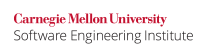
Reads of shared primitive variables in some thread may not observe the latest writes to them from other threads. It is important to ensure that the accesses see the value of the latest writes. If this is not done, multiple threads may observe stale values of the shared variables and fail to act accordingly. Visibility of latest values can be ensured by declaring variables volatile
or correctly synchronizing the code.
The use of volatile
is recommended under a very restrictive set of conditions:
- A write to a variable does not depend on its current value
- The write is not involved with writes of other variables
Both approaches also guarantee that 64-bit primitive variables of type long
and double
will always be accessed atomically (see CON25-J. Ensure atomicity when reading and writing 64-bit values for information on sharing long
and double
variables among multiple threads).
Noncompliant Code Example
This noncompliant code example uses a shutdown()
method to set a non-volatile done
flag that is checked in the run()
method. If one thread invokes the shutdown()
method to set the flag, it is possible that another thread might not observe this change. Consequently, the second thread may still observe that done
is false
and incorrectly invoke the sleep()
method.
final class ControlledStop implements Runnable { private boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } protected void shutdown() { done = true; } }
Compliant Solution (volatile
)
This compliant solution declares the done
flag as volatile
so that updates are visible to other threads.
final class ControlledStop implements Runnable { private volatile boolean done = false; public void run() { while (!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } protected void shutdown() { done = true; } }
Compliant Solution (synchronized
)
This compliant solution uses the intrinsic lock of the Class
object to ensure thread safety.
final class ControlledStop implements Runnable { private boolean done = false; public void run() { while (!isDone()) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle exception } } } protected synchronized boolean isDone() { return done; } protected synchronized void shutdown() { done = true; } }
While this is an acceptable compliant solution, it has the following disadvantages compared to declaring done
as volatile
:
- Performance: The intrinsic locks cause threads to block temporarily;
volatile
incurs no blocking - Deadlock: Improper use of locks can lead to deadlocks. Because the use of
volatile
incurs no blocking, deadlock cannot occur.
However, synchronization is a useful alternative in situations where the volatile
keyword is inappropriate, such as if a variable's new value depends on its old value. Refer to CON01-J. Do not assume that composite operations are atomic and CON07-J. Do not assume that a grouping of calls to independently atomic methods is atomic for more details.
Risk Assessment
Failing to ensure visibility of atomically modifiable shared variables can lead to a thread seeing stale values of a variable.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON00- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[Goetz 06]] 3.4.2. "Example: Using Volatile to Publish Immutable Objects"
[[JPL 06]] 14.10.3. "The Happens-Before Relationship"
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object