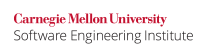
Threads always preserve class invariants when they are allowed to exit normally. Programmers often try to terminate threads abruptly when they believe that the task is accomplished, the request has been canceled, or the program or JVM needs to quickly shut down.
A few thread APIs were introduced to facilitate thread suspension, resumption, and termination but were later deprecated because of inherent design weaknesses. For example, the Thread.stop()
method causes the thread to immediately throw a ThreadDeath
exception, which usually stops the thread.
Invoking Thread.stop()
results in the release of all the locks a thread has acquired, which may corrupt the state of the object. The thread could catch the ThreadDeath
exception and use a finally
block in an attempt to repair the inconsistent object. However, this requires careful inspection of all the synchronized methods and blocks because a ThreadDeath
exception can be thrown at any point during the thread's execution. Furthermore, code must be protected from ThreadDeath
exceptions that may result when executing catch
or finally
blocks [[Sun 1999]].
More information about deprecated methods is available in guideline MET15-J. Do not use deprecated or obsolete methods. Also, refer to guideline EXC09-J. Prevent inadvertent calls to System.exit() or forced shutdown for information on preventing data corruption when the JVM is shut down abruptly.
Noncompliant Code Example (Deprecated Thread.stop()
)
This noncompliant code example shows a thread that fills a vector with pseudo-random numbers. The thread is forcefully stopped after a given amount of time.
public final class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); public Vector<Integer> getVector() { return vector; } @Override public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Thread thread = new Thread(new Container()); thread.start(); Thread.sleep(5000); thread.stop(); } }
Because the Vector
class is thread-safe, operations performed by multiple threads on its shared instance are expected to leave it in a consistent state. For instance, the Vector.size()
method always returns the correct number of elements in the vector even in the face of concurrent changes to the vector. This is because the vector instance uses its own intrinsic lock to prevent other threads from accessing it while its state is temporarily inconsistent.
However, the Thread.stop()
method causes the thread to stop what it is doing and throw a ThreadDeath
exception. All acquired locks are subsequently released [[API 2006]]. If the thread is in the process of adding a new integer to the vector when it is stopped, the vector may become accessible while it is in an inconsistent state. This can result in Vector.size()
returning an incorrect element count, for example, because the element count is incremented after adding the element.
Compliant Solution (volatile
flag)
This compliant solution uses a volatile
flag to terminate the thread. The shutdown()
accessor method is used to set the flag to true
. The thread's run()
method polls the done
flag and terminates when it becomes true.
public final class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); private volatile boolean done = false; public Vector<Integer> getVector() { return vector; } public void shutdown() { done = true; } @Override public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (!done && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container container = new Container(); Thread thread = new Thread(container); thread.start(); Thread.sleep(5000); container.shutdown(); } }
Compliant Solution (Interruptible)
In this compliant solution, the Thread.interrupt()
method is called from main()
to terminate the thread. Invoking Thread.interrupt()
sets an internal interrupt status flag. The thread polls that flag using the Thread.interrupted()
method, which returns true if the current thread has been interrupted and clears the interrupt status.
public class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); public Vector<Integer> getVector() { return vector; } @Override public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (!Thread.interrupted() && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container c = new Container(); Thread thread = new Thread(c); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
A thread may use interruption for performing tasks other than cancellation and shutdown. Consequently, a thread should not be interrupted unless its interruption policy is known in advance. Failure to do so can result in failed interruption requests.
Compliant Solution (Runtime Permission stopThread
)
Removing the default permission java.lang.RuntimePermission
stopThread
permission from the security policy file prevents threads from being stopped using the Thread.stop()
method. This approach is not recommended for trusted, custom-developed code that uses that method because the existing design presumably depends upon the ability of the system to perform this action. Furthermore, the system may not be designed to properly handle the resulting exception. In these cases, it is preferable to implement an alternate design approach corresponding to another compliant solutions described in this guideline.
Risk Assessment
Forcing a thread to stop can result in inconsistent object state. Critical resources may also leak if clean-up operations are not carried out as required.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
THI05- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 2006]] Class Thread, method stop
, interface ExecutorService
[[Sun 1999]]
[[Darwin 2004]] 24.3 Stopping a Thread
[[JDK7 2008]] Concurrency Utilities, More information: Java Thread Primitive Deprecation
[[JPL 2006]] 14.12.1. Don't stop and 23.3.3. Shutdown Strategies
[[JavaThreads 2004]] 2.4 Two Approaches to Stopping a Thread
[[Goetz 2006]] Chapter 7: Cancellation and shutdown
THI04-J. Notify all waiting threads instead of a single thread 12. Locking (LCK) LCK09-J. Do not perform operations that may block while holding a lock