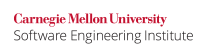
Threads always preserve class invariants when they are allowed to exit normally. Programmers often try to forcefully terminate threads when they believe that the task is accomplished, the request has been canceled or the program needs to quickly shutdown.
A few thread APIs were introduced to facilitate thread suspension, resumption and termination but were later deprecated because of inherent design weaknesses. The Thread.stop()
method is one example. It throws a ThreadDeath
exception to stop the thread. Two cases arise:
- If
ThreadDeath
is left uncaught, it allows the execution of afinally
block which performs the usual cleanup operations. Use of theThread.stop()
method is highly inadvisable because of two reasons. First, no particular thread can be forcefully stopped because an arbitrary thread can catch the thrownThreadDeath
exception and simply choose to ignore it. Second, abruptly stopping a thread results in the release of all the locks that it has acquired, violating the guarantees provided by the critical sections. Moreover, the objects end up in an inconsistent state, non-deterministic behavior being a typical outcome.
- As a remediation measure, catching the
ThreadDeath
exception on the other hand can itself ensnarl multithreaded code. For one, the exception can be thrown anywhere, making it difficult to trace and effectively recover from the exceptional condition. Also, there is nothing stopping a thread from throwing anotherThreadDeath
exception while recovery is in progress.
More information about deprecated methods is available in MET15-J. Do not use deprecated or obsolete methods.
Noncompliant Code Example (Deprecated Thread.stop()
)
This noncompliant code example shows a thread that fills a vector with pseudorandom numbers. The thread is stopped after a fixed amount of time.
public class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(); public Vector<Integer> getVector() { return vector; } public synchronized void run() { Random number = new Random(123L); int i = 10; while (i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Thread thread = new Thread(new Container()); thread.start(); Thread.sleep(5000); thread.stop(); } }
Because the class Vector
is thread-safe, operations performed by multiple threads on its shared instance are expected to leave it in a consistent state. For instance, the Vector.size()
method always reflects the true number of elements in the vector even when an element is added or removed. This is because the vector instance uses its own intrinsic lock to prevent other threads from accessing it while its state is temporarily inconsistent.
However, the Thread.stop()
method causes the thread to stop what it is doing and throw a ThreadDeath
exception, and release all locks that it has acquired [[API 06]]. If the thread is in the process of adding a new integer to the vector when it is stopped, the vector may become accessible while it is in an inconsistent state. For example, Vector.size()
may be two while the vector contains three elements (the element count is incremented after adding the element).
Compliant Solution (volatile
flag)
This compliant solution stops the thread by using a volatile
flag. An accessor method shutdown()
is used to set the flag to true
. The thread's run()
method polls the done
flag, and shuts down when it becomes true
.
public class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(); private volatile boolean done = false; public Vector<Integer> getVector() { return vector; } public void shutdown() { done = true; } public synchronized void run() { Random number = new Random(123L); int i = 10; while (!done && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container container = new Container(); Thread thread = new Thread(container); thread.start(); Thread.sleep(5000); container.shutdown(); } }
Compliant Solution (Interruptible)
This compliant solution stops the thread by using the Thread.interrupted()
method.
public class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(); public Vector<Integer> getVector() { return vector; } public synchronized void run() { Random number = new Random(123L); int i = 10; while (!Thread.interrupted() && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container c = new Container(); Thread thread = new Thread(c); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
This method interrupts the current thread, however, it only stops the thread because the thread logic polls the interrupted flag using the method Thread.interrupted()
, and shuts down when it is interrupted. No guarantees are provided by the JVM on when the interruption will be detected by blocking methods such as Thread.sleep()
and Object.wait()
[[Goetz 06]]. Upon receiving the interruption, the interrupted status of the thread is cleared and an InterruptedException
is thrown. Note that a thread should not be interrupted unless its interruption policy is known in advance. Failure to follow this advice can result in the corruption of mutable shared state.
