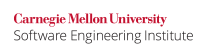
Validate method parameters to ensure that they fall within the bounds of the method's intended design. This practice ensures that operations on the method's parameters yield valid results. Failure to validate method parameters can result in incorrect calculations, runtime exceptions, violation of class invariants and inconsistent object state.
Programmers often assume that validation of arguments passed to private
methods is unnecessary because such methods cannot be accessed directly by code present outside their enclosing class. This assumption is incorrect. Programming errors often arise when legitimate code behaves in unanticipated ways. For example, a tainted value may propagate from a public
API to a private methods via its parameters.
Never use assertions should to validate parameters of public
methods. According to the Java Language Specification [[JLS 2005]], Section 14.10 "The assert
Statement"
Along similar lines, assertions should not be used for argument-checking in
public
methods. Argument-checking is typically part of the contract of a method, and this contract must be upheld whether assertions are enabled or disabled.Another problem with using assertions for argument checking is that erroneous arguments should result in an appropriate runtime exception (such as
IllegalArgumentException
,IndexOutOfBoundsException
orNullPointerException
). An assertion failure will not throw an appropriate exception. Again, it is not illegal to use assertions for argument checking onpublic
methods, but it is generally inappropriate.
When defensive copying is necessary, make the defensive copies before parameter validation; validate the copies rather than the original parameters. See guideline SER07-J. Make defensive copies of private mutable components for additional information.
Noncompliant Code Example
The method AbsAdd()
computes and returns the sum of the absolute value of parameters x
and y
. It lacks parameter validation. Consequently, it can produce incorrect results either due to integer overflow or when either or both of its arguments are Math.abs(Integer.MIN_VALUE)
.
public static int AbsAdd(int x, int y) { return Math.abs(x) + Math.abs(y); } AbsAdd(Integer.MIN_VALUE, 1);
Noncompliant Code Example
This noncompliant code example uses assertions to validate arguments of a public
method.
public static int AbsAdd(int x, int y) { assert x != Integer.MIN_VALUE; assert y != Integer.MIN_VALUE; assert ((x <= Integer.MAX_VALUE - y)); assert ((x >= Integer.MIN_VALUE - y)); return Math.abs(x) + Math.abs(y); }
The conditions checked by the assertions are reasonable. However, the validation code is omitted when executing with assertions turned off.
Compliant Solution
This compliant solution validates the method arguments by ensuring that values passed to Math.abs()
exclude Integer.MIN_VALUE
and also by checking for integer overflow. Alternatively, the addition could be performed using type long
and the result of the addition stored in a local variable of type long
. This alternate implementation would require a check to ensure that the resulting long
can be represented in the range of the type int
. Failure of this latter check would indicate that an int
version of the addition would have overflowed.
public static int AbsAdd(int x, int y) { if((x == Integer.MIN_VALUE || y == Integer.MIN_VALUE) || (x>0 && y>0 && (x > Integer.MAX_VALUE - y)) || (x<0 && y<0 && (x < Integer.MIN_VALUE - y))) throw new IllegalArgumentException(); return Math.abs(x) + Math.abs(y); }
Exceptions
MET02-EX1: Parameter validation inside a method may be omitted when the stated contract of a method requires that the caller must validate arguments passed to the method. In this case, the validation must be performed by the caller for all invocations of the method.
MET02-EX2: Parameter validation may be omitted for parameters whose type adequately constrains the state of the parameter. This constraint should be clearly documented in the code.
MET02-EX3: Complete validation of all parameters of all methods may introduce added cost and complexity that exceeds its value for all but the most critical code. See, for example, NUM00-J. Detect or prevent integer overflow exception NUM00-EX2. In such cases, consider parameter validation at API boundaries, especially those that may involve interaction with untrusted code.
Risk Assessment
Failure to validate method parameters can result in inconsistent computations, runtime exceptions, and control flow vulnerabilities.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET02-J |
medium |
probable |
medium |
P8 |
L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Bloch 2008]] Item 38: Check parameters for validity
[[Daconta 2003]] Item 7: My Assertions Are Not Gratuitous
[[ESA 2005]] Rule 68: Explicitly check method parameters for validity, and throw an adequate exception in case they are not valid. Do not use the assert statement for this purpose
[[JLS 2005]] 14.10 The assert Statement
MET00-J. Avoid ambiguous uses of overloading 05. Methods (MET)