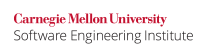
Generic code can be freely used with raw types when attempting to preserve compatibility between non-generic legacy code and newer generic code. However, using raw types with generic code will cause most Java compilers to issue "unchecked" warnings. When generic and non-generic code is used correctly, these warnings are not catastrophic, but the same warnings are issued when potentially unsafe operations are performed. If generic and non-generic code must be used together, these warnings should not be simply ignored.
According to [JLS 05] section 4.8 "Raw Types":
The use of raw types is allowed only as a concession to compatibility of legacy code. The use of raw types in code written after the introduction of genericity into the Java programming language is strongly discouraged. It is possible that future versions of the Java programming language will disallow the use of raw types.
If a parameterized type tries to access an object that is not of the parameterized type, heap pollution results. For instance, consider the code snippet below.
List l = new ArrayList<Integer>(); List<String> ls = l; // produces unchecked warning
However, one should not rely on unchecked warnings to implement this rule. According to [JLS 05] section 4.12.2.1 "Heap Pollution":
Note that this does not imply that heap pollution only occurs if an unchecked warning actually occurred. It is possible to run a program where some of the binaries were compiled by a compiler for an older version of the Java programming language, or by a compiler that allows the unchecked warnings to suppressed (sic). This practice is unhealthy at best.
Overriding legacy classes and generifying the overriding method is no panacea as this is made illegal by the Java Language Specification [JLS 05]. It is best to avoid mixing generic and non-generic code.
Noncompliant Code Example
This code ordinarily produces an unchecked warning because the raw type of the List.add()
method is being used (the list
parameter in addToList()
method) instead of the parameterized type. To make this compile cleanly, the @SuppressWarnings
annotation is used.
public class MixedTypes { @SuppressWarnings("unchecked") private static void addToList(List list, Object obj) { list.add(obj); // "unchecked" warning } private static void print() { List<String> list = new ArrayList<String> (); addToList(list, 1); System.out.println(list.get(0)); } public static void main(String[] args) { MixedTypes.print(); } }
When executed, this code produces an exception because list.get(0)
is not of the proper type.
Exception in thread "main" java.lang.ClassCastException: java.lang.Integer cannot be cast to java.lang.String at Raw.print(Test.java:11) at Raw.main(Test.java:14)
Compliant Solution
By gleaning information from the diagnostic exception message, the error can be quickly traced to the line addToList(1)
in the noncompliant code. Changing this to addToList("1")
is unfortunately a superficial defense. To resolve the real issue, use parameterized types consistently and not just abundantly. To enforce compile time checking of types, replace the parameters to addToList
with List<String> list
and String str
. Clearly, the compiler does not allow insertion of an Object
once list
is parameterized. Likewise, addToList()
cannot be called with an argument whose type produces a mismatch.
class Parameterized { private static void addToList(List<String> list, String str) { list.add(str); // "unchecked" warning } private static void print() { List<String> list = new ArrayList<String> (); addToList(list, "1"); System.out.println(list.get(0)); } public static void main(String[] args) { Parameterized.print(); } }
Noncompliant Code Example
This code suffers from related pitfalls. It compiles and runs cleanly. The method printOne
is intended to print the value one either as an int
or as a double
depending on the type of the variable type
. However, despite list
being correctly parameterized, this method will always print '1' and never '1.0' because the int value 1 is always added to list
without being type checked.
class BadListAdder { @SuppressWarnings("unchecked") private static void addToList(List list, Object obj) { list.add(obj); // "unchecked" warning } private static <T> void printOne(T type) { if (!(type instanceof Integer || type instanceof Double)) System.out.println("Cannot print in the supplied type"); List<T> list = new ArrayList<T> (); addToList(list, 1); System.out.println(list.get(0)); } public static void main(String[] args) { double d = 1; int i = 1; System.out.println(d); BadListAdder.printOne(d); System.out.println(i); BadListAdder.printOne(i); } }
This code produces the output:
1.0 1 1 1
Compliant Solution
If possible, the addToList()
method should be generified to eliminate possible type violations.
class GoodListAdder { private static void addToList(List<Integer> list, Integer i) { list.add(i); } private static void addToList(List<Double> list, Double d) { list.add(d); } private static <T> void printOne(T type) { if (type instanceof Integer) { List<Integer> list = new ArrayList<Integer> (); addToList(list, 1); System.out.println(list.get(0)); } else if (type instanceof Double) { List<Double> list = new ArrayList<Double> (); // This will not compile if addToList(list, 1) is used addToList(list, 1.0); System.out.println(list.get(0)); } else { System.out.println("Cannot print in the supplied type"); } } public static void main(String[] args) { double d = 1; int i = 1; System.out.println(d); GoodListAdder.printOne(d); System.out.println(i); GoodListAdder.printOne(i); } }
This code will compile cleanly and run as expected by printing
1.0 1.0 1 1
If addToList()
is externally defined (such as in a library or is an upcall method) and cannot be changed, the same compliant method printOne()
can be used as written above, but there will be no warnings if addToList(1)
is used instead of addToList(1.0)
. Great care must be taken to ensure type safety when generics are mixed with non-generic code.
Exceptions
EX1: Raw types must be used in class literals. For example, since List<Integer>.class
is illegal, one can use raw type List.class
. [Bloch 08]
EX2: The instanceof
operator cannot be used with generic types. Here, it is permissible to mix generic and raw code. [Bloch 08]
if(o instanceof Set) { // Raw type Set<?> m = (Set<?>) o; // Wildcard type ... }
Risk Assessment
Mixing generic and non-generic code may produce unexpected results and exceptional conditions.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC10-J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Langer 08]] Topic 3, "Coping with Legacy"
[[Bloch 08]] Item 23: "Don't use raw types in new code"
[[Bloch 07]] Generics, 1. "Avoid Raw Types in New Code"
[[Naftalin 06b]] "Principle of Indecent Exposure"
[[JLS 05]] 4.8 "Raw types" and 5.1.9 "Unchecked Conversion"