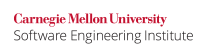
Threads always preserve class invariants when they are allowed to exit normally. Programmers often try to forcefully terminate threads when they believe that the task is accomplished, the request has been canceled or the program needs to quickly shutdown.
A few thread APIs were introduced to facilitate thread suspension, resumption and termination but were later deprecated due to inherent design weaknesses. The Thread.stop()
method is one example. It throws a ThreadDeath
exception to stop the thread. Two cases arise:
- If
ThreadDeath
is left uncaught, it allows the execution of afinally
block which performs the usual cleanup operations. Use of theThread.stop()
method is highly inadvisable because of two reasons. First, no particular thread can be forcefully stopped because an arbitrary thread can catch the thrownThreadDeath
exception and simply choose to ignore it. Second, abruptly stopping a thread results in the release of all the locks that it has acquired, violating the guarantees provided by the critical sections. Moreover, the objects end up in an inconsistent state, non-deterministic behavior being a typical outcome.
- As a remediation measure, catching the
ThreadDeath
exception on the other hand can itself ensnarl multithreaded code. For one, the exception can be thrown anywhere, making it difficult to trace and effectively recover from the exceptional condition. Also, there is nothing stopping a thread from throwing anotherThreadDeath
exception while recovery is in progress.
More information about deprecated methods is available in MET15-J. Do not use deprecated or obsolete methods.
Noncompliant Code Example (Deprecated Thread.stop()
)
This noncompliant code example shows a thread that fills a vector with strings. The thread is stopped after a fixed amount of time.
public class Container implements Runnable { private final Vector<String> vector = new Vector<String>(); private final BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); public Vector<String> getVector() { return vector; } public synchronized void run() { String string = null; do { System.out.println("Enter another string"); try { string = in.readLine(); } catch (IOException e) { // Forward to handler } vector.add(string); } while (!"END".equals(string)); } public static void main(String[] args) throws InterruptedException { Thread thread = new Thread(new Container()); thread.start(); Thread.sleep(5000); thread.stop(); } }
Because the class Vector
is thread-safe, operations performed by multiple threads on its shared instance are expected to leave it in a consistent state. For instance, the Vector.size()
method always reflects the true number of elements in the vector even when an element is added or removed. This is because the vector instance uses its own intrinsic lock to prevent other threads from accessing it while its state is temporarily inconsistent.
However, the Thread.stop()
method causes the thread to stop what it is doing and throw a ThreadDeath
exception, and release all locks that it has acquired [[API 06]]. If the thread is in the process of adding a new string to the vector when it is stopped, the vector may become accessible while it is in an inconsistent state. For instance, Vector.size()
may be three while the vector only contains two elements.
Compliant Solution (volatile
flag)
This compliant solution stops the thread by using a volatile
flag. An accessor method shutdown()
is used to set the flag to true
, after which the thread can start the cancellation process.
public class Container implements Runnable { private final Vector<String> vector = new Vector<String>(); private final BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); private volatile boolean done = false; public Vector<String> getVector() { return vector; } public void shutdown() { done = true; } public synchronized void run() { String string = null; do { System.out.println("Enter another string"); try { string = in.readLine(); } catch (IOException e) { // Forward to handler } vector.add(string); } while (!done && !"END".equals(string)); } public static void main(String[] args) throws InterruptedException { Container container = new Container(); Thread thread = new Thread(container); thread.start(); Thread.sleep(5000); container.shutdown(); } }
Compliant Solution (Interruptible)
This compliant solution stops the thread by using the Thread.interrupted()
method.
public class Container implements Runnable { private final Vector<String> vector = new Vector<String>(); private final BufferedReader in = new BufferedReader(new InputStreamReader(System.in)); public Vector<String> getVector() { return vector; } public synchronized void run() { String string = null; do { System.out.println("Enter another string"); try { string = in.readLine(); } catch (IOException e) { // Forward to handler } vector.add(string); } while (!Thread.interrupted() && !"END".equals(string)); } public static void main(String[] args) throws InterruptedException { Container c = new Container(); Thread thread = new Thread(c); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
This method interrupts the current thread, however, it does not stop the thread unless the logic polls the interrupted flag using the method Thread.interrupted()
to stop performing any pending actions.