Compliant Solution (RuntimePermission stopThread
)
Remove the default permission java.lang.RuntimePermission
stopThread
from the security policy file to deny the Thread.stop()
invoking code, the required privileges.
Noncompliant Code Example (blocking IO, volatile flag)
This noncompliant code example uses a volatile done
flag to indicate when the thread should shut down, as suggested above. However, this does not help in terminating the thread because it is blocked on some network IO as a consequence of using the readLine()
method.
class SocketReader implements Runnable { private final Socket socket; private final BufferedReader in; private volatile boolean done = false; private final Object lock = new Object(); private boolean isRunning = false; public static class MultipleUseException extends RuntimeException {}; public SocketReader() throws IOException { this.socket = new Socket("somehost", 25); this.in = new BufferedReader(new InputStreamReader(this.socket.getInputStream())); } // Only one thread can use the socket at a particular time public void run() { try { synchronized (lock) { if (isRunning) { throw new MultipleUseException(); } isRunning = true; } execute(); } catch (IOException ie) { // Forward to handler } } public void execute() throws IOException { String string; while (!done && (string = in.readLine()) != null) { // Blocks until end of stream (null) } } public void shutdown() { done = true; } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader(); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); reader.shutdown(); } }
Note that the Runnable
object prevents itself from being run in multiple threads using an isRunning()
flag. If one thread tries to invoke run()
after another thread has already done so, the method will exit with an exception. This exception is not recoverable, as it indicates a bug in the proper usage of this class, and hence, it may be unchecked.
Noncompliant Code Example (blocking IO, interruptible)
This noncompliant code example uses thread interruption to indicate when the thread should shut down, as suggested above. However, this does not help in terminating the thread because it is blocked on some network IO as a consequence of using the readLine()
method. Network I/O is not responsive to thread interruption.
class SocketReader implements Runnable { // ... public void execute() throws IOException { String string; while (!Thread.interrupted() && (string = in.readLine()) != null) { // Blocks until end of stream (null) } } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader(); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); thread.interrupt(); } }
Compliant Solution (close socket connection)
This compliant solution closes the socket connection, by having the shutdown()
method close the socket. As a result, the thread is bound to stop because of a SocketException
. Note that there is no way to keep the connection alive if the thread is to be cleanly halted immediately.
class SocketReader implements Runnable { // ... public void execute() throws IOException { String string; try { while ((string = in.readLine()) != null) { // Blocks until end of stream (null) } } finally { shutdown(); } } public void shutdown() throws IOException { socket.close(); } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader(); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); reader.shutdown(); } }
A boolean
flag can be used (as described earlier) if additional clean-up operations need to be performed.
Compliant Solution (interruptible channel)
This compliant solution uses an interruptible channel, SocketChannel
instead of a Socket
connection. If the thread performing the network IO is interrupted using the Thread.interrupt()
method, for instance, while reading the data, the thread receives a ClosedByInterruptException
and the channel is closed immediately. The thread's interrupt status is also set.
class SocketReader implements Runnable { private final SocketChannel sc; private final Object lock = new Object(); private boolean isRunning = false; private class MultipleUseException extends RuntimeException {}; public SocketReader() throws IOException { sc = SocketChannel.open(new InetSocketAddress("somehost", 25)); } public void run() { synchronized (lock) { if (isRunning) { throw new MultipleUseException(); } isRunning = true; } ByteBuffer buf = ByteBuffer.allocate(1024); try { synchronized (lock) { while (!Thread.interrupted()) { sc.read(buf); // ... } } } catch (IOException ie) { // Forward to handler } } public static void main(String[] args) throws IOException, InterruptedException { SocketReader reader = new SocketReader(); Thread thread = new Thread(reader); thread.start(); Thread.sleep(1000); thread.interrupt(); } }
This method interrupts the current thread, however, it only stops the thread because the thread logic polls the interrupted flag using the method Thread.interrupted()
, and shuts down when it is interrupted.
