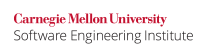
The Java Language Specification, Section 15.17.3, "Remainder Operator %" states
The remainder operation for operands that are integers after binary numeric promotion (§5.6.2) produces a result value such that (a/b)*b+(a%b) is equal to a. This identity holds even in the special case that the dividend is the negative integer of largest possible magnitude for its type and the divisor is -1 (the remainder is 0). It follows from this rule that the result of the remainder operation can be negative only if the dividend is negative, and can be positive only if the dividend is positive; moreover, the magnitude of the result is always less than the magnitude of the divisor.
The result of the remainder operator has the same sign as the dividend (the first operand in the expression).
5 % 3 produces 2 5 % (-3) produces 2 (-5) % 3 produces -2 (-5) % (-3) produces -2
Programmers could incorrectly assume that the remainder operation always returns a positive result and code based on that assumption.
Noncompliant Code Example
This noncompliant code example uses the integer hashKey
as an index into the hash
array. The hash key input could be negative, producing a negative result from the remainder operator. Consequently, the lookup function will throw a java.lang.ArrayIndexOutOfBoundsException
.
private int SIZE = 16; public int[] hash = new int[SIZE]; public int lookup(int hashKey) { return hash[hashKey % SIZE]; }
Compliant Solution
This compliant solution calls a method that returns a remainder that is always positive.
// method imod() gives non-negative result private int SIZE = 16; public int[] hash = new int[SIZE]; private int imod(int i, int j) { int temp = i % j; return (temp < 0) ? -temp : temp; // unary - will succeed without overflow // because temp cannot be Integer.MIN_VALUE } public int lookup(int hashKey) { return hash[imod(hashKey, size)]; }
Risk Assessment
Assuming a positive remainder when using the remainder operator can result in incorrect computations.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT02-J |
low |
unlikely |
high |
P1 |
L3 |
Automated Detection
Automated detection of uses of the %
operator is straightforward. Sound determination of whether those uses correctly reflect the intent of the programmer is infeasible in the general case. Heuristic warnings could be useful.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Secure Coding Standard: INT10-C. Do not assume a positive remainder when using the % operator
C++ Secure Coding Standard: INT10-CPP. Do not assume a positive remainder when using the % operator
Bibliography
[[JLS 2005]] Section 15.17.3 "Remainder Operators"
INT01-J. Check ranges before casting integers to narrower types Integers (INT) FLP10-J. Do not cast primitive integer types to floating-point types without range checks