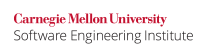
Reading a shared primitive variable in one thread may not yield the value of the latest write to the variable from another thread. It is important to ensure that a read of a shared variable sees the value of the most recent write to the variable. If this is not done, multiple threads may observe stale values of the shared variable and fail to act accordingly. Visibility of the latest value can be ensured by declaring the variable volatile
or correctly synchronizing the reads and writes to the variable.
The use of volatile
is recommended under a very restrictive set of conditions [[Goetz 06]]:
- A write to a variable does not depend on its current value
- The write is not involved with writes of other variables
- Only a single thread ever updates the value
- Locking is not required for any other reason
Synchronizing the code makes it easier to reason about the behavior of the code and is frequently, a more secure approach than using volatile
. However, it is slightly more expensive and can cause deadlocks when used excessively.
Declaring a variable as volatile or correctly synchronizing the code guarantees that 64-bit primitive variables of type long
and double
are accessed atomically (see CON25-J. Ensure atomicity when reading and writing 64-bit values for information on sharing long
and double
variables among multiple threads).
Noncompliant Code Example
This noncompliant code example uses a shutdown()
method to set a non-volatile done
flag that is checked in the run()
method.
final class ControlledStop implements Runnable { private boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } public void shutdown() { done = true; } }
If one thread invokes the shutdown()
method to set the flag, it is possible that another thread might not observe this change. Consequently, the second thread may observe that done
is still false
and incorrectly invoke the sleep()
method. In fact, a compiler is allowed to optimize the code if it determines that the value of done
is never modified by the same thread, so that the loop never terminates.
Compliant Solution (volatile
)
This compliant solution declares the done
flag as volatile so that updates are visible to other threads.
final class ControlledStop implements Runnable { private volatile boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } public void shutdown() { done = true; } }
Compliant Solution (java.util.concurrent.atomic.AtomicBoolean
)
This compliant solution uses an AtomicBoolean
flag to ensure that updates are visible to other threads.
final class ControlledStop implements Runnable { private AtomicBoolean done = new AtomicBoolean(false); public void run() { while (!done.get()) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } public void shutdown() { done.set(true); } }
Compliant Solution (synchronized
)
This compliant solution uses the intrinsic lock of the Class
object to ensure that updates are visible to other threads.
final class ControlledStop implements Runnable { private boolean done = false; public void run() { while (!isDone()) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } public synchronized boolean isDone() { return done; } public synchronized void shutdown() { done = true; } }
While this is an acceptable compliant solution, it has the following shortcomings as compared to the previously suggested ones:
- Performance: The intrinsic locks cause threads to block temporarily;
volatile
incurs no blocking - Deadlock: Excessive synchronization can make the program deadlock prone.
However, synchronization is a more secure alternative in situations where the volatile
keyword or an java.util.concurrent.atomic.Atomic*
field is inappropriate, such as if a variable's new value depends on its old value. Refer to CON01-J. Ensure that compound operations on shared variables are atomic for more information.
Exceptions
EX1: An array of class objects need not be made visible because class objects are created by the Virtual Machine and their initialization always precedes any subsequent use. JMM Mailing List
Risk Assessment
Failing to ensure visibility of shared primitive variables on accesses can lead to a thread seeing stale values of the variables.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON00- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[Goetz 06]] 3.4.2. "Example: Using Volatile to Publish Immutable Objects"
[[JPL 06]] 14.10.3. "The Happens-Before Relationship"
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object