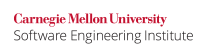
The modulus operator in Java is defined in the Java Language Specification, Second Edition, Section 15.17.3, paragraph 3:
The remainder operation for operands that are integers after binary numeric promotion (§5.6.2) produces a result value such that (a/b)*b+(a%b) is equal to a. This identity holds even in the special case that the dividend is the negative integer of largest possible magnitude for its type and the divisor is -1 (the remainder is 0). It follows from this rule that the result of the remainder operation can be negative only if the dividend is negative, and can be positive only if the dividend is positive; moreover, the magnitude of the result is always less than the magnitude of the divisor.
Although clearly defined in the Java specification, the behavior is undefined in several early C implementations and a more general algebraic solution assumes a positive result. Therefore, it is possible to have unintended behavior from use of this operator.
The result of the modulus operator implies the following behavior:
5 % 3 produces 2 5 % (-3) produces 2 (-5) % 3 produces -2 (-5) % (-3) produces -2
The result has the same sign as the dividend (the first operand in the expression).
Noncompliant Code Example
In this noncompliant example, the integer hash references an element of the hash table. However, since hash is not guaranteed to be positive, the lookup function may fail, producing a java.lang.ArrayIndexOutOfBoundsException on all negative inputs.
private int size=16; public int[] hashTable=new int[size]; public int lookup(int hash) { return hashTable[hash % size]; }
Compliant Solution
A compliant solution can call a function that returns a true (always positive) modulus.
/* modulo function giving non-negative result */ private int imod(int i, int j) { return (i < 0) ? ((-i) % j) : (i % j); } private int size=16; public int[] hashTable=new int[size]; public int lookup(int hash) { return hashTable[imod(hash, size)]; }
Or, an explicit range check must be preformed on the numerator.
private int size=16; public int[] hashTable=new int[size]; public int lookup(int hash) { if(hash < 0) return hashTable[(-hash) % size]; return hashTable[hash % size]; }
Risk Assessment
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
|
low |
unlikely |
high |
P1 |
L3 |
Automated Detection
Unknown
Other Languages
This rule appears in the C Secure Coding Standard as INT10-C. Do not assume a positive remainder when using the % operator, and INT10-CPP. Do not assume a positive remainder when using the % operator,
References
JLS 15.17.3