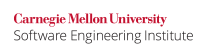
Threads always preserve class invariants when they are allowed to exit normally, as long as the specification is followed. Programmers often try to forcefully terminate threads when they believe that the task is accomplished, the request has been canceled or the program or JVM needs to quickly shut down.
A few thread APIs were introduced to facilitate thread suspension, resumption and termination but were later deprecated because of inherent design weaknesses. The Thread.stop()
method is one example. It throws a ThreadDeath
exception to stop the thread.
Invoking Thread.stop()
results in the release of all the locks a thread has acquired which may corrupt the state of the object. The thread could catch the ThreadDeath
exception and use a finally
block in an attempt to repair the inconsistent object, however, this requires careful inspection of all the synchronized methods and blocks because a ThreadDeath
exception can be thrown anywhere in the code. Furthermore, code must be protected from ThreadDeath
exceptions that may result when executing the catch
or finally
blocks [[Sun 99]] .
More information about deprecated methods is available in MET15-J. Do not use deprecated or obsolete methods. Also, refer to EXC09-J. Prevent inadvertent calls to System.exit() or forced shutdown for information on preventing data corruption when the JVM is abruptly shut down.
Noncompliant Code Example (Deprecated Thread.stop()
)
This noncompliant code example shows a thread that fills a vector with pseudorandom numbers. The thread is forcefully stopped after a given amount of time.
public final class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); public Vector<Integer> getVector() { return vector; } public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Thread thread = new Thread(new Container()); thread.start(); Thread.sleep(5000); thread.stop(); } }
Because the class Vector
is thread-safe, operations performed by multiple threads on its shared instance are expected to leave it in a consistent state. For instance, the Vector.size()
method always returns the correct number of elements in the vector even when an element is added or removed. This is because the vector instance uses its own intrinsic lock to prevent other threads from accessing it while its state is temporarily inconsistent.
However, the Thread.stop()
method causes the thread to stop what it is doing and throw a ThreadDeath
exception. All acquired locks are subsequently released [[API 06]]. If the thread is in the process of adding a new integer to the vector when it is stopped, the vector may become accessible while it is in an inconsistent state. For example, Vector.size()
may return two even though the vector contains three elements (as the element count is incremented after adding the element).
Compliant Solution (volatile
flag)
This compliant solution uses a volatile
flag to stop the thread. An accessor method shutdown()
is used to set the flag to true
. The thread's run()
method polls the done
flag, and terminates when it becomes true
.
public final class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); private volatile boolean done = false; public Vector<Integer> getVector() { return vector; } public void shutdown() { done = true; } public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (!done && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container container = new Container(); Thread thread = new Thread(container); thread.start(); Thread.sleep(5000); container.shutdown(); } }
Compliant Solution (Interruptible)
This compliant solution stops the thread using the Thread.interrupt()
method.
public class Container implements Runnable { private final Vector<Integer> vector = new Vector<Integer>(1000); public Vector<Integer> getVector() { return vector; } public synchronized void run() { Random number = new Random(123L); int i = vector.capacity(); while (!Thread.interrupted() && i > 0) { vector.add(number.nextInt(100)); i--; } } public static void main(String[] args) throws InterruptedException { Container c = new Container(); Thread thread = new Thread(c); thread.start(); Thread.sleep(5000); thread.interrupt(); } }
This method interrupts the current thread, however, it only stops the thread because the code polls the interrupted flag using the method Thread.interrupted()
.
Upon receiving the interruption, the interrupted status of the thread is cleared and an InterruptedException
is thrown. No guarantees are provided by the JVM on when the interruption will be detected by blocking methods such as Thread.sleep()
and Object.wait()
. A thread may use interruption for performing tasks other than cancellation and shutdown. Consequently, a thread should not be interrupted unless its interruption policy is known in advance. Failure to follow this advice can result in the corruption of mutable shared state.
Compliant Solution (RuntimePermission stopThread
)
Remove the default permission java.lang.RuntimePermission
stopThread
from the security policy file to deny the Thread.stop()
invoking code, the required privileges.
Risk Assessment
Forcing a thread to stop can result in inconsistent object state. Critical resources may also leak if clean-up operations are not carried out as required.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON13- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Thread, method stop
, interface ExecutorService
[[Sun 99]]
[[Darwin 04]] 24.3 Stopping a Thread
[[JDK7 08]] Concurrency Utilities, More information: Java Thread Primitive Deprecation
[[JPL 06]] 14.12.1. Don't stop and 23.3.3. Shutdown Strategies
[[JavaThreads 04]] 2.4 Two Approaches to Stopping a Thread
[[Goetz 06]] Chapter 7: Cancellation and shutdown
CON12-J. Avoid deadlock by requesting and releasing locks in the same order 11. Concurrency (CON) CON15-J. Ensure actively held locks are released on exceptional conditions