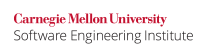
There are certain problems associated with the incorrect use of the Executor
interface. For instance, tasks that depend on other tasks should not execute in the same thread pool. A task that submits another task to a single threaded Executor
remains blocked until the results are received whereas the second task may have dependencies on the first task. This constitutes a deadlock.
Noncompliant Code Example
This noncompliant code example shows a thread starvation deadlock. This situation not only occurs in single threaded Executors, but also in those with large Thread Pools. This can happen when all the threads executing in the pool are blocked on tasks that are waiting on the queue. A blocking operation within a subtask can also lead to unbounded queue growth. [[Goetz 06]]
// Field password is defined in class InitialHandshake class NetworkServer extends InitialHandshake implements Runnable { private final ServerSocket serverSocket; private final ExecutorService pool; public NetworkServer(int port, int poolSize) throws IOException { serverSocket = new ServerSocket(port); pool = Executors.newFixedThreadPool(poolSize); } public void run() { try { // Interdependent tasks pool.submit(new SanitizeInput(password)); // password is defined in class InitialHandshake pool.submit(new CustomHandshake(password)); // for e.g. client puzzles pool.execute(new Handle(serverSocket.accept())); // Handle connection } catch (IOException ex) { pool.shutdown(); } } }
In this noncompliant code example, the SanitizeInput
task depends upon the CustomHandshake
task for the value of password
whereas the latter depends on the former to return a password
that has been correctly sanitized.
Compliant Solution
This compliant solution recommends executing the interdependent tasks as a single task within the Executor
. In other cases, where the subtasks do not require concurrency safeguards, the subtasks can be moved outside the threaded region that is required to be executed by the Executor
.
class NetworkServer extends InitialHandshake implements Runnable { private final ServerSocket serverSocket; private final ExecutorService pool; public NetworkServer(int port, int poolSize) throws IOException { serverSocket = new ServerSocket(port); pool = Executors.newFixedThreadPool(poolSize); } public void run() { try { // Execute interdependent subtasks as a single combined task within this block // Tasks SanitizeInput() and CustomHandshake() are performed together in Handle() pool.execute(new Handle(serverSocket.accept())); // Handle connection } catch (IOException ex) { pool.shutdown(); } } }
Always try to submit independent tasks to the Executor
. Thread starvation issues can be mitigated by choosing a large pool size. Note that operations that have further constraints, such as the total number of database connections or total ResultSets
open at a particular time, impose an upper bound on the thread pool size as each thread continues to block until the resource becomes available. The other rules of fair concurrency, such as not running time consuming tasks, also apply. When this is not possible, expecting to obtain real time result guarantees from the execution of tasks is conceivably, an unreasonable target.
Sometimes, a private static
ThreadLocal
variable is used per thread to maintain local state. When using thread pools, ThreadLocal
variable should be used only if their lifetime is shorter than that of the corresponding task [[Goetz 06]]. Moreover, such variables should not be used as a communication mechanism between tasks.
Risk Assessment
Executing interdependent tasks in a thread pool can lead to denial of service.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON29- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
FIO36-J. Do not create multiple buffered wrappers on an InputStream 09. Input Output (FIO) 09. Input Output (FIO)