Noncompliant Code Example (database connection)
This noncompliant code example shows a thread-safe class DBConnector
that creates a JDBC connection per thread, that is, the connection belonging to one thread is not shared by other threads. This is a common use-case because JDBC connections are not meant to be shared by multiple-threads.
class DBConnector implements Runnable { final String query; private final Object lock = new Object(); private boolean isRunning = false; private class MultipleUseException extends RuntimeException {}; DBConnector(String query) { this.query = query; } public void run() { synchronized (lock) { if (isRunning) { throw new MultipleUseException(); } isRunning = true; } Connection con; try { // Username and password are hard coded for brevity con = DriverManager.getConnection("jdbc:myDriver:name", "username","password"); Statement stmt = con.createStatement(); ResultSet rs = stmt.executeQuery(query); } catch (SQLException e) { // Forward to handler } // ... } public static void main(String[] args) throws InterruptedException { DBConnector connector = new DBConnector(/* suitable query */); Thread thread = new Thread(connector); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
Unfortunately database connections, like sockets, are not inherently interruptible. So this design does not permit a client to cancel a task by closing it if the corresponding thread is blocked on a long running activity such as a join query. Furthermore, it is important to provide a mechanism to close connections to prevent thread starvation caused because of the limited number of database connections available in the pool. Similar task cancellation mechanisms are required when using objects local to a method, such as sockets.
Compliant Solution (ThreadLocal)
This compliant solution uses a ThreadLocal
wrapper around the connection so that a thread that calls initialValue()
obtains a unique connection instance. The advantage of this approach is that a shutdownConnection()
method can be provided so that clients external to the class can also close the connection when the corresponding thread is blocked, or is performing some time consuming activity.
class DBConnector implements Runnable { final String query; DBConnector(String query) { this.query = query; } private static ThreadLocal<Connection> connectionHolder = new ThreadLocal<Connection>() { Connection connection = null; @Override public Connection initialValue() { try { // Username and password are hard coded for brevity connection = DriverManager.getConnection ("jdbc:driver:name", "username","password"); } catch (SQLException e) { // Forward to handler } return connection; } @Override public void set(Connection con) { if(connection == null) { // Shuts down connection when null value is passed try { connection.close(); } catch (SQLException e) { // Forward to handler } } else { connection = con; } } }; public static Connection getConnection() { return connectionHolder.get(); } public static void shutdownConnection() { // Allows client to close connection anytime connectionHolder.set(null); } public void run() { Connection dbConnection = getConnection(); Statement stmt; try { stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery(query); } catch (SQLException e) { // Forward to handler } // ... } public static void main(String[] args) throws InterruptedException { DBConnector connector = new DBConnector(/* suitable query */); Thread thread = new Thread(connector); thread.start(); Thread.sleep(5000); connector.shutdown(); } }
Noncompliant Code Example (database connection)
This noncompliant code example shows a thread-safe class DBConnector
that creates a JDBC connection per thread, that is, the connection belonging to one thread is not shared by other threads. This is a common use-case because JDBC connections are not meant to be shared by multiple-threads.
class DBConnector implements Runnable { final String query; private final Object lock = new Object(); private boolean isRunning = false; private class MultipleUseException extends RuntimeException {}; DBConnector(String query) { this.query = query; } public void run() { synchronized (lock) { if (isRunning) { throw new MultipleUseException(); } isRunning = true; } Connection con; try { // Username and password are hard coded for brevity con = DriverManager.getConnection("jdbc:myDriver:name", "username","password"); Statement stmt = con.createStatement(); ResultSet rs = stmt.executeQuery(query); } catch (SQLException e) { // Forward to handler } // ... } public static void main(String[] args) throws InterruptedException { DBConnector connector = new DBConnector(/* suitable query */); Thread thread = new Thread(connector); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
Unfortunately database connections, like sockets, are not inherently interruptible. So this design does not permit a client to cancel a task by closing it if the corresponding thread is blocked on a long running activity such as a join query. Furthermore, it is important to provide a mechanism to close connections to prevent thread starvation caused because of the limited number of database connections available in the pool. Similar task cancellation mechanisms are required when using objects local to a method, such as sockets.
