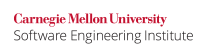
Sensitive data may be compromised if its lifetime is not limited to the period of its use. An adversary who has control of the filesystem may be able to access such data if the application:
- uses objects to store sensitive data whose values are not cleared or garbage collected after use
- has memory pages that can be swapped out to disk as required by the operating system (to perform memory management tasks and to support hibernation)
- uses any buffers to hold data (such as
BufferedReader
). The OS cache and in the in-memory copy of the data are also retained in this case. - bases its control flow on Reflection that circumvents any countermeasures to limit the lifetime of sensitive variables
- reveals sensitive data in debugging messages, log files, environment variables or through core dumps
Currently, complete mitigation requires support from the underlying operating system. For instance, if swapping out of sensitive data is an issue, a secure operating system that disables swapping and hibernation is indispensable.
Noncompliant Code Example
This noncompliant code example reads login information from the console and stores the password as a String
object. Consequently, the password may remain exposed until the garbage collector reclaims the memory associated with the object.
class BadPassword { public static void main (String args[]) throws IOException { Console c = System.console(); if (c == null) { System.err.println("No console."); System.exit(1); } String login = c.readLine("Enter your user name: "); String password = c.readLine("Enter your password: "); if (!verify(login, password)) { throw new IOException("Invalid Credentials"); } // ... } // dummy verify method, always returns true private static final boolean verify(String login, String password) { return true; } }
Compliant Solution
This compliant solution uses the Console.readPassword()
method to obtain the password from the console. This method allows the password to be returned as a sequence of characters as opposed to a String
object. This is advantageous as it gives the programmer more control over clearing the password from the array, immediately after use.
class GoodPassword { public static void main (String args[]) throws IOException { Console c = System.console(); if (c == null) { System.err.println("No console."); System.exit(1); } String login = c.readLine("Enter your user name: "); char [] password = c.readPassword("Enter your password: "); if (!verify(login, password)) { throw new IOException("Invalid Credentials"); } // ... Arrays.fill(password, ' '); } // dummy verify method, always returns true private static final boolean verify(String login, char[] password) { return true; } }
Noncompliant Code Example
This noncompliant code example uses a BufferedReader
to wrap an InputStreamReader
object so that sensitive data can be read from a file.
BufferedReader br = new BufferedReader(new InputStreamReader( new FileInputStream("file"))); // read from the file
Compliant Solution
This compliant solution uses a direct allocated NIO buffer to read sensitive data from the file. The data can be cleared immediately after use and is not cached or buffered in multiple locations. It exists only in the system memory.
private void readIntoDirectBuffer() throws IOException { ByteBuffer buffer = ByteBuffer.allocateDirect(16*1024); FileChannel rdr = (new FileInputStream("file")).getChannel(); while(rdr.read(buffer) > 0) { // do something with the buffer buffer.clear(); } rdr.close(); }
Risk Assessment
Failure to limit the lifetime of sensitive data can lead to sensitive information leaks.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC11- J |
medium |
likely |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class java.nio.ByteBuffer
[[Tutorials 08]] I/O from the Command Line
[[MITRE 09]] CWE ID 524 "Information Leak Through Caching", CWE ID 528
"Information Leak Through Core Dump Files", CWE ID 215
"Information Leak Through Debug Information", CWE ID 534
"Information Leak Through Debug Log Files" and CWE ID 526
"Information Leak Through Environmental Variables"
FIO36-J. Do not create multiple buffered wrappers on an InputStream 09. Input Output (FIO) 09. Input Output (FIO)