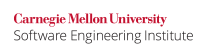
Sometimes null
is returned intentionally to account for zero available instances. This practice can lead to denial-of-service vulnerabilities when the client code does not explicitly handle the null
return case.
Noncompliant Code Example
This noncompliant code example returns a null
ArrayList
when the size of the ArrayList
is zero. The class Inventory
contains a getStock()
method that constructs a list of items that have zero inventory and returns the list of items to the caller. When the size of this list is zero, a null
is returned with the assumption that the client will install the necessary checks. Here, the client omits the check, causing a NullPointerException
at runtime.
class Inventory { private final Hashtable<String, Integer> items; public Inventory() { items = new Hashtable<String, Integer>(); } public List<String> getStock() { List<String> stock = new ArrayList<String>(); Enumeration itemKeys = items.keys(); while(itemKeys.hasMoreElements()) { Object value = itemKeys.nextElement(); if((items.get(value)) == 0) { stock.add((String)value); } } if(items.size() == 0) { return null; } else { return stock; } } } public class Client { public static void main(String[] args) { Inventory inv = new Inventory(); List<String> items = inv.getStock(); System.out.println(items.size()); // Throws an NPE } }
Compliant Solution
This compliant solution eliminates the null
return and simply returns the List
, even if it is zero-length. The client can effectively handle this situation without being interrupted by runtime exceptions. When arrays are returned instead of collections, care must be taken to ensure that the client does not access individual elements of a zero-length array. This prevents an ArrayOutOfBoundsException
.
class Inventory { private final Hashtable<String, Integer> items; public Inventory() { items = new Hashtable<String, Integer>(); } public List<String> getStock() { List<String> stock = new ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration itemKeys = items.keys(); while(itemkeys.hasMoreElements()) { Object value = itemKeys.nextElement(); if((noOfItems = items.get(value)) == 0) { stock.add((String)value); } } return stock; // Return list (possibly zero-length) } } public class Client { public static void main(String[] args) { Inventory inv = new Inventory(); List<String> items = inv.getStock(); System.out.println(items.size()); // Does not throw an NPE } }
Compliant Solution
This compliant solution returns an empty list, which is an equivalent, permissible technique.
public List<String> getStock() { List<String> stock = new ArrayList<String>(); Integer noOfItems; // Number of items left in the inventory Enumeration itemkeys = items.keys(); while(itemkeys.hasMoreElements()) { Object value = itemKeys.nextElement(); if((noOfItems = items.get(value)) == 0) { stock.add((String)value); } } if(l.isEmpty()) { return Collections.EMPTY_LIST; // Always zero-length } else { return stock; // Return list } } // Class Client ...
Risk Assessment
Returning null
rather than a zero-length array may lead to denial-of-service vulnerabilities when the client code does not handle null
properly.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MET55-JG | low | unlikely | high | P1 | L3 |
Automated Detection
Automatic detection is straightforward but fixing the problem will, most probably, require human intervention.
Related Guidelines
C Secure Coding Standard: MSC19-C. For functions that return an array, prefer returning an empty array over a null value
Bibliography
[Bloch 2008] Item 43: Return empty arrays or collections, not nulls