Compliant Solution (ThreadLocal)
This compliant solution uses a ThreadLocal
wrapper around the connection so that a thread that calls initialValue()
obtains a unique connection instance. The advantage of this approach is that a shutdownConnection()
method can be provided so that clients external to the class can also close the connection when the corresponding thread is blocked, or is performing some time consuming activity.
class DBConnector implements Runnable { final String query; DBConnector(String query) { this.query = query; } private static ThreadLocal<Connection> connectionHolder = new ThreadLocal<Connection>() { Connection connection = null; @Override public Connection initialValue() { try { // Username and password are hard coded for brevity connection = DriverManager.getConnection ("jdbc:driver:name", "username","password"); } catch (SQLException e) { // Forward to handler } return connection; } @Override public void set(Connection con) { if(connection == null) { // Shuts down connection when null value is passed try { connection.close(); } catch (SQLException e) { // Forward to handler } } else { connection = con; } } }; public static Connection getConnection() { return connectionHolder.get(); } public static void shutdownConnection() { // Allows client to close connection anytime connectionHolder.set(null); } public void run() { Connection dbConnection = getConnection(); Statement stmt; try { stmt = dbConnection.createStatement(); ResultSet rs = stmt.executeQuery(query); } catch (SQLException e) { // Forward to handler } // ... } public static void main(String[] args) throws InterruptedException { DBConnector connector = new DBConnector(/* suitable query */); Thread thread = new Thread(connector); thread.start(); Thread.sleep(5000); connector.shutdown(); } }
Noncompliant Code Example (Interrupting thread executing in thread pool)
This noncompliant code example uses a runnable task shown below:
public class Task implements Runnable { @Override public void run() { try { Thread.sleep(10000); // Do some time consuming task } catch(InterruptedException ie) { // Forward to handler } } }
The doSomething()
method of class PoolService
submits the task to a thread pool. It carries out the task and if an exception results during the execution of the code in the try
block, it interrupts the thread in an attempt to cancel it.
class PoolService { private final ExecutorService pool; public PoolService(int poolSize) { pool = Executors.newFixedThreadPool(poolSize); } public void doSomething() throws InterruptedException { Thread thread = new Thread(new Task()); try { pool.submit(thread); // ... } catch(Throwable t) { thread.interrupt(); // Forward to handler } } }
However, the interruption has no effect on the execution of the task because it is executing in a thread pool. Furthermore, note that it is unknown which tasks would be running in the thread pool at the time the interruption notification is delivered [[Goetz 06]].
Compliant Solution (Cancel using the Future)
This compliant solution obtains the return value of the task (future) and invokes the cancel()
method on it.
class PoolService { private final ExecutorService pool; public PoolService(int poolSize) { pool = Executors.newFixedThreadPool(poolSize); } public void doSomething() throws InterruptedException { Future<?> future = null; try { future = pool.submit(new Task()); // ... } catch(Throwable t) { future.cancel(true); // Forward to handler } } }
Risk Assessment
Trying to force thread shutdown can result in inconsistent object state and corrupt the object. Critical resources may also leak if cleanup operations are not carried out as required.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON13- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Thread, method stop
[[Darwin 04]] 24.3 Stopping a Thread
[[JDK7 08]] Concurrency Utilities, More information: Java Thread Primitive Deprecation
[[JPL 06]] 14.12.1. Don't stop and 23.3.3. Shutdown Strategies
[[JavaThreads 04]] 2.4 Two Approaches to Stopping a Thread
[[Goetz 06]] Chapter 7: Cancellation and shutdown
CON12-J. Avoid deadlock by requesting and releasing locks in the same order 11. Concurrency (CON) VOID CON14-J. Ensure atomicity of 64-bit operations