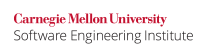
Sometimes you will want to construct a object to be shared among multiple threads. While being initialized, the object must remain exclusive to the thread constructing it, but once initialized, it can be 'published'. that is, made visible to other threads. However, the Java Memory Model permits a compiler to modify the order of statements to that a semmingly innocuous publication turns out to permit multiple threads to have access to an object before it is fully constructed.
Noncompliant Code Example
This noncompliant code example initializes a Helper
object inside a Foo
object.
public class Helper { private int n; public Helper(int n) { this.n = n; } // other fields & methods } class Foo { private Helper helper; public Helper getHelper() {return helper;} public void initialize() { helper = new Helper(42); } }
Suppose two threads have access to the same Foo
object, and initialize()
hasn't been called yet. Then both threads will see that getHelper()
returns null
. If one thread calls initialize()
, and the other thread calls getHelper(), the second thread might receive null
. Or it might receive a fully-initialized Helper
object, with the n
field set to 42. Or it might receive a partially-initialized Helper
object where n
is not initialized; that is n
has the value 0
.
In particular, the JMM permits compilers to allocate memory for the new Helper object and assign it to the helper field, then subsequently initialize it. This provides a race window during which other threads might see a partially-initialized helper object.
Compliant Solution (volatile
)
If the helper
field is declared volatile
, then it is guaranteed to be fully constructed before becoming visible.
class Foo { private volatile Helper helper; public Helper getHelper() {return helper;} public void initialize() { helper = new Helper(42); } }
Compliant Solution (final
)
If the helper
field is declared final, then it is guaranteed to be fully constructed before becoming visible.
class Foo { private final Helper helper; public Helper getHelper() {return helper;} public void initialize() { helper = new Helper(42); } }
Of course, this prevents you from subsequently setting the helper
field to a new object.
Compliant Solution (immutable
)
If the Helper
class is immutable, then it is guaranteed to be fully constructed before becoming visible. The object must be truly immutable; it is not sufficient for the program to refrain from modifying the object.
public class Helper { private final int n; public Helper(int n) { this.n = n; } // other fields & methods, all fields are final }
Note that if the Helper
object is mutable, you not only need to worry about the visibility of partially-constructed objects, but you also need to worry about the object being modified while read after it has been successfully constructed. For more information see CON11-J. Do not assume that declaring an object volatile guarantees visibility of its members.
Compliant Solution (synchronized
)
You can also prevent a partially-constructed object from becoming visible by using locks to control access to the object.
class Foo { private Helper helper; public synchronized Helper getHelper() {return helper;} public synchronized void initialize() { helper = new Helper(42); } }
Synchronizing both methods guarantees that they will never run simultaneously in different threads. If one thread calls initialize()
just before another thread calls getHelper()
, then the initialize()
block is guaranteed to finish, including the construction of the Helper
object, before getHelper()
starts. Consequently, the helper
field will only be visible after it is fully constructed.
Compliant Solution (thread-safe composition)
Some collection classes provide thread-safety over their objects. If the helper
field is contained in such a collection, then it is guaranteed to be fully constructed before becoming visible. The following code encases the helper field in a Vector.
class Foo { private Vector<Helper> helper; public Helper getHelper() {return helper.elementAt(0);} public void initialize() { helper = new Vector<Helper>(); helper.add(new Helper(42)); } }
Compliant Solution (static initialization)
In this compliant solution, the helper
field is initialized in a static block. When initialized statically, any object is guaranteed to be fully constructed before becoming visible.
class Foo { private static Helper helper = new Helper(42); public static Helper getHelper() {return helper;} }
Of course, this requires that the helper
field be static.
Risk Assessment
Failing to synchronize access to shared mutable data can cause different threads to observe different states of the object.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON26-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[Bloch 01]] Item 48: "Synchronize access to shared mutable data"
[[Goetz 06]] Section 3.5.3 "Safe Publication Idioms"
[[Goetz 07]] Pattern #2: "one-time safe publication"
[[Pugh 04]]
FIO36-J. Do not create multiple buffered wrappers on an InputStream 09. Input Output (FIO) 09. Input Output (FIO)