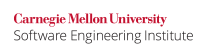
The Java Language Specification defines the Java Memory Model (JMM) which describes possible behaviors of a multi-threaded Java program. Memory that can be shared between threads is called shared memory or heap memory. The term variable is used in the context of this guideline, to refer to both fields and array elements [[JLS 05]].
All instance fields, static
fields, and array elements are stored in heap memory. Local variables, formal method parameters, or exception handler parameters are never shared between threads and are not affected by the memory model.
Concurrent executions are typically interleaved but the situation is complicated by statements that may be reordered by the compiler or runtime system. This results in execution orders that are not immediately obvious from an examination of the source-code.
There are two requirements for implementing synchronization correctly:
1. The happens-before consistency: If two accesses follow the happens-before relationship, data races cannot occur. However, this is necessary but not sufficient for acceptable program behavior. In addition, the particular execution order of a program must be sequential consistent.
Consider the following example in which a
and b
are (shared) global variables or instance fields but r1
and r2
are local variables not accessible by other threads.
Initially, let a = 0
and b = 0
.
|
|
---|---|
|
|
|
|
Because, in Thread 1
, the two assignments a = 10;
and r1 = b;
are not related, the compiler or runtime system is free to reorder them. Similarly in Thread 2
, the statements may be freely reordered. Although it may seem counter-intuitive, the Java memory model allows a read to see a write that occurs later in the execution order.
Two possible execution orders and actual assignments are:
Execution Order |
Assignment |
Assigned Value |
Notes |
---|---|---|---|
1. |
|
10 |
|
2. |
|
20 |
|
3. |
|
0 |
Reads initial value of |
4. |
|
0 |
Reads initial value of |
In this ordering, r1
and r2
read the original values of the variables a
and b
even though they might be expected to see the updated values.
Execution Order |
Statement |
Assigned Value |
Notes |
---|---|---|---|
1. |
|
20 |
Reads later value (in step 4.) of write, that is 20 |
2. |
|
10 |
Reads later value (in step 3.) of write, that is 10 |
3. |
|
10 |
|
4. |
|
20 |
|
In this ordering, r1
and r2
read the values of a
and b
written from step 3 and 4, before the steps are executed. Such counter-intuitive behavior necessitates the sequential consistency property.
2. [Sequential consistency]: "The fact that we allow a read to see a write that comes later in the execution order can sometimes thus result in unacceptable behaviors." [[JLS 05]]. In such cases, sequential consistency is required.
Declaring a variable volatile
guarantees the happens-before relationship so that writes are always visible to subsequent reads from any thread. It also ensures sequential consistency, in that, volatile reads and writes cannot be reordered and as required by the modern JMM, volatile read and write operations are also not reordered with respect to operations on non-volatile variables.
Noncompliant Code Example
This noncompliant code example uses a shutdown()
method to set a non-volatile done
flag that is checked in the run()
method. If some thread invokes the shutdown()
method to set the flag, it is possible that another thread might not observe this change. Consequently, it may be forced to sleep even though the condition variable disallows this.
final class ControlledStop implements Runnable { private boolean done = false; public void run() { while(!done) { try { // ... Thread.currentThread().sleep(1000); // Do something } catch(InterruptedException ie) { // handle } } } protected void shutdown(){ done = true; } }
Compliant Solution
This compliant solution declares the flag volatile
so that updates by one thread are immediately visible to another thread.
final class ControlledStop implements Runnable { private volatile boolean done = false; // ... }
Noncompliant Code Example
This noncompliant code example declares a non-volatile int
variable that is initialized in the constructor depending on a security check. In a multi-threading scenario, it is possible that the statements will be reordered so that the boolean
flag initialized
is set to true
before the initialization has concluded. If it is possible to obtain a partially initialized instance of the class in a subclass using a finalizer attack (OBJ04-J. Do not allow partially initialized objects to be accessed), a race condition can be exploited by invoking the getBalance()
method to obtain the balance even though initialization is still underway.
class BankOperation { private int balance = 0; private boolean initialized = false; public BankOperation() { if (!performAccountVerification()) { throw new SecurityException("Invalid Account"); } balance = 1000; initialized = true; } private int getBalance() { if(initialized == true) return balance; else return -1; } }
Compliant Solution
This compliant solution declares the initialized
flag as volatile
to ensure that the initialization statements are not reordered.
class BankOperation { private int balance = 0; private volatile boolean initialized = false; // Declared volatile // ... }
The use of the volatile
keyword is inappropriate for composite operations on shared variables (CON01-J. Ensure visibility of shared variables and atomicity of composite operations).
Risk Assessment
Failing to use volatile to guarantee visibility of shared values across multiple thread and prevent reordering of statements can result in unpredictable control flow.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON00- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object