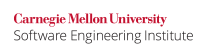
Generic code is free to be used with raw types, preserving the compatibility of non-generic legacy code and newer generic code. However, using raw types with generic code will cause the java compiler to issue "unchecked" warnings. When generic and non-generic code are used correctly together these warnings are no threat, but the same warnings are issued when unsafe operations are performed. If generic and non-generic code must be used together these warnings should not be simply ignored.
Noncompliant Code Example
This code ordinarily produces an unchecked warning because the raw type of the List.add method is being used instead of the parameterized type. To make this compile cleanly, the SuppressWarnings annotation is used.
import java.util.*; public class Test { @SuppressWarnings("unchecked") public static void addToList(List list, Object obj) { list.add(obj); // "unchecked" warning } public static void print() { List<String> list = new ArrayList<String> (); addToList(list, 1); System.out.println(list.get(0)); } public static void main(String[] args) { Test.print(); } }
When run this code will produce an exception because list.get(0)
is not of the proper type.
Exception in thread "main" java.lang.ClassCastException: java.lang.Integer cannot be cast to java.lang.String at Test.print(Test.java:11) at Test.main(Test.java:14)
Noncompliant Code Example
This code reveals the same sort of problem, but it will both compile and run cleanly.
import java.util.*; public class Test { @SuppressWarnings("unchecked") public static void addToList(List list, Object obj) { list.add(obj); // "unchecked" warning } public static <T> void printOne(T type) { if (!(type instanceof Integer || type instanceof Double)) System.out.println("Cannot print in the supplied type"); List<T> list = new ArrayList<T> (); addToList(list, 1); System.out.println(list.get(0)); } public static void main(String[] args) { double d = 1; int i = 1; System.out.println(d); Test.printOne(d); System.out.println(i); Test.printOne(i); } }
The method printOne
is inteded to print the value one either as an int or as a double depending on the type of the variable type
. However, despite list
being correctly parameterized, this method will always print '1' and never '1.0' because the int value 1 is always added to list
without being type checked.
The code will produce this output
1.0 1 1 1
Compliant Solution
If possible, the addToList
method should be generified to eliminate possible type violations.
import java.util.*; public class Test { public static void addToList(List<Integer> list, Integer obj) { list.add(obj); } public static void addToList(List<Double> list, Double obj) { list.add(obj); } public static <T> void printOne(T type) { if (type instanceof Integer) { List<Integer> list = new ArrayList<Integer> (); addToList(list, 1); System.out.println(list.get(0)); } else if (type instanceof Double) { List<Double> list = new ArrayList<Double> (); // This will not compile if addToList(list, 1) is used addToList(list, 1.0); System.out.println(list.get(0)); } else { System.out.println("Cannot print in the supplied type"); } } public static void main(String[] args) { double d = 1; int i = 1; System.out.println(d); Test.printOne(d); System.out.println(i); Test.printOne(i); } }
This code will compile cleanly and run as expected by printing
1.0 1.0 1 1
If addToList
is externally defined and cannot be changed, the same compliant method printOne
can be used as written above, but there will be no warnings if addToList(1)
is used instead of addToList(1.0)
. Great care must be taken to ensure type safety when generics are mixed with non-generic code.
Risk Assessment
Mixing generic and non-generic code may produce unexpected results or program termination.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MSC10-J |
medium |
unlikely |
high |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Langer 08]] Topic 3, "Coping with Legacy"