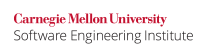
Hardcoding sensitive information, such as passwords, is an extremely dangerous practice. Doing so can have the following ominous effects -
- The sensitive information can become accessible to whoever has access to the source code, for example, the developers.
- Once the system goes into production, it can become unwieldy to manage and accommodate changes to the code.
- In certain cases, it can also violate the fundamental principle of recalling the memory used to store the sensitive information as soon as the required operation has concluded.
- Malicious users may use decompilation techniques to resurrect the hardcoded sensitive information. This is a critical security vulnerability.
Noncompliant Code Example
This noncompliant code example uses a password field instantiated as a String
.
class Hardcoded { String password = new String("guest"); public static void main(String[] args) { //.. } }
Notably, when the password is no longer required, it has to be left to the mercy of the garbage collector. This is because String
objects are immutable and continue to persist even after dereferencing them, until the garbage collector performs its job.
Secondly, a malcious user can use the javap -c Hardcoded
command to disassemble the class and uncover the hardcoded password. The output of the disassembler as shown below, makes available the password guest
in cleartext.
Compiled from "Hardcoded.java" class Hardcoded extends java.lang.Object{ java.lang.String password; Hardcoded(); Code: 0: aload_0 1: invokespecial #1; //Method java/lang/Object."<init>":()V 4: aload_0 5: new #2; //class java/lang/String 8: dup 9: ldc #3; //String guest 11: invokespecial #4; //Method java/lang/String."<init>":(Ljava/lang/String;)V 14: putfield #5; //Field password:Ljava/lang/String; 17: return public static void main(java.lang.String[]); Code: 0: return }
Compliant Solution
This compliant solution uses a char
array to store the password after it is retrieved from an external file. The password is immediately cleared out after use. This limits the exposure time.
class Password { public static void main(String[] args) throws IOException { char[] password = new char[100]; BufferedReader br = new BufferedReader(new InputStreamReader( new FileInputStream("password.txt"))); // reads the password into the char array, returns the number of bytes read int n = br.read(password); // decrypt password, perform operations for(int i= n - 1;i >= 0;i--) // manually clear out the password immediately after use password[i] = 0; } }
Risk Assessment
Hardcoding sensitive information can lead to critical security vulnerabilities.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC01-J |
high |
probable |
low |
P18 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Gong 03]] 9.4 Private Object State and Object Immutability
FIO35-J. Exclude user input from format strings 07. Input Output (FIO) 07. Input Output (FIO)