Compliant Solution (RuntimePermission stopThread
)
Remove the default permission java.lang.RuntimePermission
stopThread
from the security policy file to deny the Thread.stop()
invoking code, the required privileges.
Noncompliant Code Example (blocking IO)
This noncompliant code example uses the advice suggested in the previous compliant solution. However, this does not help in terminating the thread because it is blocked on some network IO as a consequence of using the readLine()
method.
class StopSocket extends Thread { private Socket s; private volatile boolean done = false; public void run() { while(!done) { try { s = new Socket("somehost", 25); BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream())); String s = null; while((s = br.readLine()) != null) { // Blocks until end of stream (null) } } catch (IOException ie) { // Forward to handler } finally { done = true; } } } public void shutdown() throws IOException { done = true; } } class Controller { public static void main(String[] args) throws InterruptedException, IOException { Thread thread = new Thread(new Container()); t.start(); Thread.sleep(1000); ss.shutdown(); } }
A Socket
connection is not affected by the InterruptedException
that results with the use of the Thread.interrupt()
method. The boolean
flag solution does not work in such cases.
Compliant Solution (close socket connection)
This compliant solution closes the socket connection, both using the shutdown()
method as well as the finally
block. As a result, the thread is bound to stop due to a SocketException
. Note that there is no way to keep the connection alive if the thread is to be cleanly halted immediately.
class StopSocket extends Thread { private Socket s; public void run() { try { s = new Socket("somehost", 25); BufferedReader br = new BufferedReader(new InputStreamReader(s.getInputStream())); String s = null; while((s = br.readLine()) != null) { // Blocks until end of stream (null) } } catch (IOException ie) { // Handle the exception } finally { try { if(s != null) s.close(); } catch (IOException e) { /* Forward to handler */ } } } public void shutdown() throws IOException { if(s != null) s.close(); } } class Controller { public static void main(String[] args) throws InterruptedException, IOException { StopSocket ss = new StopSocket(); Thread t = new Thread(ss); t.start(); Thread.sleep(1000); ss.shutdown(); } }
A boolean
flag can be used (as described earlier) if additional clean-up operations need to be performed.
Compliant Solution (2) (interruptible channel)
This compliant solution uses an interruptible channel, SocketChannel
instead of a Socket
connection. If the thread performing the network IO is interrupted using the Thread.interrupt()
method, for instance, while reading the data, the thread receives a ClosedByInterruptException
and the channel is closed immediately. The thread's interrupt status is also set.
class StopSocket extends Thread { private volatile boolean done = false; public void run() { while(!done) { try { InetSocketAddress addr = new InetSocketAddress("somehost", 25); SocketChannel sc = SocketChannel.open(addr); ByteBuffer buf = ByteBuffer.allocate(1024); sc.read(buf); // ... } catch (IOException ie) { // Handle the exception } finally { done = true; } } } public void shutdown() throws IOException { done = true; } }
Risk Assessment
Trying to force thread shutdown can result in inconsistent object state and corrupt the object. Critical resources may also leak if cleanup operations are not carried out as required.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON13- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Thread, method stop
[[Darwin 04]] 24.3 Stopping a Thread
[[JDK7 08]] Concurrency Utilities, More information: Java Thread Primitive Deprecation
[[JPL 06]] 14.12.1. Don't stop and 23.3.3. Shutdown Strategies
[[JavaThreads 04]] 2.4 Two Approaches to Stopping a Thread
CON12-J. Avoid deadlock by requesting and releasing locks in the same order 11. Concurrency (CON) VOID CON14-J. Ensure atomicity of 64-bit operations