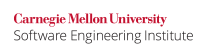
Security-intensive applications must avoid use of insecure or weak cryptographic primitives. The computational capacity of modern computers permits circumvention of such cryptography via brute-force attacks. For example, the Data Encryption Standard (DES) encryption algorithm is considered highly insecure; messages encrypted using DES have been decrypted by brute force within a single day by machines such as the Electronic Frontier Foundation's (EFF) Deep Crack.
Noncompliant Code Example
This noncompliant code example encrypts a String
input using a weak cryptographic algorithm (DES):
SecretKey key = KeyGenerator.getInstance("DES").generateKey(); Cipher cipher = Cipher.getInstance("DES"); cipher.init(Cipher.ENCRYPT_MODE, key); // Encode bytes as UTF8; strToBeEncrypted contains // the input string that is to be encrypted byte[] encoded = strToBeEncrypted.getBytes("UTF8"); // Perform encryption byte[] encrypted = cipher.doFinal(encoded);
Compliant Solution
This compliant solution uses the more secure Advanced Encryption Standard (AES) algorithm to perform the encryption.
Cipher cipher = Cipher.getInstance("AES"); KeyGenerator kgen = KeyGenerator.getInstance("AES"); kgen.init(128); // 192 and 256 bits may be unavailable SecretKey skey = kgen.generateKey(); byte[] raw = skey.getEncoded(); SecretKeySpec skeySpec = new SecretKeySpec(raw, "AES"); cipher.init(Cipher.ENCRYPT_MODE, skeySpec); // Encode bytes as UTF8; strToBeEncrypted contains // the input string that is to be encrypted byte[] encoded = strToBeEncrypted.getBytes("UTF8"); // Perform encryption byte[] encrypted = cipher.doFinal(encoded);
Applicability
Use of mathematically and computationally insecure cryptographic algorithms can result in the disclosure of sensitive information.
Weak cryptographic algorithms can be disabled in Java SE 7; see the Java PKI Programmer's Guide, Appendix D: Disabling Cryptographic Algorithms [Oracle 2011a].
Weak cryptographic algorithms may be used in scenarios that specifically call for a breakable cipher. For example, the ROT13 cipher is commonly used on bulletin boards and websites when the purpose of encryption is to protect people from the information rather than protect information from the people.
Bibliography
[Oracle 2011a] | Appendix D: Disabling Cryptographic Algorithms |
[Oracle 2013b] | Java Cryptography Architecture (JCA) Reference Guide |