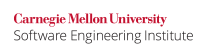
The Java Singleton pattern is a design pattern that governs the instantiation process. According to this design pattern, there can only be one instance of your class per JVM at any time. "Since there is only one Singleton instance, any instance fields of a Singleton will occur only once per class, just like static fields. Singletons often control access to resources such as database connections or sockets." [When is a Singleton not a Singleton?]
A typical implementation of a Singleton in Java is the creation of a single instance of the class as a static field.
The instance can be created using lazy initialization, which means that the instance is not created when the class loads but when it is first used.
Noncompliant Code Example
When the getter method is called by two (or more) threads or classes simultaneously, multiple instances of the Singleton class might result if one neglects to synchronize access.
class MySingleton { private static MySingleton _instance; private MySingleton() { // construct object . . . // private constructor prevents instantiation by outside callers } // lazy initialization // error, no synchronization on method access public static MySingleton getInstance() { if (_instance==null) { _instance = new MySingleton(); } return _instance; } // Remainder of class definition . . . }
Noncompliant Code Example
Multiple instances can be created even if you add a synchronized(this)
block to the constructor call.
// Also an error, synchronization does not prevent // two calls of constructor. public static MySingleton getInstance() { if (_instance==null) { synchronized (MySingleton.class) { _instance = new MySingleton(); } } return _instance; }
Compliant Solution
To avoid these issues, make getInstance()
a synchronized method.
class MySingleton { private static MySingleton _instance; private MySingleton() { // construct object . . . // private constructor prevents instantiation by outside callers } // lazy initialization public static synchronized MySingleton getInstance() { if (_instance==null) { _instance = new MySingleton(); } return _instance; } // Remainder of class definition . . . }
Applying a static modifier to the getInstance()
method which returns the Singleton allows the method to be accessed subsequently (after the initial call) without creating a new object.
Noncompliant Code Example
Another solution for Singletons to be thread-safe is double-checked locking. Unfortunately, it is not guaranteed to work because compiler optimizations can force the assignment of the new Singleton object before all its fields are initialized.
// double-checked locking public static MySingleton getInstance() { if (_instance==null) { synchronized (MySingleton.class) { if (_instance==null) { _instance = new MySingleton(); } } } }
Compliant Solution
It is still possible to create a copy of the Singleton object by cloning it using the object's clone
method, which violates the Singleton Design Pattern's objective. To prevent this, one needs to override the object's clone
method and throw a CloneNotSupportedException
exception from within it. [[Daconta 03]]
class MySingleton { private static MySingleton _instance; private MySingleton() { // construct object . . // private constructor prevents instantiation by outside callers } // lazy initialization public static synchronized MySingleton getInstance() { if (_instance==null) { _instance = new MySingleton(); } return _instance; } public Object clone() throws CloneNotSupportedException { throw new CloneNotSupportedException(); } // Remainder of class definition . . . }
See MSC05-J. Make your classes noncloneable unless required for more details about restricting the clone()
method.
To be fully compliant, it must be ensured that the class obeys the Singleton pattern's design contract. It is unreasonable to use the class for anything else, for example, as a method to share global state. [[Daconta 03]]
Risk Assessment
Using lazy initialization in a Singleton without synchronizing the getInstance() method may lead to multiple instances and can thus violate the expected contract.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON33-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Chapter 17, Threads and Locks
[[Fox 01]] When is a Singleton not a Singleton?
[[Daconta 03]] Item 15: Avoiding Singleton Pitfalls;
CON32-J. Prefer notifyAll() to notify() 08. Concurrency (CON) CON34-J. Avoid deadlock by requesting fine-grained locks in the proper order