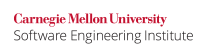
The wait()
method is employed to temporarily cede possession of a lock so that another thread can proceed. It must always be used inside a synchronized block. To resume activity, the other thread must notify the waiting thread. Moreover, the wait
method should be invoked in a loop that checks if a condition predicate holds.
A condition statement is used so that the correct thread is notified since invocation of notify
or notifyAll
in another thread cannot pin point which waiting thread needs to be resumed. Another use is that sometimes a thread needs to block until a condition becomes true, for instance, when it cannot proceed without obtaining some data from a stream.
synchronized (object) { while (<condition does not hold>) { object.wait(); } //proceed when condition holds }
Two properties come into picture here:
- Liveness: Every operation or method invocation executes to completion without interruptions, even if it goes against safety.
Safety: Its main goal is to ensure that all objects maintain consistent states in a multi-threaded environment. [[Lea 00]]
To guarantee liveness, the while loop condition should be tested before proceeding to invoke wait
. This is because the condition might be true which indicates that a notify has already been sent from the other thread. Invoking wait
after the notify has already been sent invites an infinite blocking state.
To guarantee safety, the while loop condition should be tested even after the call to wait
. While wait is meant to block indefinitely till a notification is received, this practice is touted because: [[Bloch 01]]
- Thread in the middle: A third thread can acquire the lock on the shared object during the interval between a notification being sent and the receiving thread actually resuming execution. This thread can change the state of the object leaving it inconsistent. This is akin to the "time of call, time of use" (TOCTOU) condition.
- Malicious notifications: There is no guarantee that a notification will not be sent when the condition does not hold. This means that the invocation of
wait
will be nullified by the notification. - Sometimes on receipt of a
notifyAll
signal, an unrelated thread can start executing and it is possible for its condition to be true. - Certain JVM implementations are vulnerable to spurious wakeups, that result in waiting threads waking up even without a notification.
Due to these reasons, checking the condition after wait
is called is indispensable.
Noncompliant Code Example
This noncompliant example invokes the wait
method inside a traditional if
block and fails to check the post condition after the (accidental or malicious) notification is received. This means that the thread can waken when it is not supposed to.
synchronized(object) { if(<condition does not hold>) object.wait(); //proceed when condition holds }
Compliant Solution
The compliant solution encloses the wait
method in a while
loop and as a result checks the condition during both pre and post wait
invocation times.
//condition predicate is guarded by a lock on the shared object/variable synchronized (object) { while (<condition does not hold>) { object.wait(); } //proceed when condition holds }
Risk Assessment
To guarantee liveness and safety, the wait()
method should always be called inside a while loop.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON31-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Object
[[Bloch 01]] Item 50: Never invoke wait outside a loop
[[Lea 00]] 3.2.2 Monitor Mechanics, 1.3.2 Liveness
[[Goetz 06]] Section 14.2, Using Condition Queues
CON30-J. Synchronize access to shared mutable variables 08. Concurrency (CON) CON32-J. Prefer notifyAll() to notify()