do not respond to interrupt requests. The AWT graphics library is one well-known example." [[JPL 06]]. Moreover, using the ThreadGroup
API is discouraged (see [CON17-J. Avoid using ThreadGroup APIs]).
Compliant Solution (1) (volatile
flag)
This compliant example uses a boolean
flag called done
to indicate whether the thread should be stopped after any necessary cleanup code has finished executing. An accessor method shutdown()
is used to set the flag to true
, after which the thread can start the cancellation process. The done
flag is also set immediately after the execution of the finally
block's resource clean-up statements so that the system does not continue relinquishing resources that it has already released, in the event of the done
flag staying false
.
class ControlledStop implements Runnable{ protected volatile boolean done = false; public void run() { while(!done) { try { Thread.currentThread().sleep(1000); } catch(InterruptedException ie) { // Handle the exception } finally { done = true; } } done = false; // Reset for later use System.out.println("Done!"); } protected void shutdown(){ done = true; } } class Controller { public static void main(String[] args) throws InterruptedException { ControlledStop c = new ControlledStop(); Thread t = new Thread(c); t.start(); t.interrupt(); // Artificially induce an InterruptedException Thread.sleep(1000); // Wait for some time to allow the exception // to be caught (demonstration only) c.shutdown(); } }
Compliant Solution (2) (RuntimePermission stopThread
)
Remove the default permission java.lang.RuntimePermission
stopThread
from the security policy file to deny the Thread.stop()
invoking code, the required privileges.
Noncompliant Code Example (blocking IO)
This noncompliant code example uses the advice suggested in the previous compliant solution. However, this does not help in terminating the thread because it is blocked on some network IO as a consequence of using the readLine()
method.
class StopSocket extends Thread { private Socket s; private volatile boolean done = false; public void run() { while(!done) { try { s = new Socket("somehost", 25); BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream())); String s = null; while((s = br.readLine()) != null) { // Blocks until end of stream (null) } } catch (IOException ie) { // Forward to handler } finally { done = true; } } } public void shutdown() throws IOException { done = true; } } class Controller { public static void main(String[] args) throws InterruptedException, IOException { StopSocket ss = new StopSocket(); Thread t = new Thread(ss); t.start(); Thread.sleep(1000); ss.shutdown(); } }
A Socket
connection is not affected by the InterruptedException
that results with the use of the Thread.interrupt()
method. The boolean
flag solution does not work in such cases.
Compliant Solution (close socket connection)
This compliant solution closes the socket connection, both using the shutdown()
method as well as the finally
block. As a result, the thread is bound to stop due to a SocketException
. Note that there is no way to keep the connection alive if the thread is to be cleanly halted immediately.
class StopSocket extends Thread { private Socket s; public void run() { try { s = new Socket("somehost", 25); BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream())); String s = null; while((s = br.readLine()) != null) { // Blocks until end of stream (null) } } catch (IOException ie) { // Handle the exception } finally { try { if(s != null) s.close(); } catch (IOException e) { /* Forward to handler */ } } } public void shutdown() throws IOException { if(s != null) s.close(); } } class Controller { public static void main(String[] args) throws InterruptedException, IOException { StopSocket ss = new StopSocket(); Thread t = new Thread(ss); t.start(); Thread.sleep(1000); ss.shutdown(); } }
A boolean
flag can be used (as described earlier) if additional clean-up operations need to be performed.
Compliant Solution (2) (interruptible channel)
This compliant solution uses an interruptible channel, SocketChannel
instead of a Socket
connection. If the thread performing the network IO is interrupted using the Thread.interrupt()
method, for instance, while reading the data, the thread receives a ClosedByInterruptException
and the channel is closed immediately. The thread's interrupt status is also set.
class StopSocket extends Thread { private volatile boolean done = false; public void run() { while(!done) { try { InetSocketAddress addr = new InetSocketAddress("somehost", 25); SocketChannel sc = SocketChannel.open(addr); ByteBuffer buf = ByteBuffer.allocate(1024); sc.read(buf); // ... } catch (IOException ie) { // Handle the exception } finally { done = true; } } } public void shutdown() throws IOException { done = true; } }
Risk Assessment
Trying to force thread shutdown can result in inconsistent object state and corrupt the object. Critical resources may also leak if cleanup operations are not carried out as required.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON13- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Thread, method stop
[[Darwin 04]] 24.3 Stopping a Thread
[[JDK7 08]] Concurrency Utilities, More information: Java Thread Primitive Deprecation
[[JPL 06]] 14.12.1. Don't stop and 23.3.3. Shutdown Strategies
[[JavaThreads 04]] 2.4 Two Approaches to Stopping a Thread
[!The CERT Sun Microsystems Secure Coding Standard for Java^button_arrow_left.png!] [!The CERT Sun Microsystems Secure Coding Standard for Java^button_arrow_up.png!] [!The CERT Sun Microsystems Secure Coding Standard for Java^button_arrow_right.png!]