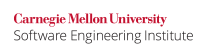
The serialization and deserialization features can be exploited to bypass security manager checks. A serializable class may employ security manager checks in its constructors for various reasons. For example, the checks prevent an attacker from modifying the internal state of the class.
Noncompliant Code Example
In this noncompliant example, security manager checks are used within the constructor but are not replicated throughout, specifically, within the readObject
and writeObject
methods that are used in the serialization-deserialization process. This allows an attacker to maliciously create instances of the class that bypass security manager checks when deserialization is performed.
import java.io.Serializable; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public final class CreditCard implements Serializable { //private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; public CreditCard() { //initialize credit_card to default value credit_card = DEFAULT; } //allows callers to modify (private) internal state public void changeCC(String newCC) { if (credit_card.equals(newCC)) { // no change return; } else { validateInput(newCC); credit_card = newCC; } } // readObject correctly enforces checks during deserialization private void readObject(ObjectInputStream in) { in.defaultReadObject(); // if the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { validateInput(credit_card); } } // allows callers to retrieve internal state public String getValue() { return somePublicValue; } // writeObject correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) { out.writeObject(credit_card); } }
Compliant Solution
The compliant solution correctly implements security manager checks in all constructors, methods that can modify internal state and methods that retrieve internal state. As a result, an attacker cannot create an instance of the object with modified state (using deserialization) and cannot simply read the serialized byte stream to uncover sensitive data (using serialization).
import java.io.Serializable; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public final class SecureCreditCard implements Serializable { //private internal state private String credit_card; private static final String DEFAULT = "DEFAULT"; public SecureCreditCard() { //initialize credit_card to default value credit_card = DEFAULT; } //allows callers to modify (private) internal state public void changeCC(String newCC) { if (credit_card.equals(newCC)) { // no change return; } else { // check permissions to modify credit_card performSecurityManagerCheck(); validateInput(newCC); credit_card = newCC; } } // readObject correctly enforces checks during deserialization private void readObject(ObjectInputStream in) { in.defaultReadObject(); // if the deserialized name does not match the default value normally // created at construction time, duplicate the checks if (!DEFAULT.equals(credit_card)) { performSecurityManagerCheck(); validateInput(credit_card); } } // allows callers to retrieve internal state public String getValue() { // check permission to get value performSecurityManagerCheck(); return somePublicValue; } // writeObject correctly enforces checks during serialization private void writeObject(ObjectOutputStream out) { // duplicate check from getValue() performSecurityManagerCheck(); out.writeObject(credit_card); } }
Risk Assessment
Allowing serialization or deserialization to bypass the Security Manager may result in sensitive data being compromised or modified.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO33-J |
high |
probable |
high |
P6 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 07]] Guideline 5-3 Duplicate the SecurityManager checks enforced in a class during serialization and deserialization
[[Long 05]] Section 2.4, Serialization
SER30-J. Do not serialize sensitive data 07. Input Output (FIO) FIO34-J. Ensure all resources are properly closed when they are no longer needed